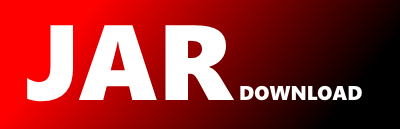
com.goeuro.sync4j.utils.Digests Maven / Gradle / Ivy
package com.goeuro.sync4j.utils;
import javax.annotation.Nonnull;
import java.io.IOException;
import java.io.InputStream;
import java.io.UncheckedIOException;
import java.nio.file.Path;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import static java.nio.file.Files.newInputStream;
import static java.util.Base64.getEncoder;
public final class Digests {
@Nonnull
public static String md5HashAsStringOf(@Nonnull Path source) {
return md5HashAsStringOf(() -> newInputStream(source));
}
@Nonnull
public static String md5HashAsStringOf(@Nonnull InputStreamOpener source) {
final byte[] hash = md5HashOf(source);
return getEncoder().encodeToString(hash);
}
@Nonnull
public static byte[] md5HashOf(@Nonnull InputStreamOpener source) {
try (final InputStream is = source.open()) {
return md5HashOf(is);
} catch (final IOException e) {
throw new UncheckedIOException(e);
}
}
@Nonnull
public static byte[] md5HashOf(@Nonnull InputStream is) throws IOException {
final MessageDigest digest = newMd5Digest();
final byte[] buf = new byte[8172];
int read = is.read(buf);
while (read >= 0) {
digest.update(buf, 0, read);
read = is.read(buf);
}
return digest.digest();
}
@Nonnull
public static MessageDigest newMd5Digest() {
try {
return MessageDigest.getInstance("MD5");
} catch (final NoSuchAlgorithmException e) {
throw new RuntimeException("There is no MD5 digester available.", e);
}
}
@FunctionalInterface
public interface InputStreamOpener {
@Nonnull
InputStream open() throws IOException;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy