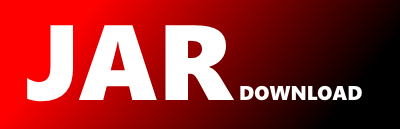
com.gs.api.accelrx.context.log4j.AccelRxMDCAdapter Maven / Gradle / Ivy
The newest version!
package com.gs.api.accelrx.context.log4j;
import io.reactiverse.contextual.logging.impl.ContextualDataImpl;
import io.vertx.core.Context;
import io.vertx.core.Vertx;
import io.vertx.core.impl.ContextInternal;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.slf4j.MDC;
import org.slf4j.helpers.NOPMDCAdapter;
import org.slf4j.helpers.Util;
import org.slf4j.spi.MDCAdapter;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
public class AccelRxMDCAdapter implements MDCAdapter {
private static final Logger log = LoggerFactory.getLogger(AccelRxMDCAdapter.class);
private static final MDCAdapter DELEGATE_MDC_ADAPTER;
static {
Optional> maybeMdcAdapterClazz = maybeGetClazz("org.apache.logging.slf4j.Log4jMDCAdapter")
.or(() -> maybeGetClazz("ch.qos.logback.classic.util.LogbackMDCAdapter"));
DELEGATE_MDC_ADAPTER = maybeMdcAdapterClazz
.map(AccelRxMDCAdapter::newInstance)
.filter(MDCAdapter.class::isInstance)
.map(MDCAdapter.class::cast)
.orElseGet(() -> {
Util.report("Failed to load log4j or logback \"org.slf4j.spi.MDCAdapter\" implementations.");
Util.report("Defaulting to no-operation MDCAdapter implementation.");
return new NOPMDCAdapter();
});
}
private static Object newInstance(Class> aClass) {
try {
return aClass.getConstructor().newInstance();
} catch (InstantiationException | IllegalAccessException | InvocationTargetException | NoSuchMethodException e) {
return null;
}
}
private static Optional> maybeGetClazz(String className) {
try {
return Optional.of(Class.forName(className));
} catch (ClassNotFoundException e) {
return Optional.empty();
}
}
public static void install() {
synchronized (AccelRxMDCAdapter.class) {
try {
final Field mdcAdapter = MDC.class.getDeclaredField("mdcAdapter");
mdcAdapter.setAccessible(true);
mdcAdapter.set(null, new AccelRxMDCAdapter());
} catch (NoSuchFieldException | IllegalAccessException e) {
throw new RuntimeException(e);
}
}
}
@Override
public void put(String key, String val) {
if (Context.isOnVertxThread()) {
if (val == null) {
remove(key);
} else {
getContextualDataMap().put(key, val);
}
} else {
log.trace("Putting context value={} with key={} using delegate", val, key);
DELEGATE_MDC_ADAPTER.put(key, val);
}
}
@Override
public String get(String key) {
if (Context.isOnVertxThread()) {
return getContextualDataMap().get(key);
} else {
log.trace("Getting context key={} using delegate", key);
return DELEGATE_MDC_ADAPTER.get(key);
}
}
@Override
public void remove(String key) {
if (Context.isOnVertxThread()) {
getContextualDataMap().remove(key);
} else {
log.trace("Removing context key={} using delegate", key);
DELEGATE_MDC_ADAPTER.remove(key);
}
}
@Override
public void clear() {
if (Context.isOnVertxThread()) {
getContextualDataMap().clear();
} else {
log.trace("Clearing context map on delegate");
DELEGATE_MDC_ADAPTER.clear();
}
}
@Override
public Map getCopyOfContextMap() {
if (Context.isOnVertxThread()) {
return new HashMap<>(getContextualDataMap());
} else {
log.trace("Getting context map from delegate");
return DELEGATE_MDC_ADAPTER.getCopyOfContextMap();
}
}
@Override
public void setContextMap(Map contextMap) {
if (Context.isOnVertxThread()) {
getContextualDataMap().clear();
getContextualDataMap().putAll(contextMap);
} else {
log.trace("Setting context map on delegate");
DELEGATE_MDC_ADAPTER.setContextMap(contextMap);
}
}
private static Map getContextualDataMap() {
ContextInternal ctx = (ContextInternal) Vertx.currentContext();
if (ctx == null) {
log.warn("Expected Vertx context to be present but was null");
return Collections.emptyMap();
} else {
return ContextualDataImpl.contextualDataMap(ctx);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy