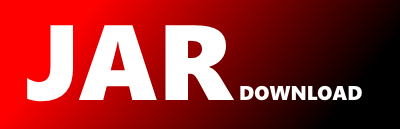
com.gocardless.services.ExportService Maven / Gradle / Ivy
package com.gocardless.services;
import com.gocardless.http.*;
import com.gocardless.resources.Export;
import com.google.common.collect.ImmutableMap;
import com.google.gson.reflect.TypeToken;
import java.util.List;
import java.util.Map;
/**
* Service class for working with export resources.
*
* File-based exports of data
*/
public class ExportService {
private final HttpClient httpClient;
/**
* Constructor. Users of this library should have no need to call this - an instance of this
* class can be obtained by calling {@link com.gocardless.GoCardlessClient#exports() }.
*/
public ExportService(HttpClient httpClient) {
this.httpClient = httpClient;
}
/**
* Returns a single export.
*/
public ExportGetRequest get(String identity) {
return new ExportGetRequest(httpClient, identity);
}
/**
* Returns a list of exports which are available for download.
*/
public ExportListRequest> list() {
return new ExportListRequest<>(httpClient, ListRequest.pagingExecutor());
}
public ExportListRequest> all() {
return new ExportListRequest<>(httpClient, ListRequest.iteratingExecutor());
}
/**
* Request class for {@link ExportService#get }.
*
* Returns a single export.
*/
public static final class ExportGetRequest extends GetRequest {
@PathParam
private final String identity;
private ExportGetRequest(HttpClient httpClient, String identity) {
super(httpClient);
this.identity = identity;
}
public ExportGetRequest withHeader(String headerName, String headerValue) {
this.addHeader(headerName, headerValue);
return this;
}
@Override
protected Map getPathParams() {
ImmutableMap.Builder params = ImmutableMap.builder();
params.put("identity", identity);
return params.build();
}
@Override
protected String getPathTemplate() {
return "exports/:identity";
}
@Override
protected String getEnvelope() {
return "exports";
}
@Override
protected Class getResponseClass() {
return Export.class;
}
}
/**
* Request class for {@link ExportService#list }.
*
* Returns a list of exports which are available for download.
*/
public static final class ExportListRequest extends ListRequest {
/**
* Cursor pointing to the start of the desired set.
*/
public ExportListRequest withAfter(String after) {
setAfter(after);
return this;
}
/**
* Cursor pointing to the end of the desired set.
*/
public ExportListRequest withBefore(String before) {
setBefore(before);
return this;
}
/**
* Number of records to return.
*/
public ExportListRequest withLimit(Integer limit) {
setLimit(limit);
return this;
}
private ExportListRequest(HttpClient httpClient, ListRequestExecutor executor) {
super(httpClient, executor);
}
public ExportListRequest withHeader(String headerName, String headerValue) {
this.addHeader(headerName, headerValue);
return this;
}
@Override
protected Map getQueryParams() {
ImmutableMap.Builder params = ImmutableMap.builder();
params.putAll(super.getQueryParams());
return params.build();
}
@Override
protected String getPathTemplate() {
return "exports";
}
@Override
protected String getEnvelope() {
return "exports";
}
@Override
protected TypeToken> getTypeToken() {
return new TypeToken>() {};
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy