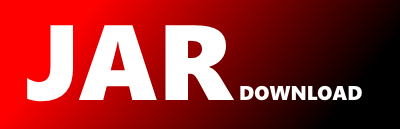
com.gocardless.http.ListRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gocardless-pro Show documentation
Show all versions of gocardless-pro Show documentation
Client library for accessing the GoCardless Pro API
package com.gocardless.http;
import com.google.common.collect.ImmutableMap;
import com.google.gson.reflect.TypeToken;
import java.util.List;
import java.util.Map;
/**
* Base class for GET requests that return multiple items.
*
* @param the type of the item returned by this request.
*/
public abstract class ListRequest extends ApiRequest> {
private final transient ListRequestExecutor executor;
private final transient String method;
private String after;
private String before;
private Integer limit;
protected ListRequest(HttpClient httpClient, ListRequestExecutor executor) {
this(httpClient, executor, "GET");
}
protected ListRequest(HttpClient httpClient, ListRequestExecutor executor,
String method) {
super(httpClient);
this.method = method;
this.executor = executor;
}
/**
* Executes this request.
*
* Returns the API response.
*
* @throws com.gocardless.GoCardlessException
*/
public S execute() {
return executor.execute(this, getHttpClient());
}
/**
* Executes this request.
*
* Returns a {@link com.gocardless.http.ApiResponse} that wraps the response entity.
*
* @throws com.gocardless.GoCardlessException
*/
public ApiResponse executeWrapped() {
return executor.executeWrapped(this, getHttpClient());
}
@Override
protected ListResponse parseResponse(String responseBody, ResponseParser responseParser) {
return responseParser.parsePage(responseBody, getEnvelope(), getTypeToken());
}
@Override
protected final String getMethod() {
return this.method;
}
@Override
protected boolean hasBody() {
return false;
}
@Override
protected Map getQueryParams() {
ImmutableMap.Builder params = ImmutableMap.builder();
if (after != null) {
params.put("after", after);
}
if (before != null) {
params.put("before", before);
}
if (limit != null) {
params.put("limit", limit);
}
return params.build();
}
protected abstract TypeToken> getTypeToken();
protected void setAfter(String after) {
this.after = after;
}
protected void setBefore(String before) {
this.before = before;
}
protected void setLimit(Integer limit) {
this.limit = limit;
}
public interface ListRequestExecutor {
S execute(ListRequest request, HttpClient client);
ApiResponse executeWrapped(ListRequest request, HttpClient client);
}
public static ListRequestExecutor, T> pagingExecutor() {
return new ListRequestExecutor, T>() {
@Override
public ListResponse execute(ListRequest, T> request,
HttpClient client) {
return client.executeWithRetries(request);
}
@Override
public ApiResponse> executeWrapped(
ListRequest, T> request, HttpClient client) {
return client.executeWrapped(request);
}
};
}
public static ListRequestExecutor, T> iteratingExecutor() {
return new ListRequestExecutor, T>() {
@Override
public Iterable execute(ListRequest, T> request, HttpClient client) {
return new PaginatingIterable<>(request, client);
}
@Override
public ApiResponse> executeWrapped(ListRequest, T> request,
HttpClient client) {
throw new IllegalStateException(
"executeWrapped not available when iterating through list responses");
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy