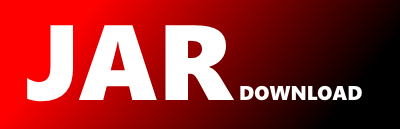
com.gs.api.accelrx.monitor.util.ManifestAttributes Maven / Gradle / Ivy
The newest version!
package com.gs.api.accelrx.monitor.util;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.net.JarURLConnection;
import java.net.URL;
import java.net.URLConnection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.jar.Attributes;
import java.util.jar.Manifest;
public class ManifestAttributes {
private static final Logger logger = LoggerFactory.getLogger(ManifestAttributes.class);
public static final String IMPLEMENTATION_VERSION = "Implementation-Version";
public static final String BUILD_JDK_SPEC = "Build-Jdk-Spec";
public static final String BUILD_DATE = "Build-Date";
public static final String BUILD_NUMBER = "Build-Number";
public static final String PIPELINE_URL = "Pipeline-Url";
public static final String COMMIT_REVISION = "Commit-Revision";
private static final String[] DEFAULT_ATTRIBUTES = {
IMPLEMENTATION_VERSION,
BUILD_JDK_SPEC,
BUILD_DATE,
BUILD_NUMBER,
PIPELINE_URL,
COMMIT_REVISION
};
private static final Map manifestAttributes = Collections.unmodifiableMap(classManifestAttributes());
public static Map get() {
return manifestAttributes;
}
private static Map classManifestAttributes() {
Map aboutProperties = new HashMap<>();
try {
URL resource = ManifestAttributes.class.getResource(ManifestAttributes.class.getSimpleName() + ".class");
URLConnection urlConnection = resource == null ? null : resource.openConnection();
if (urlConnection instanceof JarURLConnection) {
JarURLConnection jarURLConnection = (JarURLConnection) urlConnection;
Manifest manifest = jarURLConnection.getManifest();
Attributes mainAttributes = manifest.getMainAttributes();
for (String attributeName : DEFAULT_ATTRIBUTES) {
copyAttribute(mainAttributes, aboutProperties, attributeName);
}
} else {
logger.warn("Not starting from JAR, so no manifest attributes");
}
} catch (IOException e) {
logger.warn("Failed to load manifest attributes!", e);
}
return aboutProperties;
}
private static void copyAttribute(Attributes from, Map to, String key) {
String value = from.getValue(key);
to.put(key, value != null ? value : "");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy