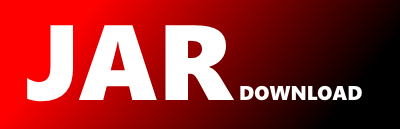
com.gs.api.accelrx.encoding.AccelRxEncodingConfig Maven / Gradle / Ivy
package com.gs.api.accelrx.encoding;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.gs.api.accelrx.config.ConfigEx;
import com.typesafe.config.Config;
import io.reactivex.rxjava3.core.Single;
public class AccelRxEncodingConfig {
@JsonProperty
private int jsonSerializerExecutorPoolSize;
@JsonProperty
private int jsonDeserializerExecutorPoolSize;
private AccelRxEncodingConfig(int jsonSerializerExecutorPoolSize,
int jsonDeserializerExecutorPoolSize) {
this.jsonSerializerExecutorPoolSize = jsonSerializerExecutorPoolSize;
this.jsonDeserializerExecutorPoolSize = jsonDeserializerExecutorPoolSize;
}
public int jsonSerializerExecutorPoolSize() {
return jsonSerializerExecutorPoolSize;
}
public int jsonDeserializerExecutorPoolSize() {
return jsonDeserializerExecutorPoolSize;
}
public static Single from(Config config) {
ConfigEx configEx = ConfigEx.adapt(config);
return Single.just(builder())
.doOnSuccess(builder -> configEx.maybeInt("jsonSerializerPoolSize").ifPresent(builder::withJsonSerializerExecutorPoolSize))
.doOnSuccess(builder -> configEx.maybeInt("jsonDeserializerPoolSize").ifPresent(builder::withJsonDeserializerExecutorPoolSize))
.map(Builder::build);
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private int jsonSerializerExecutorPoolSize = -1;
private int jsonDeserializerExecutorPoolSize = -1;
private Builder() {
}
public Builder withJsonSerializerExecutorPoolSize(int jsonSerializerExecutorPoolSize) {
this.jsonSerializerExecutorPoolSize = jsonSerializerExecutorPoolSize;
return this;
}
public Builder withJsonDeserializerExecutorPoolSize(int jsonDeserializerExecutorPoolSize) {
this.jsonDeserializerExecutorPoolSize = jsonDeserializerExecutorPoolSize;
return this;
}
public AccelRxEncodingConfig build() {
return new AccelRxEncodingConfig(jsonSerializerExecutorPoolSize, jsonDeserializerExecutorPoolSize);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy