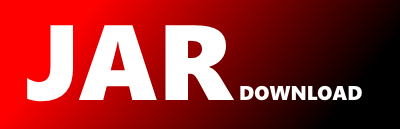
com.gs.dmn.signavio.transformation.GenerateMissingItemDefinitionsTransformer Maven / Gradle / Ivy
/*
* Copyright 2016 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License.
*
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*/
package com.gs.dmn.signavio.transformation;
import com.gs.dmn.DMNModelRepository;
import com.gs.dmn.ast.TItemDefinition;
import com.gs.dmn.log.BuildLogger;
import com.gs.dmn.log.Slf4jBuildLogger;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
public class GenerateMissingItemDefinitionsTransformer extends AbstractMissingItemDefinitionsTransformer {
private static final String ELEMENT_DEFINITIONS = "definitions";
private static final String ELEMENT_DEFINITION = "definition";
private static final String FIELD_NAME = "name";
private static final String FIELD_TYPE = "type";
private static final String FIELD_COLLECTION = "isCollection";
private List definitions;
public GenerateMissingItemDefinitionsTransformer() {
this(new Slf4jBuildLogger(LOGGER));
}
public GenerateMissingItemDefinitionsTransformer(BuildLogger logger) {
super(logger);
}
@Override
public void configure(Map configuration) {
this.definitions = parseConfigurationForDefinitions(configuration);
}
@Override
public DMNModelRepository transform(DMNModelRepository repository) {
if (isEmpty(repository)) {
logger.warn("Repository is empty; transformer will not run");
return repository;
}
if (this.definitions == null || this.definitions.isEmpty()) {
logger.warn("No definitions provided; transformer will not run");
return repository;
}
addNewDefinitions(repository, this.definitions);
this.transformRepository = false;
return repository;
}
private List parseConfigurationForDefinitions(Map configuration) {
List result = new ArrayList<>();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy