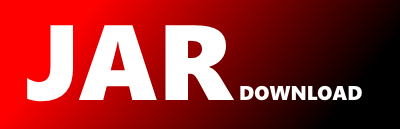
com.gs.api.accelrx.crypto.aws.AwsBlockingAesMasterKeyProvider Maven / Gradle / Ivy
The newest version!
package com.gs.api.accelrx.crypto.aws;
import com.amazonaws.encryptionsdk.*;
import com.amazonaws.encryptionsdk.exception.AwsCryptoException;
import com.amazonaws.encryptionsdk.exception.NoSuchMasterKeyException;
import com.amazonaws.encryptionsdk.exception.UnsupportedProviderException;
import com.amazonaws.encryptionsdk.jce.JceMasterKey;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.util.*;
/**
* An implementation of MasterKeyProvider for use with the aws-crypto-sdk. The said SDK has a synchronous API,
* and so should be used with caution (typically using vertx.rxExecuteBlocking() or equivalent).
*/
public class AwsBlockingAesMasterKeyProvider extends MasterKeyProvider {
/*
CAUTION: Changing provider can have an impact on consumers who need to decrypt messages.
*/
private static final String PROVIDER_NAME = BouncyCastleProvider.PROVIDER_NAME;
private static final String ACTIVE_ALIAS = "ACTIVE";
private static final String KEY_ALGO = "AES";
private static final String AWS_CRYPTO_WRAPPING_ALGORITHM = "AES/GCM/NoPadding";
private final AwsCryptoKeyRing keyRing;
public AwsBlockingAesMasterKeyProvider(AwsCryptoKeyRing keyRing) {
this.keyRing = keyRing;
}
@Override
public String getDefaultProviderId() {
return PROVIDER_NAME;
}
@Override
public JceMasterKey getMasterKey(String provider, String keyId) throws UnsupportedProviderException, NoSuchMasterKeyException {
return keyRing.getKey(keyId)
.map(key -> JceMasterKey.getInstance(
new SecretKeySpec(key.getSecretMaterial(), KEY_ALGO),
provider,
key.getId(),
AWS_CRYPTO_WRAPPING_ALGORITHM
))
.blockingGet();
}
@Override
public List getMasterKeysForEncryption(MasterKeyRequest masterKeyRequest) {
return Collections.singletonList(getMasterKey(ACTIVE_ALIAS));
}
@Override
public DataKey decryptDataKey(CryptoAlgorithm algorithm,
Collection extends EncryptedDataKey> encryptedDataKeys,
Map encryptionContext) throws AwsCryptoException {
final List exceptions = new ArrayList<>();
for (final EncryptedDataKey edk : encryptedDataKeys) {
try {
final String keyId = new String(edk.getProviderInformation(), StandardCharsets.UTF_8)
.split("\0")[0];
Exception keyIdException = new Exception("Decryption key ID: " + keyId);
exceptions.add(keyIdException);
final DataKey result = getMasterKey(keyId)
.decryptDataKey(algorithm, Collections.singletonList(edk), encryptionContext);
if (result != null) {
return result;
}
} catch (final Throwable ex) {
exceptions.add(ex);
}
}
throw buildCannotDecryptDksException(exceptions);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy