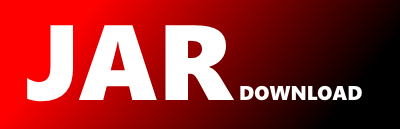
com.gs.api.accelrx.web.client.AccelRxWebClientConfig Maven / Gradle / Ivy
The newest version!
package com.gs.api.accelrx.web.client;
import com.gs.api.accelrx.config.ConfigEx;
import com.gs.api.accelrx.config.ConfigString;
import com.gs.api.accelrx.config.ProxyConfig;
import com.gs.api.accelrx.web.client.servicediscovery.ServiceLocation;
import com.typesafe.config.Config;
import io.reactivex.rxjava3.core.Single;
import io.vertx.core.json.JsonObject;
import static java.util.Objects.requireNonNull;
public class AccelRxWebClientConfig {
private String name;
private ServiceLocation serviceLocation;
private boolean validateResponse;
private boolean validateErrorResponse;
private int validationExecutorPoolSize;
private boolean disableLogging;
private boolean internal;
private boolean alwaysUseIncomingAuthToken;
private int maxPoolSize;
private int retries;
private int retryDelayInMs;
private int retryBackoffDelayInMs;
private int retryBackoffMaxDelayInMs;
private boolean keepAlive;
private int keepAliveTimeout;
private long requestTimeoutMs;
private boolean pipelining;
private int pipeliningLimit;
private int responseBodyLimit;
private boolean disableSignature;
private boolean followRedirects;
private long healthCheckScanPeriod;
private long healthCheckTimeout;
private boolean tcpFastOpen;
private boolean tcpCork;
private boolean tcpQuickAck;
private boolean reusePort;
private boolean mutualAuth;
private ConfigString clientCertPath;
private ConfigString clientCertPassword;
private ConfigString trustStorePath;
private ConfigString trustStorePassword;
private ProxyConfig proxy;
private int blockingTimeout;
protected AccelRxWebClientConfig() {
}
protected AccelRxWebClientConfig(String name,
ServiceLocation serviceLocation,
boolean validateResponse,
boolean validateErrorResponse,
int validationExecutorPoolSize,
boolean disableLogging,
boolean internal,
boolean alwaysUseIncomingAuthToken,
int maxPoolSize,
int retries,
int retryDelayInMs,
int retryBackoffDelayInMs,
int retryBackoffMaxDelayInMs,
boolean keepAlive,
int keepAliveTimeout,
long requestTimeoutMs,
boolean pipelining,
int pipeliningLimit,
int responseBodyLimit,
boolean disableSignature,
long healthCheckScanPeriod,
long healthCheckTimeout,
boolean tcpFastOpen,
boolean tcpCork,
boolean tcpQuickAck,
boolean reusePort,
boolean mutualAuth,
ConfigString clientCertPath,
ConfigString clientCertPassword,
ConfigString trustStorePath,
ConfigString trustStorePassword,
ProxyConfig proxy,
boolean followRedirects,
int blockingTimeout) {
this.name = name;
this.serviceLocation = serviceLocation;
this.validateResponse = validateResponse;
this.validateErrorResponse = validateErrorResponse;
this.validationExecutorPoolSize = validationExecutorPoolSize;
this.disableLogging = disableLogging;
this.internal = internal;
this.alwaysUseIncomingAuthToken = alwaysUseIncomingAuthToken;
this.maxPoolSize = maxPoolSize;
this.retries = retries;
this.retryDelayInMs = retryDelayInMs;
this.retryBackoffDelayInMs = retryBackoffDelayInMs;
this.retryBackoffMaxDelayInMs = retryBackoffMaxDelayInMs;
this.keepAlive = keepAlive;
this.keepAliveTimeout = keepAliveTimeout;
this.requestTimeoutMs = requestTimeoutMs;
this.pipelining = pipelining;
this.pipeliningLimit = pipeliningLimit;
this.responseBodyLimit = responseBodyLimit;
this.disableSignature = disableSignature;
this.healthCheckScanPeriod = healthCheckScanPeriod;
this.healthCheckTimeout = healthCheckTimeout;
this.tcpFastOpen = tcpFastOpen;
this.tcpCork = tcpCork;
this.tcpQuickAck = tcpQuickAck;
this.reusePort = reusePort;
this.mutualAuth = mutualAuth;
this.clientCertPath = clientCertPath;
this.clientCertPassword = clientCertPassword;
this.trustStorePath = trustStorePath;
this.trustStorePassword = trustStorePassword;
this.proxy = proxy;
this.followRedirects = followRedirects;
this.blockingTimeout = blockingTimeout;
}
public String name() {
return name;
}
public ServiceLocation serviceLocation() {
return serviceLocation;
}
public JsonObject metadata() {
return new JsonObject();
}
public boolean validateResponse() {
return validateResponse;
}
public boolean validateErrorResponse() {
return validateErrorResponse;
}
public int validationExecutorPoolSize() {
return validationExecutorPoolSize;
}
public boolean disableLogging() {
return disableLogging;
}
public boolean isProxy() {
return proxy != null;
}
public ProxyConfig proxy() {
return proxy;
}
public boolean isMutualAuth() {
return mutualAuth;
}
public String clientCertPath() {
return clientCertPath != null ? clientCertPath.value() : null;
}
public String clientCertPassword() {
return clientCertPassword != null ? clientCertPassword.value() : null;
}
public String trustStorePath() {
return trustStorePath != null ? trustStorePath.value() : null;
}
public String trustStorePassword() {
return trustStorePassword != null ? trustStorePassword.value() : null;
}
public boolean isInternal() {
return internal;
}
public boolean alwaysUseIncomingAuthToken() {
return alwaysUseIncomingAuthToken;
}
public Integer maxPoolSize() {
return maxPoolSize;
}
public Integer retries() {
return retries;
}
public Integer retryDelayInMs() {
return retryDelayInMs;
}
public Integer retryBackoffDelayInMs() {
return retryBackoffDelayInMs;
}
public Integer retryBackoffMaxDelayInMs() {
return retryBackoffMaxDelayInMs;
}
public Boolean keepAlive() {
return keepAlive;
}
public Integer keepAliveTimeout() {
return keepAliveTimeout;
}
public Long requestTimeoutMs() {
return this.requestTimeoutMs;
}
public Boolean pipelining() {
return pipelining;
}
public Integer pipeliningLimit() {
return pipeliningLimit;
}
public int responseBodyLimit() {
return responseBodyLimit;
}
public boolean disableSignature() {
return disableSignature;
}
public long healthCheckScanPeriod() {
return healthCheckScanPeriod;
}
public long healthCheckTimeout() {
return healthCheckTimeout;
}
public boolean tcpFastOpen() {
return tcpFastOpen;
}
public boolean tcpCork() {
return tcpCork;
}
public boolean tcpQuickAck() {
return tcpQuickAck;
}
public boolean reusePort() {
return reusePort;
}
public boolean followRedirects() {
return followRedirects;
}
public int blockingTimeout() {
return blockingTimeout;
}
public static Single from(String name, Config config) {
ConfigEx configEx = ConfigEx.adapt(config);
return Single.just(builder().withName(name))
.doOnSuccess(builder -> configEx.maybeString("clientCertPath").ifPresent(builder::withClientCertPath))
.doOnSuccess(builder -> configEx.maybeString("clientCertPassword").ifPresent(builder::withClientCertPassword))
.doOnSuccess(builder -> configEx.maybeBoolean("alwaysUseIncomingAuthToken").ifPresent(builder::withAlwaysUseIncomingAuthToken))
.doOnSuccess(builder -> configEx.maybeBoolean("disableSignature").ifPresent(builder::withDisableSignature))
.doOnSuccess(builder -> configEx.maybeBoolean("mutualAuth").ifPresent(builder::withMutualAuth))
.doOnSuccess(builder -> configEx.maybeString("trustStorePath").ifPresent(builder::withTrustStorePath))
.doOnSuccess(builder -> configEx.maybeString("trustStorePassword").ifPresent(builder::withTrustStorePassword))
.doOnSuccess(builder -> configEx.maybeLong("healthCheckScanPeriod").ifPresent(builder::withHealthCheckScanPeriod))
.doOnSuccess(builder -> configEx.maybeLong("healthCheckTimeout").ifPresent(builder::withHealthCheckTimeout))
.doOnSuccess(builder -> configEx.maybeBoolean("validateResponse").ifPresent(builder::withValidateResponse))
.doOnSuccess(builder -> configEx.maybeBoolean("validateErrorResponse").ifPresent(builder::withValidateErrorResponse))
.doOnSuccess(builder -> configEx.maybeInt("validationExecutorPoolSize").ifPresent(builder::withValidationExecutorPoolSize))
.doOnSuccess(builder -> configEx.maybeBoolean("disableLogging").ifPresent(builder::withDisableLogging))
.doOnSuccess(builder -> configEx.maybeBoolean("internal").ifPresent(builder::withInternal))
.doOnSuccess(builder -> configEx.maybeBoolean("pipelining").ifPresent(builder::withPipelining))
.doOnSuccess(builder -> configEx.maybeBoolean("keepAlive").ifPresent(builder::withKeepAlive))
.doOnSuccess(builder -> configEx.maybeBoolean("followRedirects").ifPresent(builder::withFollowRedirects))
// todo: switch to using ConfigEx#maybeDuration
.doOnSuccess(builder -> configEx.maybeInt("keepAliveTimeout").ifPresent(builder::withKeepAliveTimeout))
.doOnSuccess(builder -> configEx.maybeLong("requestTimeoutMs").ifPresent(builder::withRequestTimeoutMs))
.doOnSuccess(builder -> configEx.maybeInt("maxPoolSize").ifPresent(builder::withMaxPoolSize))
// todo: create a RetryPolicy config
.doOnSuccess(builder -> configEx.maybeInt("retries").ifPresent(builder::withRetries))
.doOnSuccess(builder -> configEx.maybeInt("retryDelay").ifPresent(builder::withRetryDelay))
.doOnSuccess(builder -> configEx.maybeInt("retryBackoffDelay").ifPresent(builder::withRetryBackoffDelay))
.doOnSuccess(builder -> configEx.maybeInt("retryBackoffMaxDelay").ifPresent(builder::withRetryBackoffMaxDelay))
.doOnSuccess(builder -> configEx.maybeInt("pipeliningLimit").ifPresent(builder::withPipeliningLimit))
.doOnSuccess(builder -> configEx.maybeInt("responseBodyLimit").ifPresent(builder::withResponseBodyLimit))
.doOnSuccess(builder -> configEx.maybeBoolean("tcpFastOpen").ifPresent(builder::withTcpFastOpen))
.doOnSuccess(builder -> configEx.maybeBoolean("tcpCork").ifPresent(builder::withTcpCork))
.doOnSuccess(builder -> configEx.maybeBoolean("tcpQuickAck").ifPresent(builder::withTcpQuickAck))
.doOnSuccess(builder -> configEx.maybeBoolean("reusePort").ifPresent(builder::withReusePort))
.doOnSuccess(builder -> configEx.maybeInt("blockingTimeout").ifPresent(builder::withBlockingTimeout))
.flatMap(builder -> {
return configEx.rxMaybeMappedConfig("proxy", ProxyConfig::from)
.map(builder::withProxy)
.defaultIfEmpty(builder);
})
.map(Builder::build);
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private String name;
private ServiceLocation serviceLocation;
private boolean validateResponse = true;
private boolean validateErrorResponse = true;
private int validationExecutorPoolSize = 10;
private boolean disableLogging = false;
private boolean internal = true;
private boolean alwaysUseIncomingAuthToken = false;
private int maxPoolSize = 50;
private boolean keepAlive = true;
private int keepAliveTimeout = 5;
private long requestTimeoutMs = 30 * 1000;
private boolean pipelining = false;
private int pipeliningLimit = 10;
private int responseBodyLimit = 5 * 1024 * 1024;
private boolean disableSignature = false;
private boolean followRedirects = false;
private long healthCheckScanPeriod = 2000;
private long healthCheckTimeout = 1000;
private boolean mutualAuth = false;
private ConfigString clientCertPath;
private ConfigString clientCertPassword;
private ConfigString trustStorePath;
private ConfigString trustStorePassword;
private int retries = 0;
private int retryDelayInMs = -1;
private int retryBackoffDelayInMs = 50;
private int retryBackoffMaxDelayInMs = 2000;
public boolean tcpFastOpen = false;
public boolean tcpCork = false;
public boolean tcpQuickAck = false;
public boolean reusePort = false;
private ProxyConfig proxy;
private int blockingTimeout = 30 * 1000;
private Builder() {
}
public Builder withName(String name) {
this.name = name;
return this;
}
public Builder withServiceLocation(ServiceLocation serviceLocation) {
this.serviceLocation = serviceLocation;
return this;
}
public Builder withValidateResponse(Boolean validateResponse) {
this.validateResponse = validateResponse;
return this;
}
public Builder withValidateErrorResponse(boolean validateErrorResponse) {
this.validateErrorResponse = validateErrorResponse;
return this;
}
public Builder withValidationExecutorPoolSize(int validationExecutorPoolSize) {
this.validationExecutorPoolSize = validationExecutorPoolSize;
return this;
}
public Builder withDisableLogging(boolean disableLogging) {
this.disableLogging = disableLogging;
return this;
}
public Builder withFollowRedirects(boolean followRedirects) {
this.followRedirects = followRedirects;
return this;
}
public Builder withInternal(boolean internal) {
this.internal = internal;
return this;
}
public Builder withAlwaysUseIncomingAuthToken(boolean alwaysUseIncomingAuthToken) {
this.alwaysUseIncomingAuthToken = alwaysUseIncomingAuthToken;
return this;
}
public Builder withMaxPoolSize(int maxPoolSize) {
this.maxPoolSize = maxPoolSize;
return this;
}
public Builder withRetries(int retries) {
this.retries = retries;
return this;
}
public Builder withRetryDelay(int retryDelayInMs) {
this.retryDelayInMs = retryDelayInMs;
return this;
}
public Builder withRetryBackoffDelay(int retryBackoffDelayInMs) {
this.retryBackoffDelayInMs = retryBackoffDelayInMs;
return this;
}
public Builder withRetryBackoffMaxDelay(int retryBackoffMaxDelayInMs) {
this.retryBackoffMaxDelayInMs = retryBackoffMaxDelayInMs;
return this;
}
public Builder withKeepAlive(boolean keepAlive) {
this.keepAlive = keepAlive;
return this;
}
public Builder withKeepAliveTimeout(int keepAliveTimeout) {
this.keepAliveTimeout = keepAliveTimeout;
return this;
}
public Builder withRequestTimeoutMs(long requestTimeoutMs) {
this.requestTimeoutMs = requestTimeoutMs;
return this;
}
public Builder withDisableSignature(boolean disableSignature) {
this.disableSignature = disableSignature;
return this;
}
public Builder withPipelining(boolean pipelining) {
this.pipelining = pipelining;
return this;
}
public Builder withPipeliningLimit(int pipeliningLimit) {
this.pipeliningLimit = pipeliningLimit;
return this;
}
public Builder withResponseBodyLimit(int responseBodyLimit) {
this.responseBodyLimit = responseBodyLimit;
return this;
}
public Builder withHealthCheckScanPeriod(long healthCheckScanPeriod) {
this.healthCheckScanPeriod = healthCheckScanPeriod;
return this;
}
public Builder withHealthCheckTimeout(long healthCheckTimeout) {
this.healthCheckTimeout = healthCheckTimeout;
return this;
}
public Builder withTcpFastOpen(boolean tcpFastOpen) {
this.tcpFastOpen = tcpFastOpen;
return this;
}
public Builder withTcpCork(boolean tcpCork) {
this.tcpCork = tcpCork;
return this;
}
public Builder withTcpQuickAck(boolean tcpQuickAck) {
this.tcpQuickAck = tcpQuickAck;
return this;
}
public Builder withReusePort(boolean reusePort) {
this.reusePort = reusePort;
return this;
}
public Builder withMutualAuth(boolean mutualAuth) {
this.mutualAuth = mutualAuth;
return this;
}
public Builder withBlockingTimeout(int blockingTimeout) {
this.blockingTimeout = blockingTimeout;
return this;
}
public Builder withClientCertPath(String clientCertPath) {
this.clientCertPath = ConfigString.create(clientCertPath);
return this;
}
public Builder withClientCertPassword(String clientCertPassword) {
this.clientCertPassword = ConfigString.create(clientCertPassword);
return this;
}
public Builder withTrustStorePath(String trustStorePath) {
this.trustStorePath = ConfigString.create(trustStorePath);
return this;
}
public Builder withTrustStorePassword(String trustStorePassword) {
this.trustStorePassword = ConfigString.create(trustStorePassword);
return this;
}
public Builder withProxy(ProxyConfig proxy) {
this.proxy = proxy;
return this;
}
public AccelRxWebClientConfig build() {
return new AccelRxWebClientConfig(
name,
serviceLocation,
validateResponse,
validateErrorResponse,
validationExecutorPoolSize,
disableLogging,
internal,
alwaysUseIncomingAuthToken,
maxPoolSize,
retries,
retryDelayInMs,
retryBackoffDelayInMs,
retryBackoffMaxDelayInMs,
keepAlive,
keepAliveTimeout,
requestTimeoutMs,
pipelining,
pipeliningLimit,
responseBodyLimit,
disableSignature,
healthCheckScanPeriod,
healthCheckTimeout,
tcpFastOpen,
tcpCork,
tcpQuickAck,
reusePort,
mutualAuth,
clientCertPath,
clientCertPassword,
trustStorePath,
trustStorePassword,
proxy,
followRedirects,
blockingTimeout);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy