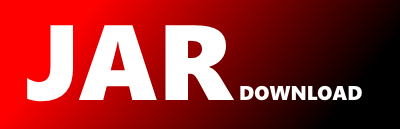
com.gs.api.accelrx.crypto.bouncycastle.BlockingBouncyCastleAesGcmCryptoProvider Maven / Gradle / Ivy
The newest version!
package com.gs.api.accelrx.crypto.bouncycastle;
import com.gs.api.accelrx.BlockingCryptoProvider;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class BlockingBouncyCastleAesGcmCryptoProvider implements BlockingCryptoProvider {
private static final int KEY_LENGTH = 32;
private static final int IV_LENGTH = 16;
private final byte[][] masterKeys;
private BlockingBouncyCastleAesGcmCryptoProvider(byte[][] masterKeys) {
this.masterKeys = masterKeys;
}
public static BlockingBouncyCastleAesGcmCryptoProvider create(byte[] key) {
return new BlockingBouncyCastleAesGcmCryptoProvider(masterKeys(key));
}
private static byte[][] masterKeys(byte[] masterKey) {
if (masterKey.length < KEY_LENGTH) {
throw new IllegalArgumentException("Invalid master key file");
}
if (masterKey.length % KEY_LENGTH != 0) {
throw new IllegalArgumentException("byte length in file is not divisible by key length.");
}
byte[][] masterKeys = new byte[masterKey.length / KEY_LENGTH][KEY_LENGTH];
for (int i = 0; i < masterKeys.length; i++) {
System.arraycopy(masterKey, i * KEY_LENGTH, masterKeys[i], 0, KEY_LENGTH);
}
return masterKeys;
}
@Override
public String encrypt(String message) throws IOException {
byte[] encryptedBytes = encrypt(message.getBytes(StandardCharsets.UTF_8));
return Base64.getEncoder().encodeToString(encryptedBytes);
}
@Override
public byte[] encrypt(byte[] message) throws IOException {
BouncyCastleGCMEncryption encryption = BouncyCastleGCMEncryption.create(masterKeys, KEY_LENGTH, IV_LENGTH);
return encryption.encrypt(message);
}
@Override
public String decrypt(String cipherBytes) throws IOException {
byte[] decryptedBytes = decrypt(Base64.getDecoder().decode(cipherBytes));
return new String(decryptedBytes, StandardCharsets.UTF_8);
}
@Override
public byte[] decrypt(byte[] cipherBytes) throws IOException {
BouncyCastleGCMEncryption encryption = BouncyCastleGCMEncryption.create(masterKeys, KEY_LENGTH, IV_LENGTH);
return encryption.decrypt(cipherBytes);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy