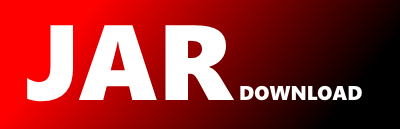
com.gocardless.services.EventService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gocardless-pro Show documentation
Show all versions of gocardless-pro Show documentation
Client library for accessing the GoCardless Pro API
package com.gocardless.services;
import com.gocardless.http.*;
import com.gocardless.resources.Event;
import com.google.common.collect.ImmutableMap;
import com.google.gson.annotations.SerializedName;
import com.google.gson.reflect.TypeToken;
import java.util.List;
import java.util.Map;
/**
* Service class for working with event resources.
*
* Events are stored for all webhooks. An event refers to a resource which has been updated, for
* example a payment which has been collected, or a mandate which has been transferred. Event
* creation is an asynchronous process, so it can take some time between an action occurring and its
* corresponding event getting included in API responses. See [here](#event-actions) for a complete
* list of event types.
*/
public class EventService {
private final HttpClient httpClient;
/**
* Constructor. Users of this library should have no need to call this - an instance of this
* class can be obtained by calling {@link com.gocardless.GoCardlessClient#events() }.
*/
public EventService(HttpClient httpClient) {
this.httpClient = httpClient;
}
/**
* Returns a [cursor-paginated](#api-usage-cursor-pagination) list of your events.
*/
public EventListRequest> list() {
return new EventListRequest<>(httpClient, ListRequest.pagingExecutor());
}
public EventListRequest> all() {
return new EventListRequest<>(httpClient, ListRequest.iteratingExecutor());
}
/**
* Retrieves the details of a single event.
*/
public EventGetRequest get(String identity) {
return new EventGetRequest(httpClient, identity);
}
/**
* Request class for {@link EventService#list }.
*
* Returns a [cursor-paginated](#api-usage-cursor-pagination) list of your events.
*/
public static final class EventListRequest extends ListRequest {
private String action;
private String billingRequest;
private CreatedAt createdAt;
private String creditor;
private Include include;
private String instalmentSchedule;
private String mandate;
private String parentEvent;
private String payerAuthorisation;
private String payment;
private String payout;
private String refund;
private ResourceType resourceType;
private String schemeIdentifier;
private String subscription;
/**
* Limit to events with a given `action`.
*/
public EventListRequest withAction(String action) {
this.action = action;
return this;
}
/**
* Cursor pointing to the start of the desired set.
*/
public EventListRequest withAfter(String after) {
setAfter(after);
return this;
}
/**
* Cursor pointing to the end of the desired set.
*/
public EventListRequest withBefore(String before) {
setBefore(before);
return this;
}
/**
* ID of a [billing request](#billing-requests-billing-requests). If specified, this
* endpoint will return all events for the given billing request.
*/
public EventListRequest withBillingRequest(String billingRequest) {
this.billingRequest = billingRequest;
return this;
}
public EventListRequest withCreatedAt(CreatedAt createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Limit to records created after the specified date-time.
*/
public EventListRequest withCreatedAtGt(String gt) {
if (createdAt == null) {
createdAt = new CreatedAt();
}
createdAt.withGt(gt);
return this;
}
/**
* Limit to records created on or after the specified date-time.
*/
public EventListRequest withCreatedAtGte(String gte) {
if (createdAt == null) {
createdAt = new CreatedAt();
}
createdAt.withGte(gte);
return this;
}
/**
* Limit to records created before the specified date-time.
*/
public EventListRequest withCreatedAtLt(String lt) {
if (createdAt == null) {
createdAt = new CreatedAt();
}
createdAt.withLt(lt);
return this;
}
/**
* Limit to records created on or before the specified date-time.
*/
public EventListRequest withCreatedAtLte(String lte) {
if (createdAt == null) {
createdAt = new CreatedAt();
}
createdAt.withLte(lte);
return this;
}
/**
* ID of an [creditor](#core-endpoints-creditors). If specified, this endpoint will return
* all events for the given creditor.
*/
public EventListRequest withCreditor(String creditor) {
this.creditor = creditor;
return this;
}
/**
* Includes linked resources in the response. Must be used with the `resource_type`
* parameter specified. The include should be one of:
*
* - `billing_request`
* - `creditor`
* - `instalment_schedule`
* - `mandate`
* - `payer_authorisation`
* - `payment`
* - `payout`
* - `refund`
* - `scheme_identifier`
* - `subscription`
*
*/
public EventListRequest withInclude(Include include) {
this.include = include;
return this;
}
/**
* ID of an [instalment schedule](#core-endpoints-instalment-schedules). If specified, this
* endpoint will return all events for the given instalment schedule.
*/
public EventListRequest withInstalmentSchedule(String instalmentSchedule) {
this.instalmentSchedule = instalmentSchedule;
return this;
}
/**
* Number of records to return.
*/
public EventListRequest withLimit(Integer limit) {
setLimit(limit);
return this;
}
/**
* ID of a [mandate](#core-endpoints-mandates). If specified, this endpoint will return all
* events for the given mandate.
*/
public EventListRequest withMandate(String mandate) {
this.mandate = mandate;
return this;
}
/**
* ID of an event. If specified, this endpoint will return all events whose parent_event is
* the given event ID.
*/
public EventListRequest withParentEvent(String parentEvent) {
this.parentEvent = parentEvent;
return this;
}
/**
* ID of a [payer authorisation](#core-endpoints-payer-authorisations).
*/
public EventListRequest withPayerAuthorisation(String payerAuthorisation) {
this.payerAuthorisation = payerAuthorisation;
return this;
}
/**
* ID of a [payment](#core-endpoints-payments). If specified, this endpoint will return all
* events for the given payment.
*/
public EventListRequest withPayment(String payment) {
this.payment = payment;
return this;
}
/**
* ID of a [payout](#core-endpoints-payouts). If specified, this endpoint will return all
* events for the given payout.
*/
public EventListRequest withPayout(String payout) {
this.payout = payout;
return this;
}
/**
* ID of a [refund](#core-endpoints-refunds). If specified, this endpoint will return all
* events for the given refund.
*/
public EventListRequest withRefund(String refund) {
this.refund = refund;
return this;
}
/**
* Type of resource that you'd like to get all events for. Cannot be used together with the
* `billing_request`, `creditor`, `instalment_schedule`, `mandate`, `payer_authorisation`,
* `payment`, `payout`, `refund`, `scheme_identifier` or `subscription` parameters. The type
* can be one of:
*
* - `billing_requests`
* - `creditors`
* - `instalment_schedules`
* - `mandates`
* - `payer_authorisations`
* - `payments`
* - `payouts`
* - `refunds`
* - `scheme_identifiers`
* - `subscriptions`
*
*/
public EventListRequest withResourceType(ResourceType resourceType) {
this.resourceType = resourceType;
return this;
}
/**
* ID of a [scheme identifier](#core-endpoints-scheme-identifiers). If specified, this
* endpoint will return all events for the given scheme identifier.
*/
public EventListRequest withSchemeIdentifier(String schemeIdentifier) {
this.schemeIdentifier = schemeIdentifier;
return this;
}
/**
* ID of a [subscription](#core-endpoints-subscriptions). If specified, this endpoint will
* return all events for the given subscription.
*/
public EventListRequest withSubscription(String subscription) {
this.subscription = subscription;
return this;
}
private EventListRequest(HttpClient httpClient, ListRequestExecutor executor) {
super(httpClient, executor);
}
public EventListRequest withHeader(String headerName, String headerValue) {
this.addHeader(headerName, headerValue);
return this;
}
@Override
protected Map getQueryParams() {
ImmutableMap.Builder params = ImmutableMap.builder();
params.putAll(super.getQueryParams());
if (action != null) {
params.put("action", action);
}
if (billingRequest != null) {
params.put("billing_request", billingRequest);
}
if (createdAt != null) {
params.putAll(createdAt.getQueryParams());
}
if (creditor != null) {
params.put("creditor", creditor);
}
if (include != null) {
params.put("include", include);
}
if (instalmentSchedule != null) {
params.put("instalment_schedule", instalmentSchedule);
}
if (mandate != null) {
params.put("mandate", mandate);
}
if (parentEvent != null) {
params.put("parent_event", parentEvent);
}
if (payerAuthorisation != null) {
params.put("payer_authorisation", payerAuthorisation);
}
if (payment != null) {
params.put("payment", payment);
}
if (payout != null) {
params.put("payout", payout);
}
if (refund != null) {
params.put("refund", refund);
}
if (resourceType != null) {
params.put("resource_type", resourceType);
}
if (schemeIdentifier != null) {
params.put("scheme_identifier", schemeIdentifier);
}
if (subscription != null) {
params.put("subscription", subscription);
}
return params.build();
}
@Override
protected String getPathTemplate() {
return "events";
}
@Override
protected String getEnvelope() {
return "events";
}
@Override
protected TypeToken> getTypeToken() {
return new TypeToken>() {};
}
public enum Include {
@SerializedName("billing_request")
BILLING_REQUEST, @SerializedName("creditor")
CREDITOR, @SerializedName("instalment_schedule")
INSTALMENT_SCHEDULE, @SerializedName("mandate")
MANDATE, @SerializedName("payer_authorisation")
PAYER_AUTHORISATION, @SerializedName("payment")
PAYMENT, @SerializedName("payout")
PAYOUT, @SerializedName("refund")
REFUND, @SerializedName("scheme_identifier")
SCHEME_IDENTIFIER, @SerializedName("subscription")
SUBSCRIPTION, @SerializedName("unknown")
UNKNOWN;
@Override
public String toString() {
return name().toLowerCase();
}
}
public enum ResourceType {
@SerializedName("billing_requests")
BILLING_REQUESTS, @SerializedName("creditors")
CREDITORS, @SerializedName("instalment_schedules")
INSTALMENT_SCHEDULES, @SerializedName("mandates")
MANDATES, @SerializedName("organisations")
ORGANISATIONS, @SerializedName("payer_authorisations")
PAYER_AUTHORISATIONS, @SerializedName("payments")
PAYMENTS, @SerializedName("payouts")
PAYOUTS, @SerializedName("refunds")
REFUNDS, @SerializedName("scheme_identifiers")
SCHEME_IDENTIFIERS, @SerializedName("subscriptions")
SUBSCRIPTIONS, @SerializedName("unknown")
UNKNOWN;
@Override
public String toString() {
return name().toLowerCase();
}
}
public static class CreatedAt {
private String gt;
private String gte;
private String lt;
private String lte;
/**
* Limit to records created after the specified date-time.
*/
public CreatedAt withGt(String gt) {
this.gt = gt;
return this;
}
/**
* Limit to records created on or after the specified date-time.
*/
public CreatedAt withGte(String gte) {
this.gte = gte;
return this;
}
/**
* Limit to records created before the specified date-time.
*/
public CreatedAt withLt(String lt) {
this.lt = lt;
return this;
}
/**
* Limit to records created on or before the specified date-time.
*/
public CreatedAt withLte(String lte) {
this.lte = lte;
return this;
}
public Map getQueryParams() {
ImmutableMap.Builder params = ImmutableMap.builder();
if (gt != null) {
params.put("created_at[gt]", gt);
}
if (gte != null) {
params.put("created_at[gte]", gte);
}
if (lt != null) {
params.put("created_at[lt]", lt);
}
if (lte != null) {
params.put("created_at[lte]", lte);
}
return params.build();
}
}
}
/**
* Request class for {@link EventService#get }.
*
* Retrieves the details of a single event.
*/
public static final class EventGetRequest extends GetRequest {
@PathParam
private final String identity;
private EventGetRequest(HttpClient httpClient, String identity) {
super(httpClient);
this.identity = identity;
}
public EventGetRequest withHeader(String headerName, String headerValue) {
this.addHeader(headerName, headerValue);
return this;
}
@Override
protected Map getPathParams() {
ImmutableMap.Builder params = ImmutableMap.builder();
params.put("identity", identity);
return params.build();
}
@Override
protected String getPathTemplate() {
return "events/:identity";
}
@Override
protected String getEnvelope() {
return "events";
}
@Override
protected Class getResponseClass() {
return Event.class;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy