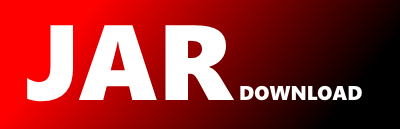
com.glookast.commons.capture.info.SoundFormat Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of commons-capture-info Show documentation
Show all versions of commons-capture-info Show documentation
Common classes used for interfacing with Glookast Products
package com.glookast.commons.capture.info;
import java.io.Serializable;
import java.util.Objects;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for SoundFormat complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="SoundFormat">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="channelCount" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="sampleSize" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="samplingRate" type="{http://www.w3.org/2001/XMLSchema}int"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SoundFormat", namespace = "http://info.capture.commons.glookast.com", propOrder = {
"channelCount",
"sampleSize",
"samplingRate"
})
public class SoundFormat implements Serializable
{
protected int channelCount;
protected int sampleSize;
protected int samplingRate;
/**
* Default no-arg constructor
*
*/
public SoundFormat() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public SoundFormat(final int channelCount, final int sampleSize, final int samplingRate) {
this.channelCount = channelCount;
this.sampleSize = sampleSize;
this.samplingRate = samplingRate;
}
/**
* Gets the value of the channelCount property.
*
*/
public int getChannelCount() {
return channelCount;
}
/**
* Sets the value of the channelCount property.
*
*/
public void setChannelCount(int value) {
this.channelCount = value;
}
/**
* Gets the value of the sampleSize property.
*
*/
public int getSampleSize() {
return sampleSize;
}
/**
* Sets the value of the sampleSize property.
*
*/
public void setSampleSize(int value) {
this.sampleSize = value;
}
/**
* Gets the value of the samplingRate property.
*
*/
public int getSamplingRate() {
return samplingRate;
}
/**
* Sets the value of the samplingRate property.
*
*/
public void setSamplingRate(int value) {
this.samplingRate = value;
}
@Override
public String toString()
{
return "SoundFormat{" +
"channelCount=" + channelCount +
", sampleSize=" + sampleSize +
", samplingRate=" + samplingRate +
'}';
}
@Override
public boolean equals(Object o)
{
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SoundFormat that = (SoundFormat) o;
return channelCount == that.channelCount &&
sampleSize == that.sampleSize &&
samplingRate == that.samplingRate;
}
@Override
public int hashCode()
{
return Objects.hash(channelCount, sampleSize, samplingRate);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy