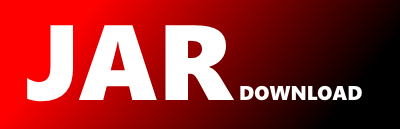
com.gs.fw.common.mithra.notification.RunsMasterQueueDatabaseObjectAbstract Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reladomo Show documentation
Show all versions of reladomo Show documentation
Reladomo is an object-relational mapping framework.
package com.gs.fw.common.mithra.notification;
import java.math.BigDecimal;
import java.sql.Timestamp;
import java.util.*;
import java.io.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.attribute.*;
import com.gs.fw.common.mithra.util.*;
import com.gs.fw.common.mithra.notification.*;
import com.gs.fw.common.mithra.notification.listener.*;
import com.gs.fw.common.mithra.list.cursor.Cursor;
import com.gs.fw.common.mithra.bulkloader.*;
import java.util.*;
import java.sql.*;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.attribute.update.AttributeUpdateWrapper;
import com.gs.fw.common.mithra.bulkloader.BulkLoader;
import com.gs.fw.common.mithra.bulkloader.BulkLoaderException;
import com.gs.fw.common.mithra.cache.*;
import com.gs.fw.common.mithra.cache.offheap.*;
import com.gs.fw.common.mithra.connectionmanager.*;
import com.gs.fw.common.mithra.database.*;
import com.gs.fw.common.mithra.databasetype.*;
import com.gs.fw.common.mithra.finder.*;
import com.gs.fw.common.mithra.finder.orderby.OrderBy;
import com.gs.fw.common.mithra.finder.integer.IntegerResultSetParser;
import com.gs.fw.common.mithra.querycache.CachedQuery;
import com.gs.fw.common.mithra.remote.RemoteMithraService;
import com.gs.fw.common.mithra.transaction.BatchUpdateOperation;
import com.gs.fw.common.mithra.transaction.UpdateOperation;
/**
* This file was automatically generated using Mithra 16.7.1. Please do not modify it.
* Add custom logic to its subclass instead.
*/
public abstract class RunsMasterQueueDatabaseObjectAbstract extends MithraAbstractTransactionalDatabaseObject implements MithraTransactionalDatabaseObject, MithraObjectFactory
{
private IntSourceConnectionManager connectionManager;
private IntSourceSchemaManager schemaManager;
private IntSourceTablePartitionManager tablePartitionManager;
private static final String COL_LIST_WITHOUT_PK = "last_update_time,entity_n,last_update_userid,rs_origin_xact_id";
private static final String COL_LIST_WITHOUT_PK_WITH_ALIAS = "t0.last_update_time,t0.entity_n,t0.last_update_userid,t0.rs_origin_xact_id";
private static final String PK_WITH_ALIAS = "t0.event_seq_no = ?";
private static final String PK_INDEX_COLS = "event_seq_no";
protected RunsMasterQueueDatabaseObjectAbstract()
{
super("RunsMasterQueue", "com.gs.fw.common.mithra.notification.RunsMasterQueueFinder",
5, 5,
COL_LIST_WITHOUT_PK, COL_LIST_WITHOUT_PK_WITH_ALIAS,
false, false, true,
PK_WITH_ALIAS,
PK_INDEX_COLS);
}
public MithraObjectPortal getMithraObjectPortal()
{
return RunsMasterQueueFinder.getMithraObjectPortal();
}
public RelatedFinder getFinder()
{
return RunsMasterQueueFinder.getFinderInstance();
}
public static RunsMasterQueueData allocateOnHeapData()
{
return new RunsMasterQueueData();
}
public static RunsMasterQueueData allocateOffHeapData()
{
throw new RuntimeException("no off heap implementation");
}
public MithraDataObject deserializeFullData(ObjectInput in) throws IOException, ClassNotFoundException
{
MithraDataObject data = new RunsMasterQueueData();
data.zDeserializeFullData(in);
return data;
}
public MithraObject deserializeForRefresh(ObjectInput in) throws IOException, ClassNotFoundException
{
RunsMasterQueueData data = new RunsMasterQueueData();
data.zDeserializePrimaryKey(in);
return this.createObject(data);
}
public Cache instantiateFullCache(MithraConfigurationManager.Config config)
{
Cache result;
if (config.isParticipatingInTx())
{
result = new FullNonDatedTransactionalCache(RunsMasterQueueFinder.getPrimaryKeyAttributes(), this, RunsMasterQueueFinder.getImmutableAttributes());
}
else
{
result = new FullNonDatedCache(RunsMasterQueueFinder.getPrimaryKeyAttributes(), this, RunsMasterQueueFinder.getImmutableAttributes(), new NonTransactionalUnderlyingObjectGetter());
}
initPortal(result, config);
return result;
}
public Cache instantiatePartialCache(MithraConfigurationManager.Config config)
{
Cache result;
if (config.isParticipatingInTx())
{
result = new PartialNonDatedTransactionalCache(RunsMasterQueueFinder.getPrimaryKeyAttributes(), this, RunsMasterQueueFinder.getImmutableAttributes(), config.getCacheTimeToLive(), config.getRelationshipCacheTimeToLive());
}
else
{
result = new PartialNonDatedCache(RunsMasterQueueFinder.getPrimaryKeyAttributes(), this, RunsMasterQueueFinder.getImmutableAttributes(), new NonTransactionalUnderlyingObjectGetter(), config.getCacheTimeToLive(), config.getRelationshipCacheTimeToLive());
}
initPortal(result, config);
return result;
}
private void initPortal(Cache cache, MithraConfigurationManager.Config config)
{
if (config.isThreeTierClient())
{
RunsMasterQueueFinder.initializeClientPortal(this, cache, config);
}
else
{
RunsMasterQueueFinder.initializePortal(this, cache, config);
}
if (config.isParticipatingInTx())
{
RunsMasterQueue.zConfigFullTx();
}
else
{
RunsMasterQueue.zConfigNonTx();
}
}
public List getSimulatedSequenceInitValues()
{
return null;
}
public Object getSourceAttributeValueForSelectedObjectGeneric(SqlQuery query, int queryNumber)
{
return query.getSourceAttributeValueForSelectedObject(queryNumber);
}
public Object getSourceAttributeValueFromObjectGeneric(MithraDataObject object)
{
RunsMasterQueueData data = (RunsMasterQueueData)object;
return Integer.valueOf(data.getSourceId());
}
public Object getSourceAttributeValueGeneric(SqlQuery query, MapperStackImpl mapperStack, int queryNumber)
{
return query.getSourceAttributeValue(mapperStack,queryNumber);
}
public String getDatabaseIdentifierGenericSource (Object source)
{
return connectionManager.getDatabaseIdentifier(((Integer)source).intValue());
}
public DatabaseType getDatabaseTypeGenericSource(Object source)
{
return connectionManager.getDatabaseType(((Integer)source).intValue());
}
public TimeZone getDatabaseTimeZoneGenericSource(Object source)
{
return getDatabaseTimeZone(((Integer)source).intValue());
}
public Connection getConnectionGenericSource(Object source)
{
return connectionManagerWrapper.getConnection(((Integer)source).intValue());
}
public BulkLoader createBulkLoaderGenericSource(Object source) throws BulkLoaderException
{
return connectionManager.createBulkLoader(((Integer)source).intValue());
}
public MithraDataObject inflateDataGenericSource(ResultSet rs, Object source, DatabaseType dt)
throws SQLException
{
return inflateRunsMasterQueueData(rs, dt ,((Integer)source).intValue());
}
public void inflateNonPkDataGenericSource(MithraDataObject data, ResultSet rs, Object source, DatabaseType dt)
throws SQLException
{
inflateNonPkRunsMasterQueueData(1, (RunsMasterQueueData) data, rs, dt ,((Integer)source).intValue());
}
public MithraDataObject inflatePkDataGenericSource(ResultSet rs, Object source, DatabaseType dt)
throws SQLException
{
return inflateRunsMasterQueuePkData(rs, dt, ((Integer)source).intValue());
}
public String getSchemaGenericSource(Object source)
{
if (this.schemaManager != null)
{
return this.schemaManager.getSchema(this.getDefaultSchema(),((Integer)source).intValue());
}
return this.getDefaultSchema();
}
public String getTableNameGenericSource(Object source) throws MithraDatabaseException
{
return getRunsMasterQueueTableName(((Integer)source).intValue());
}
public String getRunsMasterQueueTableName(int sourceAttribute) throws MithraDatabaseException
{
if (this.tablePartitionManager != null)
{
return this.tablePartitionManager.getTableName(this.getDefaultTableName(), sourceAttribute);
}
return this.getDefaultTableName();
}
public void setPrimaryKeyAttributes(PreparedStatement stm, int pos, MithraDataObject dataObj,
TimeZone databaseTimeZone, DatabaseType dt) throws SQLException
{
RunsMasterQueueData data = (RunsMasterQueueData)dataObj;
TimeZone conversionTimeZone = null;
stm.setInt(pos++, data.getEventId());
}
public int setPrimaryKeyAttributesWithoutOptimistic(PreparedStatement stm, int pos, MithraDataObject dataObj,
TimeZone databaseTimeZone, DatabaseType dt) throws SQLException
{
this.setPrimaryKeyAttributes(stm, pos, dataObj, databaseTimeZone, dt);
return -1;
}
public String getPrimaryKeyWhereSql()
{
return "event_seq_no = ?";
}
public String getPrimaryKeyWhereSqlWithNullableAttribute(MithraDataObject dataObj)
{
return "";
}
public String getPrimaryKeyWhereSqlWithNullableAttributeWithDefaultAlias(MithraDataObject dataObj)
{
return "";
}
public String getColumnListWithPk(String databaseAlias)
{
if (databaseAlias.equals(SqlQuery.DEFAULT_DATABASE_ALIAS))
{
return "t0.event_seq_no,t0.last_update_time,t0.entity_n,t0.last_update_userid,t0.rs_origin_xact_id";
}
StringBuffer result = new StringBuffer((databaseAlias.length()+15)*5);
result.append(databaseAlias).append(".").append("event_seq_no");
result.append(",").append(databaseAlias).append(".").append("last_update_time");
result.append(",").append(databaseAlias).append(".").append("entity_n");
result.append(",").append(databaseAlias).append(".").append("last_update_userid");
result.append(",").append(databaseAlias).append(".").append("rs_origin_xact_id");
return result.toString();
}
public Object getConnectionManager()
{
return connectionManager;
}
public void setConnectionManager(Object connectionManager, ConnectionManagerWrapper wrapper)
{
this.connectionManager = (IntSourceConnectionManager)connectionManager;
this.connectionManagerWrapper = wrapper;
}
public RunsMasterQueueData inflateRunsMasterQueueData(ResultSet rs, DatabaseType dt, int sourceAttribute)
throws SQLException
{
RunsMasterQueueData data = inflateRunsMasterQueuePkData(rs, dt, sourceAttribute);
inflateNonPkRunsMasterQueueData(2, data, rs, dt, sourceAttribute);
return data;
}
public RunsMasterQueueData inflateRunsMasterQueuePkData(ResultSet _rs, DatabaseType _dt, int sourceAttribute)
throws SQLException
{
RunsMasterQueueData _data = new RunsMasterQueueData();
int _pos = 1;
_data.setSourceId(sourceAttribute);
_data.setEventId(_rs.getInt(_pos++));
checkNullPrimitive(_rs, _data, "eventId");
return _data;
}
public void inflateNonPkRunsMasterQueueData(int _pos, RunsMasterQueueData _datax, ResultSet _rs, DatabaseType _dt, int sourceAttribute)
throws SQLException
{
{
RunsMasterQueueData _data = _datax;
Timestamp lastUpdateTimetimestampValue = _dt.getTimestampFromResultSet(_rs, _pos++, MithraTimestamp.DefaultTimeZone);
_data.setLastUpdateTime(lastUpdateTimetimestampValue);
_data.setEntity(trimString(_rs.getString(_pos++)));
_data.setLastUpdateUserId(trimString(_rs.getString(_pos++)));
_data.setTranId(_rs.getBytes(_pos++));
}
}
public DatabaseType getDatabaseType(int sourceAttribute)
{
return connectionManager.getDatabaseType(sourceAttribute);
}
public TimeZone getDatabaseTimeZone(int sourceAttribute)
{
return connectionManager.getDatabaseTimeZone(sourceAttribute);
}
protected String getSchema(int sourceAttribute)
{
return this.getSchemaGenericSource(sourceAttribute);
}
public void setSchemaManager(Object schemaManager)
{
if( schemaManager instanceof IntSourceSchemaManager )
{
this.schemaManager = (IntSourceSchemaManager) schemaManager;
}
else
{
throw new IllegalArgumentException( "Schema manager class " + schemaManager.getClass().getName()
+ " does not implement IntSourceSchemaManager.class" );
}
}
public void setTablePartitionManager(Object tablePartitionManager)
{
if( tablePartitionManager instanceof IntSourceTablePartitionManager )
{
this.tablePartitionManager = (IntSourceTablePartitionManager) tablePartitionManager;
}
else
{
throw new IllegalArgumentException( "Table partition manager class " + tablePartitionManager.getClass().getName()
+ " does not implement IntSourceTablePartitionManager.class" );
}
}
public String getTableName()
{
throw new RuntimeException("not implemented");
}
public String getDefaultTableName()
{
return "ap_UPD_QUEUE";
}
public void setInsertAttributes(PreparedStatement stm, MithraDataObject dataObj,
TimeZone databaseTimeZone, int pos, DatabaseType dt) throws SQLException
{
RunsMasterQueueData data = (RunsMasterQueueData)dataObj;
TimeZone conversionTimeZone = null;
stm.setInt(pos++, data.getEventId());
if (data.isLastUpdateTimeNull())
{
stm.setNull(pos++, java.sql.Types.TIMESTAMP);
}
else
{
conversionTimeZone = MithraTimestamp.DefaultTimeZone;
dt.setTimestamp(stm, pos, data.getLastUpdateTime(), false, conversionTimeZone);
pos++;
}
if (data.isEntityNull())
{
stm.setNull(pos++, java.sql.Types.VARCHAR);
}
else
{
stm.setString(pos++, data.getEntity());
}
if (data.isLastUpdateUserIdNull())
{
stm.setNull(pos++, java.sql.Types.VARCHAR);
}
else
{
stm.setString(pos++, data.getLastUpdateUserId());
}
if (data.isTranIdNull())
{
stm.setNull(pos++, java.sql.Types.VARBINARY);
}
else
{
stm.setBytes(pos++, data.getTranId());
}
}
public String getInsertFields()
{
return "event_seq_no,last_update_time,entity_n,last_update_userid,rs_origin_xact_id";
}
public String getInsertQuestionMarks()
{
return "?,?,?,?,?";
}
public String getOptimisticLockingWhereSql()
{
return "";
}
public MithraObject createObject(MithraDataObject newData)
{
RunsMasterQueue newObject = new RunsMasterQueue();
newObject.zSetFromRunsMasterQueueData((RunsMasterQueueData) newData);
return newObject;
}
public String getPkColumnList(String databaseAlias)
{
if (databaseAlias.equals(SqlQuery.DEFAULT_DATABASE_ALIAS))
{
return "t0.event_seq_no";
}
StringBuffer result = new StringBuffer((databaseAlias.length()+15)*5);
result.append(databaseAlias);
result.append(".");
result.append("event_seq_no");
return result.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy