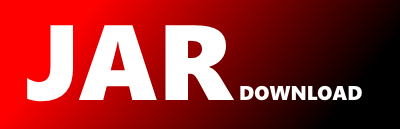
com.gluonhq.charm.down.common.NotificationsService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of charm-down-common Show documentation
Show all versions of charm-down-common Show documentation
API to access features for the mobile platforms
The newest version!
/*
* Copyright (c) 2015, Gluon
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of Gluon, any associated website, nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL GLUON BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.gluonhq.charm.down.common;
import com.gluonhq.charm.down.common.notifications.MockRemoteNotificationHandler;
import java.util.Arrays;
import java.util.EnumSet;
/**
* The interface to the platform notification services.
* Usage is as easy as:
*
* NotificationsService notificationsService = platform.getNotificationService();
* notificationsService.setRemoteNotificationHandler(myHandler);
* notificationsService.registerForNotificationTypes(NotificationType.Alert, NotificationType.Badge);
*
*/
public abstract class NotificationsService
{
/**
* The possible notification types you can register to.
* If a specific notification type is supported can be checked by calling
* {@link NotificationsService#isNotificationTypeSupported(NotificationType)}.
*/
public enum NotificationType
{
None,
Alert,
Sound,
Badge
}
/**
* The handler interface to process messages from the notifications system
*/
public interface RemoteNotificationHandler
{
/**
* received notification.
* @param notification A system dependent representation of the notification
*/
void receivedRemoteNotification(String notification);
/**
* called if an error happened during registration
* @param message A system dependent message of the failure
*/
void failToRegisterForRemoteNotifications(String message);
/**
* called if the registration succeeded
* @param deviceToken A system dependent token you have to use to send your messages to your device
*/
void didRegisterForRemoteNotifications(String deviceToken);
}
/**
* collection of {@link NotificationType}s used with the
* {@link NotificationsService#registerForNotificationTypes(NotificationType...)} call
*/
private EnumSet registeredNotificationTypes;
/**
* collection of {@link NotificationType}s the user allowed to receive
*/
protected EnumSet reportedNotificationTypes;
/**
* The notification handler
*/
private RemoteNotificationHandler remoteNotificationHandler = MockRemoteNotificationHandler.INSTANCE;
protected NotificationsService()
{
}
public RemoteNotificationHandler getRemoteNotificationHandler()
{
return remoteNotificationHandler;
}
/**
* setup your notification handler which will be called during the registration
* process or when a notification has been received.
* @param remoteNotificationHandler The notification handler
*/
public void setRemoteNotificationHandler(RemoteNotificationHandler remoteNotificationHandler)
{
this.remoteNotificationHandler = remoteNotificationHandler == null?MockRemoteNotificationHandler.INSTANCE:remoteNotificationHandler;
}
/**
* actually to register for the given notification types.
* @param notificationTypes Given notification types
*/
public final void registerForNotificationTypes(NotificationType ... notificationTypes)
{
registeredNotificationTypes = EnumSet.copyOf(Arrays.asList(notificationTypes));
doRegisterForNotificationTypes(notificationTypes);
}
/**
* return the collection of notifications you used with the
* {@link #registerForNotificationTypes(NotificationType...)} call
* @return EnumSet of notification types
*/
public EnumSet getRegisteredNotificationTypes()
{
return registeredNotificationTypes;
}
/**
* return the collection of notifications usable for the application reported by the notification system.
* This collection might differ to {@link #getRegisteredNotificationTypes()} e.g. if the user did not permit
* all notifications you requested.
* @return EnumSet of notification types
*/
public EnumSet getReportedNotificationTypes()
{
return reportedNotificationTypes;
}
/**
* Check to see if the given {@link NotificationType} is supported by the notification sytem
* @param notificationTypes notification type
* @return boolean
*/
public abstract boolean isNotificationTypeSupported(NotificationType notificationTypes);
protected abstract void doRegisterForNotificationTypes(NotificationType ... notificationTypes);
/**
* return the collection of notification currently active.
* This collection might differ to {@link #getReportedNotificationTypes()}
* e.g. if the user changed the permitted notification after program setup.
* @return EnumSet of notification types
*/
public abstract EnumSet getCurrentNotificationTypes();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy