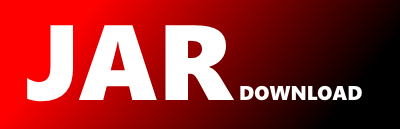
com.globalmentor.w3c.spec.HTML Maven / Gradle / Ivy
/*
* Copyright © 1996-2012 GlobalMentor, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.globalmentor.w3c.spec;
import java.net.URI;
import java.util.*;
import com.globalmentor.java.Characters;
import com.globalmentor.model.IDed;
import com.globalmentor.net.ContentType;
import static com.globalmentor.collections.Sets.*;
import static com.globalmentor.java.Characters.*;
import static com.globalmentor.java.Objects.*;
import static com.globalmentor.net.ContentTypeConstants.*;
/**
* Definitional constants of the HyperText Markup Language (HTML).
* @author Garret Wilson
* @see HTML, The Web’s Core Language
* @see W3C QA - Recommended list of DTDs
*/
public class HTML {
/** HTML MIME subtype. */
public static final String HTML_SUBTYPE = "html";
/** An XHTML application. */
public static final String XHTML_XML_SUBTYPE = "xhtml" + ContentType.SUBTYPE_SUFFIX_DELIMITER_CHAR + XML.XML_SUBTYPE_SUFFIX;
/** An XHTML fragment (not yet formally defined). */
public static final String XHTML_XML_EXTERNAL_PARSED_ENTITY_SUBTYPE = "xhtml" + ContentType.SUBTYPE_SUFFIX_DELIMITER_CHAR
+ XML.XML_EXTERNAL_PARSED_ENTITY_SUBTYPE_SUFFIX;
/** The content type for HTML: text/html
. */
public static final ContentType HTML_CONTENT_TYPE = ContentType.create(ContentType.TEXT_PRIMARY_TYPE, HTML_SUBTYPE);
/** The content type for XHTML: application/xhtml+xml
. */
public static final ContentType XHTML_CONTENT_TYPE = ContentType.create(ContentType.APPLICATION_PRIMARY_TYPE, XHTML_XML_SUBTYPE);
/** The content type for an XHTML fragment: application/xhtml+xml-external-parsed-entity
. */
public static final ContentType XHTML_FRAGMENT_CONTENT_TYPE = ContentType.create(ContentType.APPLICATION_PRIMARY_TYPE,
XHTML_XML_EXTERNAL_PARSED_ENTITY_SUBTYPE);
/** The old extension for HTML resource names. */
public static final String HTM_NAME_EXTENSION = "htm";
/** The extension for HTML resource names. */
public static final String HTML_NAME_EXTENSION = "html";
/** The extension for XHTML resource names. */
public static final String XHTML_NAME_EXTENSION = "xhtml";
/** The recommended prefix to the XHTML namespace. */
public static final String XHTML_NAMESPACE_PREFIX = "xhtml";
/** The URI to the XHTML namespace. */
public static final URI XHTML_NAMESPACE_URI = URI.create("http://www.w3.org/1999/xhtml");
/** The public ID for the HTML 2.0 DTD. */
public static final String HTML_2_0_PUBLIC_ID = "-//IETF//DTD HTML 2.0//EN";
/** The public ID for the HTML 3.2 DTD. */
public static final String HTML_3_2_PUBLIC_ID = "-//W3C//DTD HTML 3.2 Final//EN";
/** The public ID for the HTML 4.01 Strict DTD. */
public static final String HTML_4_01_STRICT_PUBLIC_ID = "-//W3C//DTD HTML 4.01//EN";
/** The system ID for the HTML 4.01 Strict DTD. */
public static final String HTML_4_01_STRICT_SYSTEM_ID = "http://www.w3.org/TR/html4/strict.dtd";
/** The public ID for the HTML 4.01 Traditional DTD. */
public static final String HTML_4_01_TRANSITIONAL_PUBLIC_ID = "-//W3C//DTD HTML 4.01 Transitional//EN";
/** The system ID for the HTML 4.01 Traditional DTD. */
public static final String HTML_4_01_TRANSITIONAL_SYSTEM_ID = "http://www.w3.org/TR/html4/loose.dtd";
/** The public ID for the HTML 4.01 Frameset DTD. */
public static final String HTML_4_01_FRAMESET_PUBLIC_ID = "-//W3C//DTD HTML 4.01 Frameset//EN";
/** The system ID for the HTML 4.01 Frameset DTD. */
public static final String HTML_4_01_FRAMESET_SYSTEM_ID = "http://www.w3.org/TR/html4/frameset.dtd";
/** The public ID for the XHTML 1.0 Strict DTD. */
public static final String XHTML_1_0_STRICT_PUBLIC_ID = "-//W3C//DTD XHTML 1.0 Strict//EN";
/** The system ID for the XHTML 1.0 Strict DTD. */
public static final String XHTML_1_0_STRICT_SYSTEM_ID = "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd";
/** The public ID for the XHTML 1.0 Traditional DTD. */
public static final String XHTML_1_0_TRANSITIONAL_PUBLIC_ID = "-//W3C//DTD XHTML 1.0 Transitional//EN";
/** The system ID for the XHTML 1.0 Traditional DTD. */
public static final String XHTML_1_0_TRANSITIONAL_SYSTEM_ID = "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd";
/** The public ID for the XHTML 1.0 Frameset DTD. */
public static final String XHTML_1_0_FRAMESET_PUBLIC_ID = "-//W3C//DTD XHTML 1.0 Frameset//EN";
/** The system ID for the XHTML 1.0 Frameset DTD. */
public static final String XHTML_1_0_FRAMESET_SYSTEM_ID = "http://www.w3.org/TR/xhtml1/DTD/xhtml1-frameset.dtd";
/** The public ID for the XHTML 1.1 DTD. */
public static final String XHTML_1_1_PUBLIC_ID = "-//W3C//DTD XHTML 1.1//EN";
/** The system ID for the XHTML 1.1 DTD. */
public static final String XHTML_1_1_SYSTEM_ID = "http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd";
/** The public ID for the XHTML+MathML+SVG DTD. */
public static final String XHTML_MATHML_SVG_PUBLIC_ID = "-//W3C//DTD XHTML 1.1 plus MathML 2.0 plus SVG 1.1//EN";
/** The system ID for the XHTML+MathML+SVG DTD. */
public static final String XHTML_MATHML_SVG_SYSTEM_ID = "http://www.w3.org/2002/04/xhtml-math-svg/xhtml-math-svg.dtd";
/**
* Characters considered space characters in HTML.
* @see HTML5 space characters
*/
public static final Characters SPACE_CHARACTERS = new Characters(SPACE_CHAR, CHARACTER_TABULATION_CHAR, LINE_FEED_CHAR, FORM_FEED_CHAR, CARRIAGE_RETURN_CHAR);
//The XHTML 1.0 document element names.
//TODO fix; some are missing since these were copied from the OEB 1.0
public static final String ELEMENT_A = "a";
public static final String ELEMENT_ADDRESS = "address";
public static final String ELEMENT_APPLET = "applet";
public static final String ELEMENT_AREA = "area";
public static final String ELEMENT_ARTICLE = "article";
public static final String ELEMENT_ASIDE = "aside";
public static final String ELEMENT_B = "b";
public static final String ELEMENT_BASE = "base";
public static final String ELEMENT_BIG = "big";
public static final String ELEMENT_BLOCKCODE = "blockcode";
public static final String ELEMENT_BLOCKQUOTE = "blockquote";
public static final String ELEMENT_BODY = "body";
public static final String ELEMENT_BR = "br";
public static final String ELEMENT_BUTTON = "button";
public static final String ELEMENT_CAPTION = "caption";
public static final String ELEMENT_CENTER = "center";
public static final String ELEMENT_CITE = "cite";
public static final String ELEMENT_COL = "col";
public static final String ELEMENT_CODE = "code";
public static final String ELEMENT_DD = "dd";
public static final String ELEMENT_DFN = "dfn";
public static final String ELEMENT_DIV = "div";
public static final String ELEMENT_DL = "dl";
public static final String ELEMENT_DT = "dt";
public static final String ELEMENT_EM = "em";
public static final String ELEMENT_EMBED = "embed";
public static final String ELEMENT_FIELDSET = "fieldset";
public static final String ELEMENT_FIGCAPTION = "figcaption";
public static final String ELEMENT_FIGURE = "figure";
public static final String ELEMENT_FONT = "font";
public static final String ELEMENT_FOOTER = "footer";
public static final String ELEMENT_FORM = "form";
public static final String ELEMENT_H1 = "h1";
public static final String ELEMENT_H2 = "h2";
public static final String ELEMENT_H3 = "h3";
public static final String ELEMENT_H4 = "h4";
public static final String ELEMENT_H5 = "h5";
public static final String ELEMENT_H6 = "h6";
public static final String ELEMENT_HEAD = "head";
public static final String ELEMENT_HEADER = "header";
public static final String ELEMENT_HR = "hr";
public static final String ELEMENT_HTML = "html";
public static final String ELEMENT_I = "i";
public static final String ELEMENT_IFRAME = "iframe";
public static final String ELEMENT_IMG = "img";
public static final String ELEMENT_INPUT = "input";
public static final String ELEMENT_KBD = "kbd";
public static final String ELEMENT_LI = "li";
public static final String ELEMENT_LABEL = "label";
public static final String ELEMENT_LEGEND = "legend";
public static final String ELEMENT_LINK = "link";
public static final String ELEMENT_MAP = "map";
public static final String ELEMENT_META = "meta";
public static final String ELEMENT_NAV = "nav";
public static final String ELEMENT_OBJECT = "object";
public static final String ELEMENT_OL = "ol";
public static final String ELEMENT_OPTION = "option";
public static final String ELEMENT_P = "p";
public static final String ELEMENT_PARAM = "param";
public static final String ELEMENT_PRE = "pre";
public static final String ELEMENT_Q = "q";
public static final String ELEMENT_S = "s";
public static final String ELEMENT_SAMP = "samp";
public static final String ELEMENT_SCRIPT = "script";
public static final String ELEMENT_SECTION = "section";
public static final String ELEMENT_SELECT = "select";
public static final String ELEMENT_SMALL = "small";
public static final String ELEMENT_SPAN = "span";
public static final String ELEMENT_STRIKE = "strike";
public static final String ELEMENT_STRONG = "strong";
public static final String ELEMENT_STYLE = "style";
public static final String ELEMENT_SUB = "sub";
public static final String ELEMENT_SUP = "sup";
public static final String ELEMENT_TABLE = "table";
public static final String ELEMENT_TD = "td";
public static final String ELEMENT_TEXTAREA = "textarea";
public static final String ELEMENT_TH = "th";
public static final String ELEMENT_TBODY = "tbody";
public static final String ELEMENT_THEAD = "thead";
public static final String ELEMENT_TFOOT = "tfoot";
public static final String ELEMENT_TITLE = "title";
public static final String ELEMENT_TR = "tr";
public static final String ELEMENT_TT = "tt";
public static final String ELEMENT_U = "u";
public static final String ELEMENT_UL = "ul";
public static final String ELEMENT_VAR = "var";
//attributes
/**
* The delimiter character for separating parts of an attribute string (e.g. for HTML5 data- attributes). This is distinct from the namespace prefix
* delimiter.
*/
public static final char ATTRIBUTE_DELIMITER_CHAR = '-';
/**
* The identifier, with no delimiter, indicating that an attribute is an HTML5 data attribute
* @see HTML 5 Data Attributes
*/
public static final String DATA_ATTRIBUTE_ID = "data";
/** The attribute for class. */
public static final String ATTRIBUTE_CLASS = "class";
/** The attribute for direction. */
public static final String ATTRIBUTE_DIR = "dir";
/** The attribute for left-to-right direction. */
public static final String DIR_LTR = "ltr";
/** The attribute for right-to-left direction. */
public static final String DIR_RTL = "rtl";
/** The attribute for ID. */
public static final String ATTRIBUTE_ID = "id";
/** The attribute for language. */
public static final String ATTRIBUTE_LANG = "lang";
/** The attribute for name. */
public static final String ATTRIBUTE_NAME = "name";
/** The attribute for style. */
public static final String ATTRIBUTE_STYLE = "style";
/** The attribute for title. */
public static final String ATTRIBUTE_TITLE = "title";
/** The attribute for value. */
public static final String ATTRIBUTE_VALUE = "value";
//event attributes
public static final String ATTRIBUTE_ONCLICK = "onclick";
public static final String ATTRIBUTE_ONLOAD = "onload";
public static final String LINK_ATTRIBUTE_REL = "rel"; //the link type attribute for , , and ; see http://www.w3.org/TR/html5/links.html#linkTypes
public static final String LINK_ATTRIBUTE_TYPE = "type"; //the link MIME type attribute for , , and ; see http://www.w3.org/TR/html5/links.html#attr-hyperlink-type
//link types for , , and ; see http://www.w3.org/TR/html5/links.html#linkTypes
public static final String LINK_REL_ALTERNATE = "alternate"; //, /
public static final String LINK_REL_AUTHOR = "author"; //, /
public static final String LINK_REL_BOOKMARK = "bookmark"; ///
public static final String LINK_REL_EXTERNAL = "external"; ///
public static final String LINK_REL_HELP = "help"; //, /
public static final String LINK_REL_ICON = "icon"; //
public static final String LINK_REL_LICENSE = "license"; //, /
public static final String LINK_REL_NEXT = "next"; //, /
public static final String LINK_REL_NOFOLLOW = "nofollow"; ///
public static final String LINK_REL_NOREFERRER = "noreferrer"; ///
public static final String LINK_REL_PINGBACK = "pingback"; //
public static final String LINK_REL_PREFETCH = "prefetch"; //, /
public static final String LINK_REL_PREV = "prev"; //, /
public static final String LINK_REL_SEARCH = "search"; //, /
public static final String LINK_REL_SIDEBAR = "sidebar"; //, /
public static final String LINK_REL_STYLESHEET = "stylesheet"; //
public static final String LINK_REL_TAG = "tag"; //, /
//attributes for
public static final String ELEMENT_A_ATTRIBUTE_HREF = "href";
public static final String ELEMENT_A_ATTRIBUTE_TARGET = "target";
public static final String ELEMENT_A_ATTRIBUTE_REL = LINK_ATTRIBUTE_REL;
/**
* Link types for {@code}, {@code}, and {@code}.
* @author Garret Wilson
* @see HTML5 Link Types
*/
public enum LinkType implements IDed {
ALTERNATE(LINK_REL_ALTERNATE), //, /
AUTHOR(LINK_REL_AUTHOR), //, /
BOOKMARK(LINK_REL_BOOKMARK), ///
EXTERNAL(LINK_REL_EXTERNAL), ///
HELP(LINK_REL_HELP), //, /
ICON(LINK_REL_ICON), //
LICENSE(LINK_REL_LICENSE), //, /
NEXT(LINK_REL_NEXT), //, /
NOFOLLOW(LINK_REL_NOFOLLOW), ///
NOREFERRER(LINK_REL_NOREFERRER), ///
PINGBACK(LINK_REL_PINGBACK), //
PREFETCH(LINK_REL_PREFETCH), //, /
PREV(LINK_REL_PREV), //, /
SEARCH(LINK_REL_SEARCH), //, /
SIDEBAR(LINK_REL_SIDEBAR), //, /
STYLESHEET(LINK_REL_STYLESHEET), //
TAG(LINK_REL_TAG); //, /
private final String id;
private LinkType(final String id) {
this.id = checkInstance(id);
}
@Override
public String getID() {
return id;
}
}
//attributes for