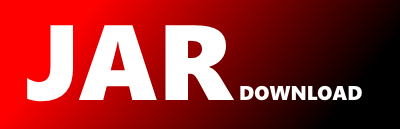
com.gs.api.accelrx.monitor.remote.RemoteMetricsSourceConfig Maven / Gradle / Ivy
The newest version!
package com.gs.api.accelrx.monitor.remote;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.gs.api.accelrx.config.ConfigEx;
import com.typesafe.config.Config;
import io.reactivex.rxjava3.core.Single;
import java.time.Duration;
public class RemoteMetricsSourceConfig {
@JsonProperty
private final String prefix;
private final Duration initialDelay;
private final Duration delay;
private final String host;
private final String path;
private final int port;
private final boolean ssl;
private final boolean enabled;
private RemoteMetricsSourceConfig(String prefix, Duration initialDelay, Duration delay, String host, String path, int port, boolean ssl, boolean enabled) {
if (prefix == null || !prefix.matches("[a-zA-Z_:][a-zA-Z0-9_:]+")) {
throw new IllegalArgumentException("prefix must be non-null and match \"[a-zA-Z_:][a-zA-Z0-9_:]+\", prefix=" + prefix);
}
this.prefix = prefix.endsWith("_") || prefix.endsWith(":") ? prefix : prefix + "_";
this.initialDelay = initialDelay;
this.delay = delay;
this.host = host;
this.path = path;
this.port = port;
this.ssl = ssl;
this.enabled = enabled;
}
public String prefix() {
return prefix;
}
public String host() {
return host;
}
public String path() {
return path;
}
public int port() {
return port;
}
public boolean ssl() {
return ssl;
}
public boolean enabled() {
return enabled;
}
public Duration initialDelay() {
return initialDelay;
}
public Duration delay() {
return delay;
}
public static Single from(Config config) {
ConfigEx configEx = ConfigEx.adapt(config);
return Single.just(builder())
.doOnSuccess(builder -> configEx.maybeString("prefix").ifPresent(builder::withPrefix))
.doOnSuccess(builder -> configEx.maybeDuration("initialDelay").ifPresent(builder::withInitialDelay))
.doOnSuccess(builder -> configEx.maybeDuration("delay").ifPresent(builder::withDelay))
.doOnSuccess(builder -> configEx.maybeString("host").ifPresent(builder::withHost))
.doOnSuccess(builder -> configEx.maybeString("path").ifPresent(builder::withPath))
.doOnSuccess(builder -> configEx.maybeInt("port").ifPresent(builder::withPort))
.doOnSuccess(builder -> configEx.maybeBoolean("ssl").ifPresent(builder::withSsl))
.doOnSuccess(builder -> configEx.maybeBoolean("enabled").ifPresent(builder::withEnabled))
.map(Builder::build);
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private String prefix;
private Duration initialDelay = Duration.ofSeconds(5);
private Duration delay = Duration.ofSeconds(15);
private String host;
private String path = "/";
private int port = 443;
private boolean ssl = true;
private boolean enabled = true;
private Builder() {
}
public Builder from(RemoteMetricsSourceConfig config) {
withInitialDelay(config.initialDelay());
withDelay(config.delay());
return this;
}
public Builder withPrefix(String prefix) {
this.prefix = prefix;
return this;
}
public Builder withHost(String host) {
this.host = host;
return this;
}
public Builder withPath(String path) {
this.path = path;
return this;
}
public Builder withPort(int port) {
this.port = port;
return this;
}
public Builder withSsl(boolean ssl) {
this.ssl = ssl;
return this;
}
public Builder withEnabled(boolean enabled) {
this.enabled = enabled;
return this;
}
public Builder withInitialDelay(Duration initialDelay) {
this.initialDelay = initialDelay;
return this;
}
public Builder withDelay(Duration delay) {
this.delay = delay;
return this;
}
public RemoteMetricsSourceConfig build() {
return new RemoteMetricsSourceConfig(prefix, initialDelay, delay, host, path, port, ssl, enabled);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy