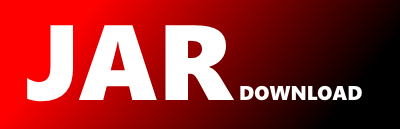
com.gs.api.accelrx.crypto.bouncycastle.BlockingBouncyCastleAesGcmObfuscationProvider Maven / Gradle / Ivy
package com.gs.api.accelrx.crypto.bouncycastle;
import com.gs.api.accelrx.BlockingObfuscationProvider;
import io.reactivex.rxjava3.core.Single;
import io.vertx.rxjava3.core.Vertx;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class BlockingBouncyCastleAesGcmObfuscationProvider implements BlockingObfuscationProvider {
private static final Logger log = LoggerFactory.getLogger(BlockingBouncyCastleAesGcmObfuscationProvider.class);
private static final int KEY_LENGTH = 32;
private static final int IV_LENGTH = 16;
private final byte[][] masterKeys;
private final byte[][] salts;
private BlockingBouncyCastleAesGcmObfuscationProvider(byte[][] masterKeys, byte[][] salts) {
this.masterKeys = masterKeys;
this.salts = salts;
}
static BlockingBouncyCastleAesGcmObfuscationProvider create(byte[] key, byte[] salt) {
return new BlockingBouncyCastleAesGcmObfuscationProvider(getKeys(key), getKeys(salt));
}
public static Single create(Vertx vertx, String keyFilename, String saltFilename) {
log.info("Creating obfuscator using keyFile={} and saltFile={}", keyFilename, saltFilename);
return Single.zip(readKey(vertx, keyFilename), readKey(vertx, saltFilename), BlockingBouncyCastleAesGcmObfuscationProvider::create);
}
private static Single readKey(Vertx vertx, String filename) {
return vertx.fileSystem()
.rxReadFile(filename)
.map(buffer -> buffer.getDelegate().getBytes());
}
@Override
public String obfuscate(String message) throws IOException {
byte[] encryptedBytes = obfuscate(message.getBytes(StandardCharsets.UTF_8));
return Base64.getEncoder().encodeToString(encryptedBytes);
}
@Override
public byte[] obfuscate(byte[] message) throws IOException {
BouncyCastleGCMObfuscation obfuscation = BouncyCastleGCMObfuscation.create(masterKeys, salts, KEY_LENGTH, IV_LENGTH);
return obfuscation.obfuscate(message);
}
private static byte[][] getKeys(byte[] key) {
if (key.length < KEY_LENGTH) {
throw new IllegalArgumentException("Invalid key file");
}
if (key.length % KEY_LENGTH != 0) {
throw new IllegalArgumentException("byte length in file is not divisible by key length.");
}
byte[][] keys = new byte[key.length / KEY_LENGTH][KEY_LENGTH];
for (int i = 0; i < keys.length; i++) {
System.arraycopy(key, i * KEY_LENGTH, keys[i], 0, KEY_LENGTH);
}
return keys;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy