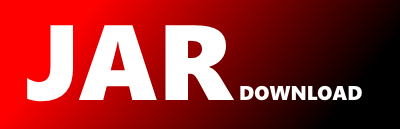
com.goeuro.sync4j.fs.FileSystem Maven / Gradle / Ivy
package com.goeuro.sync4j.fs;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import java.io.IOException;
import java.io.InputStream;
import java.math.BigInteger;
import java.net.URI;
import java.util.Optional;
import java.util.function.Predicate;
import java.util.stream.Stream;
public interface FileSystem {
WriteListener DEFAULT_WRITE_LISTENER = (actual, transferred) -> {
};
Predicate> DEFAULT_DELETE_PREDICATE = candidate -> true;
boolean couldHandle(@Nonnull LoosePath plainLoosePath);
boolean couldHandle(@Nonnull URI uri);
@Nonnull
Path resolve(@Nonnull URI uri);
@Nonnull
Path resolve(@Nonnull String path);
@Nonnull
Path resolve(@Nonnull T parent, @Nonnull String path);
@Nonnull
String relativize(@Nonnull T base, @Nonnull T other);
@Nonnull
Optional> normalize(@Nonnull Path input);
@Nonnull
Stream> list(@Nonnull T path);
@Nonnull
Stream> listAll(@Nonnull T path);
@Nonnull
Optional> detailsOf(@Nonnull T plain);
@Nonnull
Optional open(@Nonnull T path) throws IOException;
boolean isTemporary(@Nonnull Exception e, @Nonnull T source);
@Nonnull
default File write(@Nonnull T path, @Nonnull InputStream is) throws IOException {
return write(path, is, DEFAULT_WRITE_LISTENER);
}
@Nonnull
File write(@Nonnull T path, @Nonnull InputStream is, @Nonnull WriteListener extends LoosePath> listener) throws IOException;
@Nonnull
Directory createDirectory(@Nonnull T path) throws IOException;
default void delete(@Nonnull T path) {
//noinspection unchecked,RedundantCast,rawtypes
delete(path, (Predicate extends Path>) (Predicate) DEFAULT_DELETE_PREDICATE);
}
void delete(@Nonnull T path, @Nonnull Predicate extends Path> shouldBeDeleted);
@FunctionalInterface
interface WriteListener {
void onTransferProgress(@Nonnull T path, @Nonnegative @Nonnull BigInteger transferred);
default void onTransferDone(@Nonnull T path, @Nonnegative @Nonnull BigInteger total) {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy