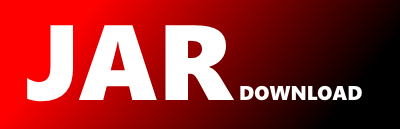
com.gocardless.services.TaxRateService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gocardless-pro Show documentation
Show all versions of gocardless-pro Show documentation
Client library for accessing the GoCardless Pro API
package com.gocardless.services;
import com.gocardless.http.*;
import com.gocardless.resources.TaxRate;
import com.google.common.collect.ImmutableMap;
import com.google.gson.reflect.TypeToken;
import java.util.List;
import java.util.Map;
/**
* Service class for working with tax rate resources.
*
* Tax rates from tax authority.
*
* We also maintain a [static list of the tax rates for each jurisdiction](#appendix-tax-rates).
*/
public class TaxRateService {
private final HttpClient httpClient;
/**
* Constructor. Users of this library should have no need to call this - an instance of this
* class can be obtained by calling {@link com.gocardless.GoCardlessClient#taxRates() }.
*/
public TaxRateService(HttpClient httpClient) {
this.httpClient = httpClient;
}
/**
* Returns a [cursor-paginated](#api-usage-cursor-pagination) list of all tax rates.
*/
public TaxRateListRequest> list() {
return new TaxRateListRequest<>(httpClient, ListRequest.pagingExecutor());
}
public TaxRateListRequest> all() {
return new TaxRateListRequest<>(httpClient, ListRequest.iteratingExecutor());
}
/**
* Retrieves the details of a tax rate.
*/
public TaxRateGetRequest get(String identity) {
return new TaxRateGetRequest(httpClient, identity);
}
/**
* Request class for {@link TaxRateService#list }.
*
* Returns a [cursor-paginated](#api-usage-cursor-pagination) list of all tax rates.
*/
public static final class TaxRateListRequest extends ListRequest {
private String jurisdiction;
/**
* Cursor pointing to the start of the desired set.
*/
public TaxRateListRequest withAfter(String after) {
setAfter(after);
return this;
}
/**
* Cursor pointing to the end of the desired set.
*/
public TaxRateListRequest withBefore(String before) {
setBefore(before);
return this;
}
/**
* The jurisdiction this tax rate applies to
*/
public TaxRateListRequest withJurisdiction(String jurisdiction) {
this.jurisdiction = jurisdiction;
return this;
}
/**
* Number of records to return.
*/
public TaxRateListRequest withLimit(Integer limit) {
setLimit(limit);
return this;
}
private TaxRateListRequest(HttpClient httpClient,
ListRequestExecutor executor) {
super(httpClient, executor);
}
public TaxRateListRequest withHeader(String headerName, String headerValue) {
this.addHeader(headerName, headerValue);
return this;
}
@Override
protected Map getQueryParams() {
ImmutableMap.Builder params = ImmutableMap.builder();
params.putAll(super.getQueryParams());
if (jurisdiction != null) {
params.put("jurisdiction", jurisdiction);
}
return params.build();
}
@Override
protected String getPathTemplate() {
return "tax_rates";
}
@Override
protected String getEnvelope() {
return "tax_rates";
}
@Override
protected TypeToken> getTypeToken() {
return new TypeToken>() {};
}
}
/**
* Request class for {@link TaxRateService#get }.
*
* Retrieves the details of a tax rate.
*/
public static final class TaxRateGetRequest extends GetRequest {
@PathParam
private final String identity;
private TaxRateGetRequest(HttpClient httpClient, String identity) {
super(httpClient);
this.identity = identity;
}
public TaxRateGetRequest withHeader(String headerName, String headerValue) {
this.addHeader(headerName, headerValue);
return this;
}
@Override
protected Map getPathParams() {
ImmutableMap.Builder params = ImmutableMap.builder();
params.put("identity", identity);
return params.build();
}
@Override
protected String getPathTemplate() {
return "tax_rates/:identity";
}
@Override
protected String getEnvelope() {
return "tax_rates";
}
@Override
protected Class getResponseClass() {
return TaxRate.class;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy