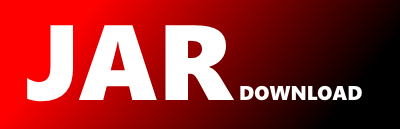
com.globalmentor.w3c.spec.XLink Maven / Gradle / Ivy
/*
* Copyright © 1996-2008 GlobalMentor, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.globalmentor.w3c.spec;
import java.net.URI;
import static com.globalmentor.w3c.spec.XML.*;
import org.w3c.dom.Element;
/**
* Definitions for XLink defined by the W3C at XML Linking Language (XLink).
* @author Garret Wilson
* @see XML Linking Language (XLink)
*/
public class XLink {
/** The default XLink namespace prefix. */
public static final String XLINK_NAMESPACE_PREFIX = "xlink";
/** The XLink namespace URI. */
public static final URI XLINK_NAMESPACE_URI = URI.create("http://www.w3.org/1999/xlink");
/** The type attribute (without the namespace). */
public static final String ATTRIBUTE_TYPE = "type";
/** The XLink simple link type. */
public static final String SIMPLE_TYPE = "simple";
/** The XLink extended link type. */
public static final String EXTENDED_TYPE = "extended";
/** The href attribute (without the namespace). */
public static final String ATTRIBUTE_HREF = "href";
/** The role attribute (without the namespace). */
public static final String ATTRIBUTE_ROLE = "role";
/** The title attribute (without the namespace). */
public static final String ATTRIBUTE_TITLE = "title";
/** The show attribute (without the namespace). */
public static final String ATTRIBUTE_SHOW = "show";
/** The actuate attribute (without the namespace). */
public static final String ATTRIBUTE_ACTUATE = "actuate";
/**
* Sets the xlink:type
attribute of the given element.
* @param element The element for which the XLink attribute should be set.
* @param type The xlink:type value to use, either XLink.SIMPLE_TYPE
or XLink.EXTENDED_TYPE
.
*/
public static void setXLinkType(final Element element, final String type) {
element.setAttributeNS(XLINK_NAMESPACE_URI.toString(), createQName(XLINK_NAMESPACE_PREFIX, ATTRIBUTE_TYPE), type); //set xlink:type=type
}
/**
* Sets the xlink:href
attribute of the given element.
* @param element The element for which the XLink attribute should be set.
* @param href The xlink:href value to use.
*/
public static void setXLinkHRef(final Element element, final String href) {
element.setAttributeNS(XLINK_NAMESPACE_URI.toString(), createQName(XLINK_NAMESPACE_PREFIX, ATTRIBUTE_HREF), href); //set xlink:href=href
}
/**
* Sets the xlink:type
and xlink:href
attributes of the given element.
* @param element The element for which the XLink attributes should be set.
* @param type The xlink:type value to use, either XLink.SIMPLE_TYPE
or XLink.EXTENDED_TYPE
.
* @param href The xlink:href value to use.
*/
public static void setXLink(final Element element, final String type, final String href) {
setXLinkType(element, type); //set the XLink type
setXLinkHRef(element, href); //set the XLink href
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy