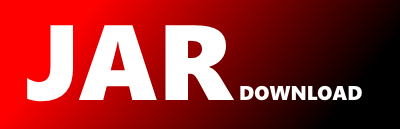
com.godmao.mqbroker.handler.BrokerDecoderHandler Maven / Gradle / Ivy
package com.godmao.mqbroker.handler;
import com.godmao.mqbroker.message.*;
import com.godmao.netty.handler.AbstractDecoderHandler;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandler;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
@ChannelHandler.Sharable
public class BrokerDecoderHandler extends AbstractDecoderHandler {
public static final BrokerDecoderHandler INSTANCE = new BrokerDecoderHandler();
@Override
public Object decode(ByteBuf msg) {
final byte cmd = msg.readByte();
Object decode = msg;
switch (cmd) {
case SingleMessage.cmd:// 消息转发 -单服务消息
final byte model = msg.readByte();// 消息模型
final long delay = msg.readLong();// 消息延时
//
final String topic = msg.readSlice(msg.readInt()).toString(StandardCharsets.UTF_8);
final byte[] body_byte = new byte[msg.readableBytes()];
msg.readBytes(body_byte);
decode = new SingleMessage.Request(model, delay, topic, body_byte);
break;
case MultipleMessage.cmd:// 消息转发 -多服务消息
final byte _model = msg.readByte();// 消息模型
final long _delay = msg.readLong();// 消息延时
//
final int _topic_lenth = msg.readInt();
final int _data_lenth = msg.readInt();
//
final List _topics = new ArrayList<>(_topic_lenth);
final List _body_bytes = new ArrayList<>(_data_lenth);
//
for (int i = 0; i < _topic_lenth; i++) {
final ByteBuf _byteBuf = msg.readSlice(msg.readInt());
final String _topic = _byteBuf.toString(StandardCharsets.UTF_8);
_topics.add(i, _topic);
}
for (int i = 0; i < _data_lenth; i++) {
final ByteBuf _byteBuf = msg.readSlice(msg.readInt());
final byte[] _body_byte = new byte[_byteBuf.readableBytes()];
_byteBuf.readBytes(_body_byte);
_body_bytes.add(i, _body_byte);
}
decode = new MultipleMessage.Request(_model, _delay, _topics, _body_bytes);
break;
case HeartbeatMessage.cmd:// 心跳
final long version = msg.readLong();
decode = new HeartbeatMessage.Request(version);
break;
case BindMessage.cmd:// 连接
final String name = msg.readSlice(msg.readInt()).toString(StandardCharsets.UTF_8);
final String address = msg.readSlice(msg.readableBytes()).toString(StandardCharsets.UTF_8);
decode = new BindMessage(name, address);
break;
case TopicSubscribeMessage.cmd:// 主题订阅
final int topic_lenth1 = msg.readInt();
final Set topics1 = new HashSet<>(topic_lenth1);
for (int i = 0; i < topic_lenth1; i++) {
topics1.add(msg.readSlice(msg.readInt()).toString(StandardCharsets.UTF_8));
}
decode = new TopicSubscribeMessage(topics1);
break;
case TopicSubscribeCancelMessage.cmd:// 取消订阅
final int topic_lenth2 = msg.readInt();
final Set topics2 = new HashSet<>(topic_lenth2);
for (int i = 0; i < topic_lenth2; i++) {
topics2.add(msg.readSlice(msg.readInt()).toString(StandardCharsets.UTF_8));
}
decode = new TopicSubscribeCancelMessage(topics2);
break;
}
return decode;
}
// public static void main(String[] args) {
// ByteBuf msg = ByteBufAllocator.DEFAULT.directBuffer();
// msg.writeBoolean(true);
// List topics = Arrays.asList("a", "b", "c", "d");
//// for (String topic : topics) {
//// msg.writeInt(topic.getBytes().length);
//// msg.writeBytes(topic.getBytes());
//// }
//
// String string1 = Arrays.toString(new String[]{"a", "b", "c", "d"});
//
// byte[] bytes = string1.getBytes();
// msg.writeBytes(bytes);
//
// boolean all = msg.readBoolean();
//
// String string = msg.toString(StandardCharsets.UTF_8);
// // 分割字符串为单个元素值
// String[] arr = string.replace("[", "").replace("]", "").split(", ");
//
//
// System.out.println(arr);
//
//
// final Set topics1 = new HashSet<>();
// while (msg.isReadable()) {
// topics1.add(msg.readSlice(msg.readInt()).toString(StandardCharsets.UTF_8));
// }
// TopicRegisterMessage decode = new TopicRegisterMessage(all, topics1);
// System.out.println(1);
// }
// public static void main(String[] args) {
// ByteBuf msg = ByteBufAllocator.DEFAULT.directBuffer();
// msg.writeByte(1);
// msg.writeLong(0L);
// msg.writeInt("topic".getBytes().length);
// msg.writeBytes("topic".getBytes());
// msg.writeBytes(new byte[]{1, 2, 3});
//
// final byte model = msg.readByte();
// final long delay = msg.readLong();
// //
// ByteBuf msg_slice = msg.slice();// top_size + top + data
// final String topic = msg.readSlice(msg.readInt()).toString(StandardCharsets.UTF_8);
// final byte[] body_byte = new byte[msg_slice.writerIndex()];
// msg_slice.readBytes(body_byte);
//
// ServerMessage decode = new ServerMessage(model, delay, topic, body_byte);
//
// msg.release();
// msg_slice.release();
// }
// public static void main(String[] args) {
// ByteBuf byteBuf1 = ByteBufAllocator.DEFAULT.directBuffer();
//
// String topic = "a";
// byte[] topic_bytes = topic.getBytes();
// byte[] bytes = new byte[]{1, 2, 3};
//
// byteBuf1.writeInt(topic_bytes.length);// 4
// byteBuf1.writeBytes(topic_bytes);// 1
// byteBuf1.writeBytes(bytes);// 3
//
//
// ByteBuf byteBuf2 = byteBuf1.readSlice(byteBuf1.readableBytes());// top_size + top + data
// ByteBuf byteBuf3 = byteBuf2.slice();
//
// String topic_ = byteBuf2.readSlice(byteBuf2.readInt()).toString(StandardCharsets.UTF_8);
// byte[] topic_bytes_ = topic_.getBytes();
//
// // 0 0 0 1 97 1 2 3
//
//// ByteBuf byteBuf3 = byteBuf2.slice();
//
// final byte[] bytes_ = new byte[byteBuf3.writerIndex()];
// byteBuf3.readBytes(bytes_);
//
// byteBuf1.release();
// System.out.println(1);
//
// }
// public static void main(String[] args) {
// ByteBuf byteBuf1 = ByteBufAllocator.DEFAULT.directBuffer();
// byteBuf1.writeBytes("godmao".getBytes());
//
//
// final byte[] body_byte = new byte[byteBuf1.readableBytes()];
// ByteBuf body_byteBuf = byteBuf1.readBytes(body_byte);
//
// System.out.println(new String(body_byte));
//
// body_byteBuf.release();
// byteBuf1.release();
//
//
// }
// public static void main(String[] args) {
// ByteBuf byteBuf1 = ByteBufAllocator.DEFAULT.directBuffer();
//
// Set topics = new HashSet<>();
// topics.add("godmao1");
// topics.add("godma1321o2");
//
//
// for (String topic : topics) {
// byte[] bytes = topic.getBytes();
// byteBuf1.writeInt(bytes.length);
// byteBuf1.writeBytes(bytes);
// }
//
// do {
// if (!byteBuf1.isReadable()) {
// break;
// }
// System.out.println(byteBuf1.readSlice(byteBuf1.readInt()).toString(StandardCharsets.UTF_8));
// System.out.println("====================");
//
// } while (true);
//
//
// ByteBuf byteBuf2 = byteBuf1.readSlice(byteBuf1.readInt());
// ByteBuf byteBuf3 = byteBuf1.readSlice(byteBuf1.readInt());
//
// System.out.println(byteBuf2.toString(StandardCharsets.UTF_8));
// System.out.println(byteBuf3.toString(StandardCharsets.UTF_8));
//
// byteBuf1.release();
// }
// public static void decode2(ByteBuf msg) {
// if (!msg.isReadable()) {
// return;
// }
// byte cmd = msg.readByte();
// if (cmd < 1) {
// return;
// }
//
// Object decode = null;
// switch (cmd) {
// case 1:// 消息转发
// System.err.println(111111111);
// break;
// case 3:// 主题订阅
// System.err.println(333333333);
// decode = new TopicRegisterMessage(msg.readBoolean(), null);
// break;
// case 4:// 取消订阅
// System.err.println(44444444);
// decode = new TopicCancelMessage(msg.readBoolean(), null);
// break;
// }
// System.out.println(555555555);
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy