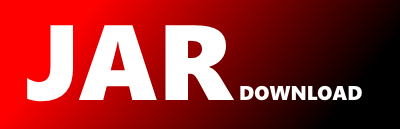
com.godmao.mqbroker.handler.SingleMessageHandler Maven / Gradle / Ivy
package com.godmao.mqbroker.handler;
import com.godmao.mqbroker.Broker;
import com.godmao.mqbroker.Static;
import com.godmao.mqbroker.message.SingleMessage;
import com.godmao.netty.channel.ChannelService;
import io.netty.channel.*;
import java.util.*;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicLong;
/**
* 服务器消息处理器
*/
public class SingleMessageHandler extends SimpleChannelInboundHandler {
private final Broker connect;
public SingleMessageHandler(Broker connect) {
this.connect = connect;
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, SingleMessage.Request msg) {
final byte[] _data = msg.getData();
final byte model = msg.getModel();
final long delay = msg.getDelay();
final String _topic = msg.getTopic();
final Runnable runnable = () -> {
// key channelid value channel(匹配到有主题的channel)
final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy