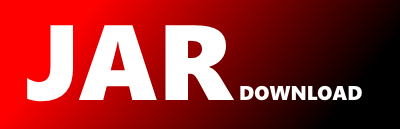
com.goeuro.sync4j.utils.Applications Maven / Gradle / Ivy
package com.goeuro.sync4j.utils;
import javax.annotation.Nonnull;
import java.io.IOException;
import java.io.InputStream;
import java.io.UncheckedIOException;
import java.time.OffsetDateTime;
import java.util.Optional;
import java.util.Properties;
import static java.time.format.DateTimeFormatter.ISO_OFFSET_DATE_TIME;
public final class Applications {
@Nonnull
private static final String PROPERTIES_FILENAME = "com/goeuro/sync4j/version.properties";
@Nonnull
private static final Properties PROPERTIES = loadProperties();
@Nonnull
private static final String VERSION = getProperty(PROPERTIES, "project.version");
@Nonnull
private static final String NAME = getProperty(PROPERTIES, "project.name");
@Nonnull
private static final OffsetDateTime BUILD_TIMESTAMP = getOffsetDateTimeProperty(PROPERTIES, "project.build.timestamp");
@Nonnull
public static String version() {
return VERSION;
}
@Nonnull
public static String name() {
return NAME;
}
@Nonnull
public static OffsetDateTime buildTimestamp() {
return BUILD_TIMESTAMP;
}
@Nonnull
private static OffsetDateTime getOffsetDateTimeProperty(@Nonnull Properties properties, @Nonnull String propertyName) {
final String plain = getProperty(properties, propertyName);
return OffsetDateTime.parse(plain, ISO_OFFSET_DATE_TIME);
}
@Nonnull
private static String getProperty(@Nonnull Properties properties, @Nonnull String propertyName) {
return findProperty(properties, propertyName)
.orElseThrow(() -> new IllegalStateException("'" + PROPERTIES_FILENAME + "' in classpath does not contain required property '" + propertyName + "'."))
;
}
@Nonnull
private static Optional findProperty(@Nonnull Properties properties, @Nonnull String propertyName) {
return Optional.ofNullable(properties.getProperty(propertyName));
}
@Nonnull
private static Properties loadProperties() {
final Properties properties = new Properties();
try (final InputStream is = Applications.class.getClassLoader().getResourceAsStream(PROPERTIES_FILENAME)) {
if (is == null) {
throw new IllegalStateException("The classpath does not contain '" + PROPERTIES_FILENAME + "'.");
}
properties.load(is);
} catch (final IOException e) {
throw new UncheckedIOException("Could not load '" + PROPERTIES_FILENAME + "'.", e);
}
return properties;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy