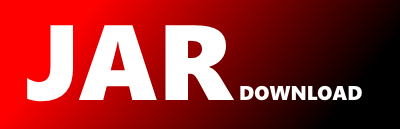
com.gs.obevo.impl.changepredicate.ChangeKeyPredicateBuilder Maven / Gradle / Ivy
/**
* Copyright 2017 Goldman Sachs.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.gs.obevo.impl.changepredicate;
import com.gs.obevo.api.appdata.Change;
import com.gs.obevo.util.VisibleForTesting;
import com.gs.obevo.util.lookuppredicate.LookupPredicateBuilder;
import org.eclipse.collections.api.block.function.Function;
import org.eclipse.collections.api.block.predicate.Predicate;
import org.eclipse.collections.api.collection.ImmutableCollection;
import org.eclipse.collections.api.list.ImmutableList;
import org.eclipse.collections.api.list.MutableList;
import org.eclipse.collections.impl.block.factory.Predicates;
import org.eclipse.collections.impl.factory.Lists;
import org.eclipse.collections.impl.list.fixed.ArrayAdapter;
import static com.gs.obevo.api.appdata.ObjectTypeAndNamePredicateBuilder.PREDICATE_SPLITTER;
import static com.gs.obevo.api.appdata.ObjectTypeAndNamePredicateBuilder.PART_SPLITTER;
import static com.gs.obevo.api.appdata.ObjectTypeAndNamePredicateBuilder.SINGLE_PREDICATE_SPLITTER;
/**
* Predicate to allow clients to only select specific Changes based on the identity fields, e.g. schema, change type,
* object name, and change name.
*
* Any combination of those fields can be applied here. See the unit test for examples.
*
* TODO more docs to come here.
*/
public class ChangeKeyPredicateBuilder {
public static ChangeKeyInclusionPredicateBuilder newBuilder() {
return new ChangeKeyInclusionPredicateBuilder();
}
public static Predicate super Change> parseFullPredicate(String fullPredicateString) {
ImmutableList fullPredicateParts = ArrayAdapter.adapt(fullPredicateString.split(PREDICATE_SPLITTER)).toImmutable();
ImmutableList> singlePredicates = fullPredicateParts.collect(new Function>() {
@Override
public Predicate super Change> valueOf(String singlePredicateString) {
return parseSinglePredicate(singlePredicateString);
}
});
return Predicates.or(singlePredicates);
}
@VisibleForTesting
static Predicate super Change> parseSinglePredicate(String singlePredicateString) {
MutableList changeParts = ArrayAdapter.adapt(singlePredicateString.split(SINGLE_PREDICATE_SPLITTER));
if (changeParts.size() > 4) {
throw new IllegalArgumentException("Cannot have more than 4 parts here (i.e. splits via the tilde ~)");
}
ImmutableList schemas = changeParts.size() > 0 ? parseSinglePredicatePart(changeParts.get(0)) : null;
ImmutableList changeTypes = changeParts.size() > 1 ? parseSinglePredicatePart(changeParts.get(1)) : null;
ImmutableList objectNames = changeParts.size() > 2 ? parseSinglePredicatePart(changeParts.get(2)) : null;
ImmutableList changeNames = changeParts.size() > 3 ? parseSinglePredicatePart(changeParts.get(3)) : null;
return newBuilder()
.setSchemas(schemas)
.setChangeTypes(changeTypes)
.setObjectNames(objectNames)
.setChangeNames(changeNames)
.build();
}
private static ImmutableList parseSinglePredicatePart(String predicateString) {
return ArrayAdapter.adapt(predicateString.split(PART_SPLITTER)).toImmutable();
}
public static class ChangeKeyInclusionPredicateBuilder {
private ImmutableCollection schemas;
private ImmutableCollection changeTypes;
private ImmutableCollection objectNames;
private ImmutableCollection changeNames;
private ChangeKeyInclusionPredicateBuilder() {
}
public ChangeKeyInclusionPredicateBuilder setSchemas(String... schemas) {
return this.setSchemas(Lists.immutable.with(schemas));
}
public ChangeKeyInclusionPredicateBuilder setSchemas(ImmutableCollection schemas) {
this.schemas = schemas;
return this;
}
public ChangeKeyInclusionPredicateBuilder setChangeTypes(String... changeTypes) {
return this.setChangeTypes(Lists.immutable.with(changeTypes));
}
public ChangeKeyInclusionPredicateBuilder setChangeTypes(ImmutableCollection changeTypes) {
this.changeTypes = changeTypes;
return this;
}
public ChangeKeyInclusionPredicateBuilder setObjectNames(String... objectNames) {
return this.setObjectNames(Lists.immutable.with(objectNames));
}
public ChangeKeyInclusionPredicateBuilder setObjectNames(ImmutableCollection objectNames) {
this.objectNames = objectNames;
return this;
}
public ChangeKeyInclusionPredicateBuilder setChangeNames(String... changeNames) {
return this.setChangeNames(Lists.immutable.with(changeNames));
}
public ChangeKeyInclusionPredicateBuilder setChangeNames(ImmutableCollection changeNames) {
this.changeNames = changeNames;
return this;
}
public Predicate super Change> build() {
return Predicates.attributePredicate(Change.TO_SCHEMA, LookupPredicateBuilder.convert(schemas))
.and(Predicates.attributePredicate(Change.TO_CHANGE_TYPE_NAME, LookupPredicateBuilder.convert(changeTypes)))
.and(Predicates.attributePredicate(Change.objectName(), LookupPredicateBuilder.convert(objectNames)))
.and(Predicates.attributePredicate(Change.TO_CHANGE_NAME, LookupPredicateBuilder.convert(changeNames)));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy