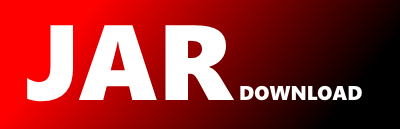
com.gs.dmn.feel.lib.FEELLib Maven / Gradle / Ivy
/*
* Copyright 2016 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License.
*
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*/
package com.gs.dmn.feel.lib;
import com.gs.dmn.feel.lib.type.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.List;
public interface FEELLib extends
NumericType, StringType, BooleanType, ListType, ContextType,
DateType, TimeType
© 2015 - 2025 Weber Informatics LLC | Privacy Policy