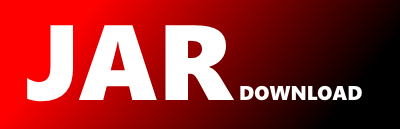
com.gs.api.accelrx.context.rxjava3.ContextPropagatorOnFlowableSubscribeAction Maven / Gradle / Ivy
package com.gs.api.accelrx.context.rxjava3;
import io.reactivex.rxjava3.core.Flowable;
import io.reactivex.rxjava3.core.FlowableSubscriber;
import io.reactivex.rxjava3.functions.BiFunction;
import org.reactivestreams.Subscriber;
import org.reactivestreams.Subscription;
import java.util.concurrent.Executor;
@SuppressWarnings("rawtypes")
public class ContextPropagatorOnFlowableSubscribeAction implements BiFunction {
private final ThreadContext threadContext;
public ContextPropagatorOnFlowableSubscribeAction(ThreadContext threadContext) {
this.threadContext = threadContext;
}
@Override
@SuppressWarnings({"rawtypes", "unchecked"})
public Subscriber apply(Flowable flowable, Subscriber subscriber) throws Throwable {
return new ContextCapturerFlowable<>(subscriber, threadContext.currentContextExecutor());
}
private static final class ContextCapturerFlowable implements FlowableSubscriber {
private final Subscriber downstream;
private final Executor contextExecutor;
public ContextCapturerFlowable(Subscriber observer, Executor contextExecutor) {
this.downstream = observer;
this.contextExecutor = contextExecutor;
}
@Override
public void onSubscribe(Subscription d) {
contextExecutor.execute(() -> downstream.onSubscribe(d));
}
@Override
public void onNext(T t) {
contextExecutor.execute(() -> downstream.onNext(t));
}
@Override
public void onError(Throwable e) {
contextExecutor.execute(() -> downstream.onError(e));
}
@Override
public void onComplete() {
contextExecutor.execute(downstream::onComplete);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy