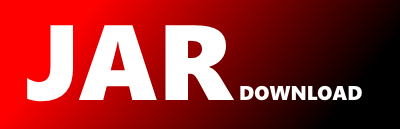
com.gluonhq.charm.down.common.ble.ScanDetection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of charm-down-common Show documentation
Show all versions of charm-down-common Show documentation
API to access features for the mobile platforms
The newest version!
/*
* Copyright (c) 2016, Gluon
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of Gluon, any associated website, nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL GLUON BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.gluonhq.charm.down.common.ble;
/**
* ScanDetection is a wrapper class to hold the main data obtained when scanning a beacon:
* uuid, major and minor ids, rssi and proximity estimation
*/
public class ScanDetection {
/**
* PROXIMITY is based on the estimated distance between beacon and device. It just gives a general sense of distance
* - iOS iBeacon protocol returns this value:
* https://developer.apple.com/library/ios/documentation/CoreLocation/Reference/CLBeacon_class/index.html#//apple_ref/occ/instm/CLBeacon/proximity
* - Android implementation has to provide it. Based on this:
* https://github.com/RadiusNetworks/android-ibeacon-service/blob/tip/src/main/java/com/radiusnetworks/ibeacon/IBeacon.java
*/
public enum PROXIMITY {
FAR(3),
NEAR(2),
IMMEDIATE(1),
UNKNOWN(0);
int proximity;
PROXIMITY(int proximity) {
this.proximity = proximity;
}
public int getProximity() {
return proximity;
}
}
/**
* Each beacon transmits its uuid, major and minor id values, and measured power.
* - Measured Power: a factory-calibrated, read-only constant which indicates what's the expected RSSI at a
* distance of 1 meter to the beacon
* - Broadcasting Power is the power with which the beacon broadcasts its signal. The more power, the longer the range,
* affecting the battery life.
* - Received Signal Strength Indicator (RSSI). It is the strength of the beacon's signal as seen on the receiving device.
* The signal strength depends on distance and Broadcasting Power value.
*
*/
private String uuid;
private int major;
private int minor;
private int rssi;
private PROXIMITY proximity;
public String getUuid() {
return uuid;
}
public void setUuid(String uuid) {
this.uuid = uuid;
}
public int getMajor() {
return major;
}
public void setMajor(int major) {
this.major = major;
}
public int getMinor() {
return minor;
}
public void setMinor(int minor) {
this.minor = minor;
}
public int getRssi() {
return rssi;
}
public void setRssi(int rssi) {
this.rssi = rssi;
}
public PROXIMITY getProximity() {
return proximity;
}
public void setProximity(int proximity) {
for( PROXIMITY p : PROXIMITY.values()) {
if (p.getProximity() == proximity) {
this.proximity = p;
break;
}
}
}
@Override
public String toString() {
return "ScanDetection{" + ", uuid=" + uuid + ", major=" + major + ", minor=" + minor +
"rssi=" + rssi + ", proximity=" + proximity.name() + '}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy