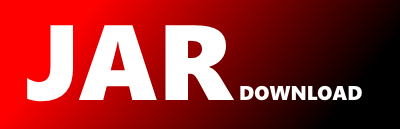
com.gs.obevo.db.sqlparser.syntaxparser.SqlParser Maven / Gradle / Ivy
/* Generated By:JJTree&JavaCC: Do not edit this line. SqlParser.java */
package com.gs.obevo.db.sqlparser.syntaxparser;
public class SqlParser/*@bgen(jjtree)*/implements SqlParserTreeConstants, SqlParserConstants {/*@bgen(jjtree)*/
protected JJTSqlParserState jjtree = new JJTSqlParserState();
/*****************************************
* THE SQL LANGUAGE GRAMMAR STARTS HERE *
*****************************************/
final public ASTCompilationUnit CompilationUnit() throws ParseException {
/*@bgen(jjtree) CompilationUnit */
ASTCompilationUnit jjtn000 = (ASTCompilationUnit)ASTCompilationUnit.jjtCreate(this, JJTCOMPILATIONUNIT);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case CREATE:
CreateStatement();
break;
case ALTER:
AlterStatement();
break;
case DROP:
DropStatement();
break;
default:
jj_la1[0] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
jj_consume_token(0);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
{if (true) return jjtn000;}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
throw new Error("Missing return statement in function");
}
final public void AlterStatement() throws ParseException {
/*@bgen(jjtree) AlterStatement */
ASTAlterStatement jjtn000 = (ASTAlterStatement)ASTAlterStatement.jjtCreate(this, JJTALTERSTATEMENT);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(ALTER);
jj_consume_token(TABLE);
TableName();
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case ADD:
AlterTableAdd();
break;
case DROP:
DropStatement();
break;
default:
jj_la1[1] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void AlterTableAdd() throws ParseException {
/*@bgen(jjtree) AlterTableAdd */
ASTAlterTableAdd jjtn000 = (ASTAlterTableAdd)ASTAlterTableAdd.jjtCreate(this, JJTALTERTABLEADD);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(ADD);
ConstraintClause();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void DropStatement() throws ParseException {
/*@bgen(jjtree) DropStatement */
ASTDropStatement jjtn000 = (ASTDropStatement)ASTDropStatement.jjtCreate(this, JJTDROPSTATEMENT);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(DROP);
DropObjectType();
DropObjectName();
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case TABLE:
case INDEX:
case SEQUENCE:
case CONSTRAINT:
case PRIMARY:
case KEY:
case UNIQUE:
case INTEGER_LITERAL:
case FLOATING_POINT_LITERAL:
case STRING_LITERAL:
case IDENTIFIER:
case LPAREN:
case MINUS:
case ASSIGN:
case 50:
PostObjectTableClauses();
break;
default:
jj_la1[2] = jj_gen;
;
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void CreateStatement() throws ParseException {
/*@bgen(jjtree) CreateStatement */
ASTCreateStatement jjtn000 = (ASTCreateStatement)ASTCreateStatement.jjtCreate(this, JJTCREATESTATEMENT);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(CREATE);
if (jj_2_1(3)) {
CreateTable();
} else {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case INDEX:
case UNIQUE:
case CLUSTERED:
case NONCLUSTERED:
case IDENTIFIER:
CreateIndex();
break;
default:
jj_la1[3] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void CreateTable() throws ParseException {
/*@bgen(jjtree) CreateTable */
ASTCreateTable jjtn000 = (ASTCreateTable)ASTCreateTable.jjtCreate(this, JJTCREATETABLE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(TABLE);
TableName();
jj_consume_token(LPAREN);
CreateTableColumnList();
label_1:
while (true) {
if (jj_2_2(2)) {
;
} else {
break label_1;
}
jj_consume_token(50);
CreateTableEnd();
}
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 50:
jj_consume_token(50);
break;
default:
jj_la1[4] = jj_gen;
;
}
jj_consume_token(RPAREN);
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case TABLE:
case INDEX:
case SEQUENCE:
case CONSTRAINT:
case PRIMARY:
case KEY:
case UNIQUE:
case INTEGER_LITERAL:
case FLOATING_POINT_LITERAL:
case STRING_LITERAL:
case IDENTIFIER:
case LPAREN:
case MINUS:
case ASSIGN:
case 50:
PostObjectTableClauses();
break;
default:
jj_la1[5] = jj_gen;
;
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void CreateTableEnd() throws ParseException {
/*@bgen(jjtree) CreateTableEnd */
ASTCreateTableEnd jjtn000 = (ASTCreateTableEnd)ASTCreateTableEnd.jjtCreate(this, JJTCREATETABLEEND);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case CONSTRAINT:
ConstraintClause();
break;
case PRIMARY:
PrimaryKeyClause();
break;
case UNIQUE:
UniqueClause();
break;
default:
jj_la1[6] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void ConstraintClause() throws ParseException {
/*@bgen(jjtree) ConstraintClause */
ASTConstraintClause jjtn000 = (ASTConstraintClause)ASTConstraintClause.jjtCreate(this, JJTCONSTRAINTCLAUSE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(CONSTRAINT);
ConstraintName();
if (jj_2_3(3)) {
PrimaryKeyClause();
} else if (jj_2_4(3)) {
UniqueClause();
} else {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case TABLE:
case INDEX:
case SEQUENCE:
case CONSTRAINT:
case PRIMARY:
case KEY:
case UNIQUE:
case INTEGER_LITERAL:
case FLOATING_POINT_LITERAL:
case STRING_LITERAL:
case IDENTIFIER:
case LPAREN:
case MINUS:
case ASSIGN:
case 50:
OtherConstraintClause();
break;
default:
jj_la1[7] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void PrimaryKeyClause() throws ParseException {
/*@bgen(jjtree) PrimaryKeyClause */
ASTPrimaryKeyClause jjtn000 = (ASTPrimaryKeyClause)ASTPrimaryKeyClause.jjtCreate(this, JJTPRIMARYKEYCLAUSE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(PRIMARY);
jj_consume_token(KEY);
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case CLUSTERED:
case NONCLUSTERED:
ClusterClause();
break;
default:
jj_la1[8] = jj_gen;
;
}
IndexColumnList();
if (jj_2_5(2)) {
PostConstraintClauses();
} else {
;
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void UniqueClause() throws ParseException {
/*@bgen(jjtree) UniqueClause */
ASTUniqueClause jjtn000 = (ASTUniqueClause)ASTUniqueClause.jjtCreate(this, JJTUNIQUECLAUSE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(UNIQUE);
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case CLUSTERED:
case NONCLUSTERED:
ClusterClause();
break;
default:
jj_la1[9] = jj_gen;
;
}
IndexColumnList();
if (jj_2_6(2)) {
PostConstraintClauses();
} else {
;
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void CreateIndex() throws ParseException {
/*@bgen(jjtree) CreateIndex */
ASTCreateIndex jjtn000 = (ASTCreateIndex)ASTCreateIndex.jjtCreate(this, JJTCREATEINDEX);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case UNIQUE:
Unique();
break;
default:
jj_la1[10] = jj_gen;
;
}
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case CLUSTERED:
case NONCLUSTERED:
ClusterClause();
break;
default:
jj_la1[11] = jj_gen;
;
}
if (jj_2_7(2)) {
IndexQualifier();
} else {
;
}
jj_consume_token(INDEX);
IndexName();
jj_consume_token(ON);
TableName();
IndexColumnList();
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case TABLE:
case INDEX:
case SEQUENCE:
case CONSTRAINT:
case PRIMARY:
case KEY:
case UNIQUE:
case INTEGER_LITERAL:
case FLOATING_POINT_LITERAL:
case STRING_LITERAL:
case IDENTIFIER:
case LPAREN:
case MINUS:
case ASSIGN:
case 50:
PostObjectTableClauses();
break;
default:
jj_la1[12] = jj_gen;
;
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void Unique() throws ParseException {
/*@bgen(jjtree) Unique */
ASTUnique jjtn000 = (ASTUnique)ASTUnique.jjtCreate(this, JJTUNIQUE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(UNIQUE);
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void ClusterClause() throws ParseException {
/*@bgen(jjtree) ClusterClause */
ASTClusterClause jjtn000 = (ASTClusterClause)ASTClusterClause.jjtCreate(this, JJTCLUSTERCLAUSE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case CLUSTERED:
jj_consume_token(CLUSTERED);
break;
case NONCLUSTERED:
jj_consume_token(NONCLUSTERED);
break;
default:
jj_la1[13] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void DropObjectType() throws ParseException {
/*@bgen(jjtree) DropObjectType */
ASTDropObjectType jjtn000 = (ASTDropObjectType)ASTDropObjectType.jjtCreate(this, JJTDROPOBJECTTYPE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case TABLE:
jj_consume_token(TABLE);
break;
case INDEX:
jj_consume_token(INDEX);
break;
case CONSTRAINT:
jj_consume_token(CONSTRAINT);
break;
default:
jj_la1[14] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void TableName() throws ParseException {
/*@bgen(jjtree) TableName */
ASTTableName jjtn000 = (ASTTableName)ASTTableName.jjtCreate(this, JJTTABLENAME);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
IdentifierName();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void DropObjectName() throws ParseException {
/*@bgen(jjtree) DropObjectName */
ASTDropObjectName jjtn000 = (ASTDropObjectName)ASTDropObjectName.jjtCreate(this, JJTDROPOBJECTNAME);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
IdentifierName();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void ConstraintName() throws ParseException {
/*@bgen(jjtree) ConstraintName */
ASTConstraintName jjtn000 = (ASTConstraintName)ASTConstraintName.jjtCreate(this, JJTCONSTRAINTNAME);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
IdentifierName();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void IndexName() throws ParseException {
/*@bgen(jjtree) IndexName */
ASTIndexName jjtn000 = (ASTIndexName)ASTIndexName.jjtCreate(this, JJTINDEXNAME);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
IdentifierName();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void CreateTableColumnList() throws ParseException {
/*@bgen(jjtree) CreateTableColumnList */
ASTCreateTableColumnList jjtn000 = (ASTCreateTableColumnList)ASTCreateTableColumnList.jjtCreate(this, JJTCREATETABLECOLUMNLIST);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
CreateTableColumn();
label_2:
while (true) {
if (jj_2_8(2)) {
;
} else {
break label_2;
}
jj_consume_token(50);
CreateTableColumn();
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void CreateTableColumn() throws ParseException {
/*@bgen(jjtree) CreateTableColumn */
ASTCreateTableColumn jjtn000 = (ASTCreateTableColumn)ASTCreateTableColumn.jjtCreate(this, JJTCREATETABLECOLUMN);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
ColumnName();
DataType();
if (jj_2_9(2)) {
PostColumnClauses();
} else {
;
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
// only for Sybase IQ, just need to say qualifier
final public void IndexQualifier() throws ParseException {
/*@bgen(jjtree) IndexQualifier */
ASTIndexQualifier jjtn000 = (ASTIndexQualifier)ASTIndexQualifier.jjtCreate(this, JJTINDEXQUALIFIER);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(IDENTIFIER);
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void ColumnName() throws ParseException {
/*@bgen(jjtree) ColumnName */
ASTColumnName jjtn000 = (ASTColumnName)ASTColumnName.jjtCreate(this, JJTCOLUMNNAME);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
IdentifierName();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void DataTypeName() throws ParseException {
/*@bgen(jjtree) DataTypeName */
ASTDataTypeName jjtn000 = (ASTDataTypeName)ASTDataTypeName.jjtCreate(this, JJTDATATYPENAME);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
IdentifierName();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void IdentifierName() throws ParseException {
/*@bgen(jjtree) IdentifierName */
ASTIdentifierName jjtn000 = (ASTIdentifierName)ASTIdentifierName.jjtCreate(this, JJTIDENTIFIERNAME);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case IDENTIFIER:
jj_consume_token(IDENTIFIER);
break;
case TABLE:
jj_consume_token(TABLE);
break;
case INDEX:
jj_consume_token(INDEX);
break;
case SEQUENCE:
jj_consume_token(SEQUENCE);
break;
default:
jj_la1[15] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void DataType() throws ParseException {
/*@bgen(jjtree) DataType */
ASTDataType jjtn000 = (ASTDataType)ASTDataType.jjtCreate(this, JJTDATATYPE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
DataTypeName();
if (jj_2_10(2)) {
jj_consume_token(LPAREN);
DataTypeLenList();
jj_consume_token(RPAREN);
} else {
;
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void DataTypeLenList() throws ParseException {
/*@bgen(jjtree) DataTypeLenList */
ASTDataTypeLenList jjtn000 = (ASTDataTypeLenList)ASTDataTypeLenList.jjtCreate(this, JJTDATATYPELENLIST);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(INTEGER_LITERAL);
label_3:
while (true) {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 50:
;
break;
default:
jj_la1[16] = jj_gen;
break label_3;
}
jj_consume_token(50);
jj_consume_token(INTEGER_LITERAL);
}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void NoCommaSimpleExpression() throws ParseException {
/*@bgen(jjtree) NoCommaSimpleExpression */
ASTNoCommaSimpleExpression jjtn000 = (ASTNoCommaSimpleExpression)ASTNoCommaSimpleExpression.jjtCreate(this, JJTNOCOMMASIMPLEEXPRESSION);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case TABLE:
case INDEX:
case SEQUENCE:
case IDENTIFIER:
IdentifierName();
break;
case STRING_LITERAL:
jj_consume_token(STRING_LITERAL);
break;
case PRIMARY:
jj_consume_token(PRIMARY);
break;
case KEY:
jj_consume_token(KEY);
break;
case UNIQUE:
jj_consume_token(UNIQUE);
break;
case CONSTRAINT:
jj_consume_token(CONSTRAINT);
break;
case INTEGER_LITERAL:
jj_consume_token(INTEGER_LITERAL);
break;
case FLOATING_POINT_LITERAL:
jj_consume_token(FLOATING_POINT_LITERAL);
break;
case MINUS:
jj_consume_token(MINUS);
break;
case ASSIGN:
jj_consume_token(ASSIGN);
break;
default:
jj_la1[17] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void SimpleExpression() throws ParseException {
/*@bgen(jjtree) SimpleExpression */
ASTSimpleExpression jjtn000 = (ASTSimpleExpression)ASTSimpleExpression.jjtCreate(this, JJTSIMPLEEXPRESSION);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case TABLE:
case INDEX:
case SEQUENCE:
case CONSTRAINT:
case PRIMARY:
case KEY:
case UNIQUE:
case INTEGER_LITERAL:
case FLOATING_POINT_LITERAL:
case STRING_LITERAL:
case IDENTIFIER:
case MINUS:
case ASSIGN:
NoCommaSimpleExpression();
break;
case 50:
jj_consume_token(50);
break;
default:
jj_la1[18] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
/****** START EXPRESSION BLOCK THAT ALLOWS COMMAS ******/
final public void ExpressionList() throws ParseException {
/*@bgen(jjtree) ExpressionList */
ASTExpressionList jjtn000 = (ASTExpressionList)ASTExpressionList.jjtCreate(this, JJTEXPRESSIONLIST);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
Expression();
label_4:
while (true) {
if (jj_2_11(500)) {
;
} else {
break label_4;
}
Expression();
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void Expression() throws ParseException {
/*@bgen(jjtree) Expression */
ASTExpression jjtn000 = (ASTExpression)ASTExpression.jjtCreate(this, JJTEXPRESSION);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case TABLE:
case INDEX:
case SEQUENCE:
case CONSTRAINT:
case PRIMARY:
case KEY:
case UNIQUE:
case INTEGER_LITERAL:
case FLOATING_POINT_LITERAL:
case STRING_LITERAL:
case IDENTIFIER:
case MINUS:
case ASSIGN:
case 50:
SimpleExpression();
break;
case LPAREN:
NestedExpression();
break;
default:
jj_la1[19] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void NestedExpression() throws ParseException {
/*@bgen(jjtree) NestedExpression */
ASTNestedExpression jjtn000 = (ASTNestedExpression)ASTNestedExpression.jjtCreate(this, JJTNESTEDEXPRESSION);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(LPAREN);
NestedExpressionList();
label_5:
while (true) {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 50:
;
break;
default:
jj_la1[20] = jj_gen;
break label_5;
}
jj_consume_token(50);
NestedExpressionList();
}
jj_consume_token(RPAREN);
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void NestedExpressionList() throws ParseException {
/*@bgen(jjtree) NestedExpressionList */
ASTNestedExpressionList jjtn000 = (ASTNestedExpressionList)ASTNestedExpressionList.jjtCreate(this, JJTNESTEDEXPRESSIONLIST);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
Expression();
label_6:
while (true) {
if (jj_2_12(2)) {
;
} else {
break label_6;
}
Expression();
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
/****** END EXPRESSION BLOCK THAT ALLOWS COMMAS ******/
/****** START EXPRESSION BLOCK THAT NoComma ******/
final public void NoCommaExpressionList() throws ParseException {
/*@bgen(jjtree) NoCommaExpressionList */
ASTNoCommaExpressionList jjtn000 = (ASTNoCommaExpressionList)ASTNoCommaExpressionList.jjtCreate(this, JJTNOCOMMAEXPRESSIONLIST);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
NoCommaExpression();
label_7:
while (true) {
if (jj_2_13(2)) {
;
} else {
break label_7;
}
NoCommaExpression();
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void NoCommaExpression() throws ParseException {
/*@bgen(jjtree) NoCommaExpression */
ASTNoCommaExpression jjtn000 = (ASTNoCommaExpression)ASTNoCommaExpression.jjtCreate(this, JJTNOCOMMAEXPRESSION);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case TABLE:
case INDEX:
case SEQUENCE:
case CONSTRAINT:
case PRIMARY:
case KEY:
case UNIQUE:
case INTEGER_LITERAL:
case FLOATING_POINT_LITERAL:
case STRING_LITERAL:
case IDENTIFIER:
case MINUS:
case ASSIGN:
NoCommaSimpleExpression();
break;
case LPAREN:
NoCommaNestedExpression();
break;
default:
jj_la1[21] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void NoCommaNestedExpression() throws ParseException {
/*@bgen(jjtree) NoCommaNestedExpression */
ASTNoCommaNestedExpression jjtn000 = (ASTNoCommaNestedExpression)ASTNoCommaNestedExpression.jjtCreate(this, JJTNOCOMMANESTEDEXPRESSION);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(LPAREN);
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case TABLE:
case INDEX:
case SEQUENCE:
case CONSTRAINT:
case PRIMARY:
case KEY:
case UNIQUE:
case INTEGER_LITERAL:
case FLOATING_POINT_LITERAL:
case STRING_LITERAL:
case IDENTIFIER:
case LPAREN:
case MINUS:
case ASSIGN:
NoCommaNestedExpressionList();
label_8:
while (true) {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case 50:
;
break;
default:
jj_la1[22] = jj_gen;
break label_8;
}
jj_consume_token(50);
NoCommaNestedExpressionList();
}
break;
default:
jj_la1[23] = jj_gen;
;
}
jj_consume_token(RPAREN);
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void NoCommaNestedExpressionList() throws ParseException {
/*@bgen(jjtree) NoCommaNestedExpressionList */
ASTNoCommaNestedExpressionList jjtn000 = (ASTNoCommaNestedExpressionList)ASTNoCommaNestedExpressionList.jjtCreate(this, JJTNOCOMMANESTEDEXPRESSIONLIST);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
NoCommaExpression();
label_9:
while (true) {
if (jj_2_14(2)) {
;
} else {
break label_9;
}
NoCommaExpression();
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
/****** END EXPRESSION BLOCK THAT NoComma ******/
final public void PostColumnClauses() throws ParseException {
/*@bgen(jjtree) PostColumnClauses */
ASTPostColumnClauses jjtn000 = (ASTPostColumnClauses)ASTPostColumnClauses.jjtCreate(this, JJTPOSTCOLUMNCLAUSES);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
NoCommaExpressionList();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void PostConstraintClauses() throws ParseException {
/*@bgen(jjtree) PostConstraintClauses */
ASTPostConstraintClauses jjtn000 = (ASTPostConstraintClauses)ASTPostConstraintClauses.jjtCreate(this, JJTPOSTCONSTRAINTCLAUSES);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
NoCommaExpressionList();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void PostObjectTableClauses() throws ParseException {
/*@bgen(jjtree) PostObjectTableClauses */
ASTPostObjectTableClauses jjtn000 = (ASTPostObjectTableClauses)ASTPostObjectTableClauses.jjtCreate(this, JJTPOSTOBJECTTABLECLAUSES);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
ExpressionList();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void OtherConstraintClause() throws ParseException {
/*@bgen(jjtree) OtherConstraintClause */
ASTOtherConstraintClause jjtn000 = (ASTOtherConstraintClause)ASTOtherConstraintClause.jjtCreate(this, JJTOTHERCONSTRAINTCLAUSE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
ExpressionList();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
final public void IndexColumnList() throws ParseException {
/*@bgen(jjtree) IndexColumnList */
ASTIndexColumnList jjtn000 = (ASTIndexColumnList)ASTIndexColumnList.jjtCreate(this, JJTINDEXCOLUMNLIST);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
NestedExpression();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
private boolean jj_2_1(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_1(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(0, xla); }
}
private boolean jj_2_2(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_2(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(1, xla); }
}
private boolean jj_2_3(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_3(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(2, xla); }
}
private boolean jj_2_4(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_4(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(3, xla); }
}
private boolean jj_2_5(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_5(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(4, xla); }
}
private boolean jj_2_6(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_6(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(5, xla); }
}
private boolean jj_2_7(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_7(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(6, xla); }
}
private boolean jj_2_8(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_8(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(7, xla); }
}
private boolean jj_2_9(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_9(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(8, xla); }
}
private boolean jj_2_10(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_10(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(9, xla); }
}
private boolean jj_2_11(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_11(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(10, xla); }
}
private boolean jj_2_12(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_12(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(11, xla); }
}
private boolean jj_2_13(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_13(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(12, xla); }
}
private boolean jj_2_14(int xla) {
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_14(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(13, xla); }
}
private boolean jj_3R_45() {
if (jj_3R_46()) return true;
return false;
}
private boolean jj_3R_35() {
if (jj_scan_token(CONSTRAINT)) return true;
return false;
}
private boolean jj_3R_29() {
if (jj_3R_34()) return true;
return false;
}
private boolean jj_3R_40() {
if (jj_scan_token(LPAREN)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_45()) jj_scanpos = xsp;
if (jj_scan_token(RPAREN)) return true;
return false;
}
private boolean jj_3R_11() {
Token xsp;
xsp = jj_scanpos;
if (jj_3R_22()) {
jj_scanpos = xsp;
if (jj_3R_23()) {
jj_scanpos = xsp;
if (jj_3R_24()) return true;
}
}
return false;
}
private boolean jj_3R_22() {
if (jj_3R_35()) return true;
return false;
}
private boolean jj_3R_15() {
if (jj_scan_token(IDENTIFIER)) return true;
return false;
}
private boolean jj_3R_32() {
if (jj_3R_39()) return true;
return false;
}
private boolean jj_3R_20() {
Token xsp;
xsp = jj_scanpos;
if (jj_3R_32()) {
jj_scanpos = xsp;
if (jj_3R_33()) return true;
}
return false;
}
private boolean jj_3_1() {
if (jj_3R_10()) return true;
return false;
}
private boolean jj_3R_28() {
if (jj_3R_20()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3_13()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_10() {
if (jj_scan_token(TABLE)) return true;
if (jj_3R_21()) return true;
if (jj_scan_token(LPAREN)) return true;
return false;
}
private boolean jj_3R_16() {
if (jj_3R_29()) return true;
return false;
}
private boolean jj_3R_31() {
if (jj_3R_37()) return true;
return false;
}
private boolean jj_3_8() {
if (jj_scan_token(50)) return true;
if (jj_3R_16()) return true;
return false;
}
private boolean jj_3_12() {
if (jj_3R_19()) return true;
return false;
}
private boolean jj_3R_41() {
if (jj_3R_19()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3_12()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_43() {
if (jj_scan_token(50)) return true;
if (jj_3R_41()) return true;
return false;
}
private boolean jj_3_5() {
if (jj_3R_14()) return true;
return false;
}
private boolean jj_3_6() {
if (jj_3R_14()) return true;
return false;
}
private boolean jj_3_11() {
if (jj_3R_19()) return true;
return false;
}
private boolean jj_3R_37() {
if (jj_scan_token(LPAREN)) return true;
if (jj_3R_41()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_43()) { jj_scanpos = xsp; break; }
}
if (jj_scan_token(RPAREN)) return true;
return false;
}
private boolean jj_3_7() {
if (jj_3R_15()) return true;
return false;
}
private boolean jj_3R_19() {
Token xsp;
xsp = jj_scanpos;
if (jj_3R_30()) {
jj_scanpos = xsp;
if (jj_3R_31()) return true;
}
return false;
}
private boolean jj_3R_30() {
if (jj_3R_38()) return true;
return false;
}
private boolean jj_3R_21() {
if (jj_3R_34()) return true;
return false;
}
private boolean jj_3R_42() {
if (jj_3R_39()) return true;
return false;
}
private boolean jj_3R_38() {
Token xsp;
xsp = jj_scanpos;
if (jj_3R_42()) {
jj_scanpos = xsp;
if (jj_scan_token(50)) return true;
}
return false;
}
private boolean jj_3R_26() {
if (jj_3R_37()) return true;
return false;
}
private boolean jj_3R_36() {
Token xsp;
xsp = jj_scanpos;
if (jj_scan_token(29)) {
jj_scanpos = xsp;
if (jj_scan_token(30)) return true;
}
return false;
}
private boolean jj_3R_24() {
if (jj_3R_13()) return true;
return false;
}
private boolean jj_3R_44() {
if (jj_3R_34()) return true;
return false;
}
private boolean jj_3R_39() {
Token xsp;
xsp = jj_scanpos;
if (jj_3R_44()) {
jj_scanpos = xsp;
if (jj_scan_token(37)) {
jj_scanpos = xsp;
if (jj_scan_token(24)) {
jj_scanpos = xsp;
if (jj_scan_token(26)) {
jj_scanpos = xsp;
if (jj_scan_token(28)) {
jj_scanpos = xsp;
if (jj_scan_token(23)) {
jj_scanpos = xsp;
if (jj_scan_token(31)) {
jj_scanpos = xsp;
if (jj_scan_token(35)) {
jj_scanpos = xsp;
if (jj_scan_token(43)) {
jj_scanpos = xsp;
if (jj_scan_token(44)) return true;
}
}
}
}
}
}
}
}
}
return false;
}
private boolean jj_3_10() {
if (jj_scan_token(LPAREN)) return true;
if (jj_3R_18()) return true;
return false;
}
private boolean jj_3R_25() {
if (jj_3R_36()) return true;
return false;
}
private boolean jj_3R_27() {
if (jj_3R_36()) return true;
return false;
}
private boolean jj_3R_18() {
if (jj_scan_token(INTEGER_LITERAL)) return true;
return false;
}
private boolean jj_3R_14() {
if (jj_3R_28()) return true;
return false;
}
private boolean jj_3R_33() {
if (jj_3R_40()) return true;
return false;
}
private boolean jj_3_2() {
if (jj_scan_token(50)) return true;
if (jj_3R_11()) return true;
return false;
}
private boolean jj_3R_13() {
if (jj_scan_token(UNIQUE)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_27()) jj_scanpos = xsp;
if (jj_3R_26()) return true;
return false;
}
private boolean jj_3R_17() {
if (jj_3R_28()) return true;
return false;
}
private boolean jj_3R_23() {
if (jj_3R_12()) return true;
return false;
}
private boolean jj_3R_34() {
Token xsp;
xsp = jj_scanpos;
if (jj_scan_token(38)) {
jj_scanpos = xsp;
if (jj_scan_token(18)) {
jj_scanpos = xsp;
if (jj_scan_token(19)) {
jj_scanpos = xsp;
if (jj_scan_token(21)) return true;
}
}
}
return false;
}
private boolean jj_3R_12() {
if (jj_scan_token(PRIMARY)) return true;
if (jj_scan_token(KEY)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_25()) jj_scanpos = xsp;
if (jj_3R_26()) return true;
return false;
}
private boolean jj_3_9() {
if (jj_3R_17()) return true;
return false;
}
private boolean jj_3_14() {
if (jj_3R_20()) return true;
return false;
}
private boolean jj_3_4() {
if (jj_3R_13()) return true;
return false;
}
private boolean jj_3_13() {
if (jj_3R_20()) return true;
return false;
}
private boolean jj_3R_46() {
if (jj_3R_20()) return true;
return false;
}
private boolean jj_3_3() {
if (jj_3R_12()) return true;
return false;
}
/** Generated Token Manager. */
public SqlParserTokenManager token_source;
JavaCharStream jj_input_stream;
/** Current token. */
public Token token;
/** Next token. */
public Token jj_nt;
private int jj_ntk;
private Token jj_scanpos, jj_lastpos;
private int jj_la;
private int jj_gen;
final private int[] jj_la1 = new int[24];
static private int[] jj_la1_0;
static private int[] jj_la1_1;
static {
jj_la1_init_0();
jj_la1_init_1();
}
private static void jj_la1_init_0() {
jj_la1_0 = new int[] {0x2c000,0x30000,0x95ac0000,0x70080000,0x0,0x95ac0000,0x11800000,0x95ac0000,0x60000000,0x60000000,0x10000000,0x60000000,0x95ac0000,0x60000000,0x8c0000,0x2c0000,0x0,0x95ac0000,0x95ac0000,0x95ac0000,0x0,0x95ac0000,0x0,0x95ac0000,};
}
private static void jj_la1_init_1() {
jj_la1_1 = new int[] {0x0,0x0,0x41a68,0x40,0x40000,0x41a68,0x0,0x41a68,0x0,0x0,0x0,0x0,0x41a68,0x0,0x0,0x40,0x40000,0x1868,0x41868,0x41a68,0x40000,0x1a68,0x40000,0x1a68,};
}
final private JJCalls[] jj_2_rtns = new JJCalls[14];
private boolean jj_rescan = false;
private int jj_gc = 0;
/** Constructor with InputStream. */
public SqlParser(java.io.InputStream stream) {
this(stream, null);
}
/** Constructor with InputStream and supplied encoding */
public SqlParser(java.io.InputStream stream, String encoding) {
try { jj_input_stream = new JavaCharStream(stream, encoding, 1, 1); } catch(java.io.UnsupportedEncodingException e) { throw new RuntimeException(e); }
token_source = new SqlParserTokenManager(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 24; i++) jj_la1[i] = -1;
for (int i = 0; i < jj_2_rtns.length; i++) jj_2_rtns[i] = new JJCalls();
}
/** Reinitialise. */
public void ReInit(java.io.InputStream stream) {
ReInit(stream, null);
}
/** Reinitialise. */
public void ReInit(java.io.InputStream stream, String encoding) {
try { jj_input_stream.ReInit(stream, encoding, 1, 1); } catch(java.io.UnsupportedEncodingException e) { throw new RuntimeException(e); }
token_source.ReInit(jj_input_stream);
token = new Token();
jj_ntk = -1;
jjtree.reset();
jj_gen = 0;
for (int i = 0; i < 24; i++) jj_la1[i] = -1;
for (int i = 0; i < jj_2_rtns.length; i++) jj_2_rtns[i] = new JJCalls();
}
/** Constructor. */
public SqlParser(java.io.Reader stream) {
jj_input_stream = new JavaCharStream(stream, 1, 1);
token_source = new SqlParserTokenManager(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 24; i++) jj_la1[i] = -1;
for (int i = 0; i < jj_2_rtns.length; i++) jj_2_rtns[i] = new JJCalls();
}
/** Reinitialise. */
public void ReInit(java.io.Reader stream) {
jj_input_stream.ReInit(stream, 1, 1);
token_source.ReInit(jj_input_stream);
token = new Token();
jj_ntk = -1;
jjtree.reset();
jj_gen = 0;
for (int i = 0; i < 24; i++) jj_la1[i] = -1;
for (int i = 0; i < jj_2_rtns.length; i++) jj_2_rtns[i] = new JJCalls();
}
/** Constructor with generated Token Manager. */
public SqlParser(SqlParserTokenManager tm) {
token_source = tm;
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 24; i++) jj_la1[i] = -1;
for (int i = 0; i < jj_2_rtns.length; i++) jj_2_rtns[i] = new JJCalls();
}
/** Reinitialise. */
public void ReInit(SqlParserTokenManager tm) {
token_source = tm;
token = new Token();
jj_ntk = -1;
jjtree.reset();
jj_gen = 0;
for (int i = 0; i < 24; i++) jj_la1[i] = -1;
for (int i = 0; i < jj_2_rtns.length; i++) jj_2_rtns[i] = new JJCalls();
}
private Token jj_consume_token(int kind) throws ParseException {
Token oldToken;
if ((oldToken = token).next != null) token = token.next;
else token = token.next = token_source.getNextToken();
jj_ntk = -1;
if (token.kind == kind) {
jj_gen++;
if (++jj_gc > 100) {
jj_gc = 0;
for (int i = 0; i < jj_2_rtns.length; i++) {
JJCalls c = jj_2_rtns[i];
while (c != null) {
if (c.gen < jj_gen) c.first = null;
c = c.next;
}
}
}
return token;
}
token = oldToken;
jj_kind = kind;
throw generateParseException();
}
static private final class LookaheadSuccess extends java.lang.Error { }
final private LookaheadSuccess jj_ls = new LookaheadSuccess();
private boolean jj_scan_token(int kind) {
if (jj_scanpos == jj_lastpos) {
jj_la--;
if (jj_scanpos.next == null) {
jj_lastpos = jj_scanpos = jj_scanpos.next = token_source.getNextToken();
} else {
jj_lastpos = jj_scanpos = jj_scanpos.next;
}
} else {
jj_scanpos = jj_scanpos.next;
}
if (jj_rescan) {
int i = 0; Token tok = token;
while (tok != null && tok != jj_scanpos) { i++; tok = tok.next; }
if (tok != null) jj_add_error_token(kind, i);
}
if (jj_scanpos.kind != kind) return true;
if (jj_la == 0 && jj_scanpos == jj_lastpos) throw jj_ls;
return false;
}
/** Get the next Token. */
final public Token getNextToken() {
if (token.next != null) token = token.next;
else token = token.next = token_source.getNextToken();
jj_ntk = -1;
jj_gen++;
return token;
}
/** Get the specific Token. */
final public Token getToken(int index) {
Token t = token;
for (int i = 0; i < index; i++) {
if (t.next != null) t = t.next;
else t = t.next = token_source.getNextToken();
}
return t;
}
private int jj_ntk() {
if ((jj_nt=token.next) == null)
return (jj_ntk = (token.next=token_source.getNextToken()).kind);
else
return (jj_ntk = jj_nt.kind);
}
private java.util.List jj_expentries = new java.util.ArrayList();
private int[] jj_expentry;
private int jj_kind = -1;
private int[] jj_lasttokens = new int[100];
private int jj_endpos;
private void jj_add_error_token(int kind, int pos) {
if (pos >= 100) return;
if (pos == jj_endpos + 1) {
jj_lasttokens[jj_endpos++] = kind;
} else if (jj_endpos != 0) {
jj_expentry = new int[jj_endpos];
for (int i = 0; i < jj_endpos; i++) {
jj_expentry[i] = jj_lasttokens[i];
}
jj_entries_loop: for (java.util.Iterator> it = jj_expentries.iterator(); it.hasNext();) {
int[] oldentry = (int[])(it.next());
if (oldentry.length == jj_expentry.length) {
for (int i = 0; i < jj_expentry.length; i++) {
if (oldentry[i] != jj_expentry[i]) {
continue jj_entries_loop;
}
}
jj_expentries.add(jj_expentry);
break jj_entries_loop;
}
}
if (pos != 0) jj_lasttokens[(jj_endpos = pos) - 1] = kind;
}
}
/** Generate ParseException. */
public ParseException generateParseException() {
jj_expentries.clear();
boolean[] la1tokens = new boolean[51];
if (jj_kind >= 0) {
la1tokens[jj_kind] = true;
jj_kind = -1;
}
for (int i = 0; i < 24; i++) {
if (jj_la1[i] == jj_gen) {
for (int j = 0; j < 32; j++) {
if ((jj_la1_0[i] & (1< jj_gen) {
jj_la = p.arg; jj_lastpos = jj_scanpos = p.first;
switch (i) {
case 0: jj_3_1(); break;
case 1: jj_3_2(); break;
case 2: jj_3_3(); break;
case 3: jj_3_4(); break;
case 4: jj_3_5(); break;
case 5: jj_3_6(); break;
case 6: jj_3_7(); break;
case 7: jj_3_8(); break;
case 8: jj_3_9(); break;
case 9: jj_3_10(); break;
case 10: jj_3_11(); break;
case 11: jj_3_12(); break;
case 12: jj_3_13(); break;
case 13: jj_3_14(); break;
}
}
p = p.next;
} while (p != null);
} catch(LookaheadSuccess ls) { }
}
jj_rescan = false;
}
private void jj_save(int index, int xla) {
JJCalls p = jj_2_rtns[index];
while (p.gen > jj_gen) {
if (p.next == null) { p = p.next = new JJCalls(); break; }
p = p.next;
}
p.gen = jj_gen + xla - jj_la; p.first = token; p.arg = xla;
}
static final class JJCalls {
int gen;
Token first;
int arg;
JJCalls next;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy