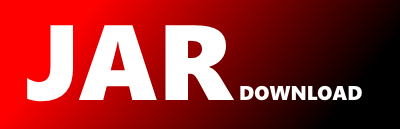
com.gluonhq.plugin.netbeans.template.visuals.GluonUserOptInVisual Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com-gluonhq-plugin-netbeans
Show all versions of com-gluonhq-plugin-netbeans
Gluon Plugin allows developers to create Java applications and their native images targeting Android, iOS, Linux, MacOS and Windows platforms using the same codebase.
/*
* Copyright (c) 2018, Gluon Software
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* * Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* * Neither the name of the copyright holder nor the names of its
* contributors may be used to endorse or promote products derived from
* this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.gluonhq.plugin.netbeans.template.visuals;
import com.gluonhq.plugin.templates.ProjectConstants;
import com.gluonhq.plugin.templates.TemplateUtils;
import org.openide.WizardDescriptor;
public class GluonUserOptInVisual extends GluonBasePanelVisual {
public GluonUserOptInVisual(GluonUserOptInPanel panel) {
initComponents();
this.panel = panel;
// Register listener on the textFields to make the automatic updates
emailTextField.getDocument().addDocumentListener(this);
uptodateCheckbox.addChangeListener(this);
mobileLicenseTextField.getDocument().addDocumentListener(this);
desktopLicenseTextField.getDocument().addDocumentListener(this);
}
/**
* This method is called from within the constructor to initialize the form. WARNING: Do NOT modify this code. The content of this method is always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
emailLabel = new javax.swing.JLabel();
emailTextField = new javax.swing.JTextField();
uptodateCheckbox = new javax.swing.JCheckBox();
jLabel1 = new javax.swing.JLabel();
jLabel2 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
jLabel4 = new javax.swing.JLabel();
jLabel5 = new javax.swing.JLabel();
mobileLicenseTextField = new javax.swing.JTextField();
desktopLicenseTextField = new javax.swing.JTextField();
emailLabel.setLabelFor(emailTextField);
org.openide.awt.Mnemonics.setLocalizedText(emailLabel, org.openide.util.NbBundle.getMessage(GluonUserOptInVisual.class, "GluonUserOptInVisual.emailLabel.text")); // NOI18N
emailTextField.setText(org.openide.util.NbBundle.getMessage(GluonUserOptInVisual.class, "GluonUserOptInVisual.emailTextField.text")); // NOI18N
uptodateCheckbox.setSelected(true);
org.openide.awt.Mnemonics.setLocalizedText(uptodateCheckbox, org.openide.util.NbBundle.getMessage(GluonUserOptInVisual.class, "GluonUserOptInVisual.uptodateCheckbox.text")); // NOI18N
org.openide.awt.Mnemonics.setLocalizedText(jLabel1, org.openide.util.NbBundle.getMessage(GluonUserOptInVisual.class, "GluonUserOptInVisual.jLabel1.text")); // NOI18N
jLabel1.setVerticalAlignment(javax.swing.SwingConstants.TOP);
org.openide.awt.Mnemonics.setLocalizedText(jLabel2, org.openide.util.NbBundle.getMessage(GluonUserOptInVisual.class, "GluonUserOptInVisual.jLabel2.text")); // NOI18N
org.openide.awt.Mnemonics.setLocalizedText(jLabel3, org.openide.util.NbBundle.getMessage(GluonUserOptInVisual.class, "GluonUserOptInVisual.jLabel3.text")); // NOI18N
org.openide.awt.Mnemonics.setLocalizedText(jLabel4, org.openide.util.NbBundle.getMessage(GluonUserOptInVisual.class, "GluonUserOptInVisual.jLabel4.text")); // NOI18N
org.openide.awt.Mnemonics.setLocalizedText(jLabel5, org.openide.util.NbBundle.getMessage(GluonUserOptInVisual.class, "GluonUserOptInVisual.jLabel5.text")); // NOI18N
mobileLicenseTextField.setText(org.openide.util.NbBundle.getMessage(GluonUserOptInVisual.class, "GluonUserOptInVisual.mobileLicenseTextField.text")); // NOI18N
desktopLicenseTextField.setText(org.openide.util.NbBundle.getMessage(GluonUserOptInVisual.class, "GluonUserOptInVisual.desktopLicenseTextField.text")); // NOI18N
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(26, 26, 26)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel3, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(layout.createSequentialGroup()
.addComponent(emailLabel)
.addGap(79, 79, 79)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(uptodateCheckbox)
.addGap(0, 223, Short.MAX_VALUE))
.addComponent(emailTextField)))
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel2)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 376, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(0, 0, Short.MAX_VALUE))
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel4)
.addComponent(jLabel5))
.addGap(38, 38, 38)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(mobileLicenseTextField)
.addComponent(desktopLicenseTextField))))
.addGap(34, 34, 34))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(14, 14, 14)
.addComponent(jLabel1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel2)
.addGap(27, 27, 27)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(emailTextField, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(emailLabel))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(uptodateCheckbox)
.addGap(18, 18, 18)
.addComponent(jLabel3)
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel4)
.addComponent(mobileLicenseTextField, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel5)
.addComponent(desktopLicenseTextField, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(89, Short.MAX_VALUE))
);
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JTextField desktopLicenseTextField;
private javax.swing.JLabel emailLabel;
private javax.swing.JTextField emailTextField;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JTextField mobileLicenseTextField;
private javax.swing.JCheckBox uptodateCheckbox;
// End of variables declaration//GEN-END:variables
@Override
public void addNotify() {
super.addNotify();
//same problem as in 31086, initial focus on Cancel button
emailTextField.requestFocus();
}
@Override
protected boolean valid(WizardDescriptor wizardDescriptor) {
if (!TemplateUtils.isValidEmail(emailTextField.getText())) {
wizardDescriptor.putProperty("WizardPanel_errorMessage", "A valid email address is required");
return false;
}
wizardDescriptor.putProperty("WizardPanel_errorMessage", "");
return true;
}
@Override
protected void store(WizardDescriptor d) {
String email = emailTextField.getText().trim();
boolean uptodate = uptodateCheckbox.isSelected();
String mobileLicense = mobileLicenseTextField.getText();
String desktopLicense = desktopLicenseTextField.getText();
d.putProperty(ProjectConstants.PARAM_USER_EMAIL, email);
d.putProperty(ProjectConstants.PARAM_USER_UPTODATE, uptodate);
d.putProperty(ProjectConstants.PARAM_USER_LICENSE_MOBILE, mobileLicense);
d.putProperty(ProjectConstants.PARAM_USER_LICENSE_DESKTOP, desktopLicense);
d.putProperty(ProjectConstants.PARAM_USER_MAC_ADDRESS, TemplateUtils.getMacAddress());
d.putProperty(ProjectConstants.PARAM_USER_PLUGIN_VERSION, ProjectConstants.PLUGIN_VERSION);
}
@Override
protected void read(WizardDescriptor settings) {
this.emailTextField.selectAll();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy