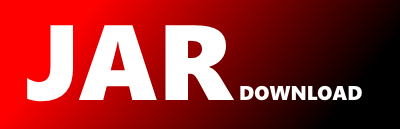
com.amazonaws.services.cognitoidentity.AmazonCognitoIdentityClient Maven / Gradle / Ivy
/*
* Copyright 2010-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.cognitoidentity;
import java.util.*;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.services.cognitoidentity.model.*;
import com.amazonaws.services.cognitoidentity.model.transform.*;
/**
* Client for accessing Amazon Cognito Identity. All service calls made using
* this client are blocking, and will not return until the service call
* completes.
*
* Amazon Cognito
*
* Amazon Cognito is a web service that delivers scoped temporary credentials to
* mobile devices and other untrusted environments. Amazon Cognito uniquely
* identifies a device and supplies the user with a consistent identity over the
* lifetime of an application.
*
*
* Using Amazon Cognito, you can enable authentication with one or more
* third-party identity providers (Facebook, Google, or Login with Amazon), and
* you can also choose to support unauthenticated access from your app. Cognito
* delivers a unique identifier for each user and acts as an OpenID token
* provider trusted by AWS Security Token Service (STS) to access temporary,
* limited-privilege AWS credentials.
*
*
* To provide end-user credentials, first make an unsigned call to GetId.
* If the end user is authenticated with one of the supported identity
* providers, set the Logins
map with the identity provider token.
* GetId
returns a unique identifier for the user.
*
*
* Next, make an unsigned call to GetCredentialsForIdentity. This call
* expects the same Logins
map as the GetId
call, as
* well as the IdentityID
originally returned by GetId
* . Assuming your identity pool has been configured via the
* SetIdentityPoolRoles operation, GetCredentialsForIdentity
* will return AWS credentials for your use. If your pool has not been
* configured with SetIdentityPoolRoles
, or if you want to follow
* legacy flow, make an unsigned call to GetOpenIdToken, which returns
* the OpenID token necessary to call STS and retrieve AWS credentials. This
* call expects the same Logins
map as the GetId
call,
* as well as the IdentityID
originally returned by
* GetId
. The token returned by GetOpenIdToken
can be
* passed to the STS operation AssumeRoleWithWebIdentity to retrieve AWS credentials.
*
*
* If you want to use Amazon Cognito in an Android, iOS, or Unity application,
* you will probably want to make API calls via the AWS Mobile SDK. To learn
* more, see the AWS
* Mobile SDK Developer Guide.
*
*/
public class AmazonCognitoIdentityClient extends AmazonWebServiceClient implements
AmazonCognitoIdentity {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
/**
* List of exception unmarshallers for all Amazon Cognito Identity
* exceptions.
*/
protected List jsonErrorUnmarshallers;
/**
* Constructs a new client to invoke service methods on
* AmazonCognitoIdentity. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
@Deprecated
public AmazonCognitoIdentityClient() {
this(new DefaultAWSCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonCognitoIdentity. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param clientConfiguration The client configuration options controlling
* how this client connects to AmazonCognitoIdentity (ex: proxy
* settings, retry counts, etc.).
* @see DefaultAWSCredentialsProviderChain
*/
@Deprecated
public AmazonCognitoIdentityClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on
* AmazonCognitoIdentity using the specified AWS account credentials.
*
* If AWS session credentials are passed in, then those credentials will be
* used to authenticate requests. Otherwise, if AWS long-term credentials
* are passed in, then session management will be handled automatically by
* the SDK. Callers are encouraged to use long-term credentials and let the
* SDK handle starting and renewing sessions.
*
* Automatically managed sessions will be shared among all clients that use
* the same credentials and service endpoint. To opt out of this behavior,
* explicitly provide an instance of {@link AWSCredentialsProvider} that
* returns {@link AWSSessionCredentials}.
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key)
* to use when authenticating with AWS services.
*/
public AmazonCognitoIdentityClient(AWSCredentials awsCredentials) {
this(awsCredentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonCognitoIdentity using the specified AWS account credentials and
* client configuration options.
*
* If AWS session credentials are passed in, then those credentials will be
* used to authenticate requests. Otherwise, if AWS long-term credentials
* are passed in, then session management will be handled automatically by
* the SDK. Callers are encouraged to use long-term credentials and let the
* SDK handle starting and renewing sessions.
*
* Automatically managed sessions will be shared among all clients that use
* the same credentials and service endpoint. To opt out of this behavior,
* explicitly provide an instance of {@link AWSCredentialsProvider} that
* returns {@link AWSSessionCredentials}.
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key)
* to use when authenticating with AWS services.
* @param clientConfiguration The client configuration options controlling
* how this client connects to AmazonCognitoIdentity (ex: proxy
* settings, retry counts, etc.).
*/
public AmazonCognitoIdentityClient(AWSCredentials awsCredentials,
ClientConfiguration clientConfiguration) {
this(new StaticCredentialsProvider(awsCredentials), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on
* AmazonCognitoIdentity using the specified AWS account credentials
* provider.
*
* If AWS session credentials are passed in, then those credentials will be
* used to authenticate requests. Otherwise, if AWS long-term credentials
* are passed in, then session management will be handled automatically by
* the SDK. Callers are encouraged to use long-term credentials and let the
* SDK handle starting and renewing sessions.
*
* Automatically managed sessions will be shared among all clients that use
* the same credentials and service endpoint. To opt out of this behavior,
* explicitly provide an instance of {@link AWSCredentialsProvider} that
* returns {@link AWSSessionCredentials}.
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider The AWS credentials provider which will
* provide credentials to authenticate requests with AWS
* services.
*/
public AmazonCognitoIdentityClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonCognitoIdentity using the specified AWS account credentials
* provider and client configuration options.
*
* If AWS session credentials are passed in, then those credentials will be
* used to authenticate requests. Otherwise, if AWS long-term credentials
* are passed in, then session management will be handled automatically by
* the SDK. Callers are encouraged to use long-term credentials and let the
* SDK handle starting and renewing sessions.
*
* Automatically managed sessions will be shared among all clients that use
* the same credentials and service endpoint. To opt out of this behavior,
* explicitly provide an instance of {@link AWSCredentialsProvider} that
* returns {@link AWSSessionCredentials}.
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider The AWS credentials provider which will
* provide credentials to authenticate requests with AWS
* services.
* @param clientConfiguration The client configuration options controlling
* how this client connects to AmazonCognitoIdentity (ex: proxy
* settings, retry counts, etc.).
*/
public AmazonCognitoIdentityClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, new UrlHttpClient(clientConfiguration));
}
/**
* Constructs a new client to invoke service methods on
* AmazonCognitoIdentity using the specified AWS account credentials
* provider, client configuration options and request metric collector.
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider The AWS credentials provider which will
* provide credentials to authenticate requests with AWS
* services.
* @param clientConfiguration The client configuration options controlling
* how this client connects to AmazonCognitoIdentity (ex: proxy
* settings, retry counts, etc.).
* @param requestMetricCollector optional request metric collector
*/
@Deprecated
public AmazonCognitoIdentityClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(adjustClientConfiguration(clientConfiguration), requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
/**
* Constructs a new client to invoke service methods on
* AmazonCognitoIdentity using the specified AWS account credentials
* provider, client configuration options and request metric collector.
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider The AWS credentials provider which will
* provide credentials to authenticate requests with AWS
* services.
* @param clientConfiguration The client configuration options controlling
* how this client connects to AmazonCognitoIdentity (ex: proxy
* settings, retry counts, etc.).
* @param httpClient A http client
*/
public AmazonCognitoIdentityClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration, HttpClient httpClient) {
super(adjustClientConfiguration(clientConfiguration), httpClient);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
jsonErrorUnmarshallers = new ArrayList();
jsonErrorUnmarshallers.add(new ExternalServiceExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new InternalErrorExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new InvalidIdentityPoolConfigurationExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new InvalidParameterExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new LimitExceededExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new NotAuthorizedExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new ResourceConflictExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new ResourceNotFoundExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new TooManyRequestsExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new JsonErrorUnmarshaller());
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("cognito-identity.us-east-1.amazonaws.com");
this.endpointPrefix = "cognito-identity";
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain(
"/com/amazonaws/services/cognitoidentity/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain(
"/com/amazonaws/services/cognitoidentity/request.handler2s"));
}
private static ClientConfiguration adjustClientConfiguration(ClientConfiguration orig) {
ClientConfiguration config = orig;
return config;
}
/**
*
* Returns credentials for the provided identity ID. Any provided logins
* will be validated against supported login providers. If the token is for
* cognito-identity.amazonaws.com, it will be passed through to AWS Security
* Token Service with the appropriate role for the token.
*
*
* This is a public API. You do not need any credentials to call this API.
*
*
* @param getCredentialsForIdentityRequest
* Input to the GetCredentialsForIdentity
action.
*
* @return getCredentialsForIdentityResult The response from the
* GetCredentialsForIdentity service method, as returned by Amazon
* Cognito Identity.
* @throws InvalidParameterException
* @throws ResourceNotFoundException
* @throws NotAuthorizedException
* @throws ResourceConflictException
* @throws TooManyRequestsException
* @throws InvalidIdentityPoolConfigurationException
* @throws InternalErrorException
* @throws ExternalServiceException
* @throws AmazonClientException If any internal errors are encountered
* inside the client while attempting to make the request or
* handle the response. For example if a network connection is
* not available.
* @throws AmazonServiceException If an error response is returned by Amazon
* Cognito Identity indicating either a problem with the data in
* the request, or a server side issue.
*/
public GetCredentialsForIdentityResult getCredentialsForIdentity(
GetCredentialsForIdentityRequest getCredentialsForIdentityRequest)
throws AmazonServiceException, AmazonClientException {
ExecutionContext executionContext = createExecutionContext(getCredentialsForIdentityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCredentialsForIdentityRequestMarshaller()
.marshall(getCredentialsForIdentityRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new GetCredentialsForIdentityResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(
unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Generates (or retrieves) a Cognito ID. Supplying multiple logins will
* create an implicit linked account.
*
*
* This is a public API. You do not need any credentials to call this API.
*
*
* @param getIdRequest Input to the GetId action.
* @return getIdResult The response from the GetId service method, as
* returned by Amazon Cognito Identity.
* @throws InvalidParameterException
* @throws ResourceNotFoundException
* @throws NotAuthorizedException
* @throws ResourceConflictException
* @throws TooManyRequestsException
* @throws InternalErrorException
* @throws LimitExceededException
* @throws ExternalServiceException
* @throws AmazonClientException If any internal errors are encountered
* inside the client while attempting to make the request or
* handle the response. For example if a network connection is
* not available.
* @throws AmazonServiceException If an error response is returned by Amazon
* Cognito Identity indicating either a problem with the data in
* the request, or a server side issue.
*/
public GetIdResult getId(GetIdRequest getIdRequest)
throws AmazonServiceException, AmazonClientException {
ExecutionContext executionContext = createExecutionContext(getIdRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetIdRequestMarshaller().marshall(getIdRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new GetIdResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(
unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Gets an OpenID token, using a known Cognito ID. This known Cognito ID is
* returned by GetId. You can optionally add additional logins for
* the identity. Supplying multiple logins creates an implicit link.
*
*
* The OpenId token is valid for 15 minutes.
*
*
* This is a public API. You do not need any credentials to call this API.
*
*
* @param getOpenIdTokenRequest Input to the GetOpenIdToken action.
* @return getOpenIdTokenResult The response from the GetOpenIdToken service
* method, as returned by Amazon Cognito Identity.
* @throws InvalidParameterException
* @throws ResourceNotFoundException
* @throws NotAuthorizedException
* @throws ResourceConflictException
* @throws TooManyRequestsException
* @throws InternalErrorException
* @throws ExternalServiceException
* @throws AmazonClientException If any internal errors are encountered
* inside the client while attempting to make the request or
* handle the response. For example if a network connection is
* not available.
* @throws AmazonServiceException If an error response is returned by Amazon
* Cognito Identity indicating either a problem with the data in
* the request, or a server side issue.
*/
public GetOpenIdTokenResult getOpenIdToken(GetOpenIdTokenRequest getOpenIdTokenRequest)
throws AmazonServiceException, AmazonClientException {
ExecutionContext executionContext = createExecutionContext(getOpenIdTokenRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetOpenIdTokenRequestMarshaller().marshall(getOpenIdTokenRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new GetOpenIdTokenResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(
unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
* Returns additional metadata for a previously executed successful,
* request, typically used for debugging issues where a service isn't acting
* as expected. This data isn't considered part of the result data returned
* by an operation, so it's available through this separate, diagnostic
* interface.
*
* Response metadata is only cached for a limited period of time, so if you
* need to access this extra diagnostic information for an executed request,
* you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request The originally executed request
* @return The response metadata for the specified request, or null if none
* is available.
* @deprecated ResponseMetadata cache can hold up to 50 requests and
* responses in memory and will cause memory issue. This method
* now always returns null.
*/
@Deprecated
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
private Response invoke(Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
AWSCredentials credentials;
awsRequestMetrics.startEvent(Field.CredentialsRequestTime);
try {
credentials = awsCredentialsProvider.getCredentials();
} finally {
awsRequestMetrics.endEvent(Field.CredentialsRequestTime);
}
AmazonWebServiceRequest originalRequest = request.getOriginalRequest();
if (originalRequest != null && originalRequest.getRequestCredentials() != null) {
credentials = originalRequest.getRequestCredentials();
}
executionContext.setCredentials(credentials);
JsonErrorResponseHandler errorResponseHandler = new JsonErrorResponseHandler(
jsonErrorUnmarshallers);
Response result = client.execute(request, responseHandler,
errorResponseHandler, executionContext);
return result;
}
}