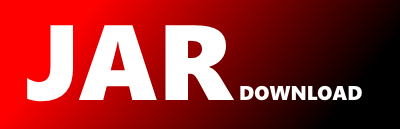
com.gs.fw.common.mithra.finder.NotExistsOperation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reladomo Show documentation
Show all versions of reladomo Show documentation
Reladomo is an object-relational mapping framework.
/*
Copyright 2016 Goldman Sachs.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.gs.fw.common.mithra.finder;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.gs.fw.common.mithra.MithraObjectPortal;
import com.gs.fw.common.mithra.attribute.AsOfAttribute;
import com.gs.fw.common.mithra.attribute.Attribute;
import com.gs.fw.common.mithra.cache.ConcurrentFullUniqueIndex;
import com.gs.fw.common.mithra.cache.ExtractorBasedHashStrategy;
import com.gs.fw.common.mithra.cache.FullUniqueIndex;
import com.gs.fw.common.mithra.extractor.Extractor;
import com.gs.fw.common.mithra.finder.sqcache.ExactMatchSmr;
import com.gs.fw.common.mithra.finder.sqcache.NoMatchRequiresExactSmr;
import com.gs.fw.common.mithra.finder.sqcache.ShapeMatchResult;
import com.gs.fw.common.mithra.notification.MithraDatabaseIdentifierExtractor;
import com.gs.fw.common.mithra.util.*;
import com.gs.reladomo.metadata.PrivateReladomoClassMetaData;
public class NotExistsOperation implements Operation
{
private Operation op;
private Mapper mapper;
private transient NotExistsOperation originalContainer;
private static final long serialVersionUID = 1674543777147403981L;
public NotExistsOperation(Mapper mapper, Operation op)
{
this.op = op;
this.mapper = mapper;
this.originalContainer = this;
if (mapper instanceof LinkedMapper)
{
LinkedMapper linkedMapper = (LinkedMapper) mapper;
List mappers = linkedMapper.getMappers();
this.mapper = (Mapper) mappers.get(0);
for (int i = mappers.size() - 1; i > 0; i--)
{
Mapper m = (Mapper) mappers.get(i);
this.op = new NotExistsOperation(m, this.op);
}
}
}
protected NotExistsOperation(Mapper mapper, Operation op, NotExistsOperation originalContainer)
{
this(mapper, op);
this.originalContainer = originalContainer;
}
public List applyOperationToFullCache()
{
List everything = this.mapper.getAllPossibleResultObjectsForFullCache();
if (everything == null)
{
return null;
}
return applyOperation(everything);
}
public List applyOperationToPartialCache()
{
return null;
}
private List applyOneByOne(List joinedList, Mapper reverseMapper, Operation defaults)
{
Operation toApplyWith = this.op;
Operation toApplyAfter = null;
if (!AbstractMapper.isOperationEligibleForMapperCombo(toApplyWith))
{
toApplyWith = defaults;
toApplyAfter = this.op;
}
else if (defaults != null)
{
toApplyWith = toApplyWith.and(defaults);
}
Set leftAttributes = mapper.getAllLeftAttributes();
Extractor[] leftExtractors = new Extractor[leftAttributes.size()];
leftAttributes.toArray(leftExtractors);
FullUniqueIndex accepted = new FullUniqueIndex("", leftExtractors);
FullUniqueIndex rejected = new FullUniqueIndex("", leftExtractors);
MithraFastList result = new MithraFastList();
for(int i=0;i 0)
{
rejected.put(joined);
if (rejected.size() == 1024)
{
rejected.ensureCapacity(joinedList.size()/i*accepted.size());
}
}
else
{
accepted.put(joined);
result.add(joined);
if (accepted.size() == 1024)
{
accepted.ensureCapacity(joinedList.size()/i*accepted.size());
}
}
}
return result;
}
private Operation getDefaultOrExistingAsOfOps(AsOfAttribute[] rightAsOfAttributes, Mapper reverseMapper)
{
AsOfAttribute[] probe = new AsOfAttribute[1];
Operation defaults = NoOperation.instance();
for(int i=0;i 1 && MithraCpuBoundThreadPool.isParallelizable(filteredList.size()))
{
return parallelMatchLeftToRight(filteredList, rightIndex, extractors);
}
MithraFastList result = new MithraFastList(filteredList.size());
if (filteredList.size() != originalList.size())
{
FullUniqueIndex filtered = new FullUniqueIndex(ExtractorBasedHashStrategy.IDENTITY_HASH_STRATEGY, filteredList.size());
filtered.addAll(filteredList);
for(int i=0;i result)
{
result.addAll(mapper.getAllLeftAttributes());
}
public Operation zSubstituteForTempJoin(Map attributeMap, Object prototypeObject)
{
Mapper newMapper = this.mapper.createMapperForTempJoin(attributeMap, prototypeObject, 0);
return new NotExistsOperation(newMapper, this.op);
}
public Operation zGetAsOfOp(AsOfAttribute asOfAttribute)
{
return null;
}
public void generateSql(SqlQuery query)
{
pushContainer(query);
query.setNotExistsForNextOperation();
mapper.generateSql(query);
query.beginAnd();
op.generateSql(query);
query.endAnd();
mapper.popMappers(query);
query.popMapperContainer();
}
private void pushContainer(MapperStack query)
{
query.pushMapperContainer(this.originalContainer == null ? this : this.originalContainer); // can be null when deserialized
}
public int getClauseCount(SqlQuery query)
{
int count = this.op.getClauseCount(query);
count += this.mapper.getClauseCount(query);
return count;
}
public int hashCode()
{
return this.op.hashCode() ^ this.mapper.hashCode() ^ 0x34812695;
}
public boolean equals(Object obj)
{
if (obj instanceof NotExistsOperation)
{
NotExistsOperation other = (NotExistsOperation) obj;
return this.mapper.equals(other.mapper) && this.op.equals(other.op);
}
return false;
}
public void addDependentPortalsToSet(Set set)
{
mapper.addDepenedentPortalsToSet(set);
op.addDependentPortalsToSet(set);
}
public void addDepenedentAttributesToSet(Set set)
{
mapper.addDepenedentAttributesToSet(set);
op.addDepenedentAttributesToSet(set);
}
public boolean isJoinedWith(MithraObjectPortal portal)
{
return this.mapper.isJoinedWith(portal);
}
protected Mapper getMapper()
{
return mapper;
}
public Operation zCombinedAndWithMapped(NotExistsOperation op)
{
return null;
}
public Operation zCombinedAndWithMultiEquality(MultiEqualityOperation op)
{
return null;
}
@Override
public Operation zCombinedAndWithRange(RangeOperation op)
{
return null;
}
public Operation zCombinedAndWithIn(InOperation op)
{
return null;
}
public Operation zCombinedAndWithMapped(MappedOperation op)
{
return null;
}
public Operation zCombinedAnd(Operation op)
{
return null;
}
public Operation zCombinedAndWithAtomicEquality(AtomicEqualityOperation op)
{
return null;
}
public void registerAsOfAttributesAndOperations(AsOfEqualityChecker checker)
{
pushContainer(checker);
this.mapper.registerAsOfAttributesAndOperations(checker);
this.op.registerAsOfAttributesAndOperations(checker);
this.mapper.popMappers(checker);
checker.popMapperContainer();
}
public Operation insertAsOfEqOperation(AtomicOperation[] asOfEqOperations, MapperStackImpl insertPosition, AsOfEqualityChecker stack)
{
if (insertPosition.equals(stack.getCurrentMapperList()))
{
return new AndOperation(MultiEqualityOperation.createEqOperation(asOfEqOperations), this);
}
pushContainer(stack);
this.mapper.pushMappers(stack);
Operation newOperation = this.op.insertAsOfEqOperation(asOfEqOperations, insertPosition, stack);
this.mapper.popMappers(stack);
if (newOperation != null)
{
stack.popMapperContainer();
Operation result = new NotExistsOperation(this.getMapper(), newOperation, this);
stack.substituteContainer(this, result);
return result;
}
Mapper newMapper = this.mapper.insertAsOfOperationInMiddle(asOfEqOperations, insertPosition, stack);
stack.popMapperContainer();
if (newMapper != null)
{
Operation result = new NotExistsOperation(newMapper, this.op, this);
stack.substituteContainer(this, result);
return result;
}
return null;
}
public Operation zInsertTransitiveOps(MapperStack insertPosition, InternalList toInsert, TransitivePropagator transitivePropagator)
{
return this;
}
public Operation zInsertAsOfEqOperationOnLeft(AtomicOperation[] asOfEqOperations)
{
return new NotExistsOperation(mapper.insertAsOfOperationOnLeft(asOfEqOperations), this.op, this);
}
public void registerOperation(MithraDatabaseIdentifierExtractor extractor, boolean registerEquality)
{
pushContainer(extractor);
mapper.registerOperation(extractor, registerEquality);
op.registerOperation(extractor, registerEquality);
mapper.popMappers(extractor);
extractor.popMapperContainer();
}
public boolean zHasAsOfOperation()
{
return false;
}
public Operation zFlipToOneMapper(Mapper mapper)
{
return null;
}
public Boolean matches(Object o)
{
List result = this.applyOperation(Collections.singletonList(o));
if (result == null) return null;
return result.size() == 1;
}
public boolean zPrefersBulkMatching()
{
return true;
}
@Override
public String toString()
{
return ToStringContext.createAndToString(this);
}
@Override
public boolean zContainsMappedOperation()
{
return true;
}
@Override
public boolean zHasParallelApply()
{
return true;
}
@Override
public boolean zCanFilterInMemory()
{
return false;
}
@Override
public boolean zIsShapeCachable()
{
return false;
}
@Override
public ShapeMatchResult zShapeMatch(Operation existingOperation)
{
return this.equals(existingOperation) ? ExactMatchSmr.INSTANCE : NoMatchRequiresExactSmr.INSTANCE;
}
@Override
public int zShapeHash()
{
return this.hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy