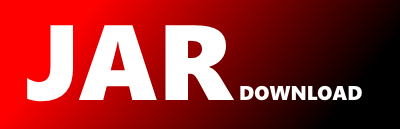
com.gs.fw.common.mithra.notification.RunsMasterQueueFinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reladomo Show documentation
Show all versions of reladomo Show documentation
Reladomo is an object-relational mapping framework.
package com.gs.fw.common.mithra.notification;
import java.math.BigDecimal;
import java.sql.Timestamp;
import java.util.*;
import java.io.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.attribute.*;
import com.gs.fw.common.mithra.util.*;
import com.gs.fw.common.mithra.notification.*;
import com.gs.fw.common.mithra.notification.listener.*;
import com.gs.fw.common.mithra.list.cursor.Cursor;
import com.gs.fw.common.mithra.bulkloader.*;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.attribute.*;
import com.gs.fw.common.mithra.attribute.calculator.procedure.ObjectProcedure;
import com.gs.fw.common.mithra.behavior.txparticipation.*;
import com.gs.fw.common.mithra.cache.Cache;
import com.gs.fw.common.mithra.cache.bean.*;
import com.gs.fw.common.mithra.extractor.*;
import com.gs.fw.common.mithra.finder.*;
import com.gs.fw.common.mithra.finder.booleanop.*;
import com.gs.fw.common.mithra.finder.integer.*;
import com.gs.fw.common.mithra.finder.longop.*;
import com.gs.fw.common.mithra.finder.orderby.OrderBy;
import com.gs.fw.common.mithra.finder.string.*;
import com.gs.fw.common.mithra.extractor.NormalAndListValueSelector;
import com.gs.fw.common.mithra.list.NulledRelation;
import com.gs.fw.common.mithra.querycache.CachedQuery;
import com.gs.fw.common.mithra.querycache.QueryCache;
import com.gs.fw.common.mithra.portal.*;
import com.gs.fw.common.mithra.remote.*;
import com.gs.fw.common.mithra.transaction.MithraObjectPersister;
import com.gs.fw.common.mithra.util.TimestampPool;
import com.gs.collections.impl.map.mutable.UnifiedMap;
import java.io.Serializable;
/**
* This file was automatically generated using Mithra 16.7.1. Please do not modify it.
* Add custom logic to its subclass instead.
*/
public class RunsMasterQueueFinder
{
private static final SourceAttributeType zSourceAttributeType = SourceAttributeType.create("sourceId",
IntegerAttribute.class);
private static final String IMPL_CLASS_NAME_WITH_SLASHES = "com/gs/fw/common/mithra/notification/RunsMasterQueue";
private static final String BUSINESS_CLASS_NAME_WITH_DOTS = "com.gs.fw.common.mithra.notification.RunsMasterQueue";
private static final FinderMethodMap finderMethodMap;
private static boolean isFullCache;
private static boolean isOffHeap;
private static volatile MithraObjectPortal objectPortal = new UninitializedPortal("com.gs.fw.common.mithra.notification.RunsMasterQueue");
private static final RunsMasterQueueSingleFinder finder = new RunsMasterQueueSingleFinder();
private static ConstantStringSet[] constantStringSets = new ConstantStringSet[0];
private static ConstantIntSet[] constantIntSets = new ConstantIntSet[0];
private static ConstantShortSet[] constantShortSets = new ConstantShortSet[0];
static
{
finderMethodMap = new FinderMethodMap(RunsMasterQueueFinder.RunsMasterQueueRelatedFinder.class);
finderMethodMap.addNormalAttributeName("eventId");
finderMethodMap.addNormalAttributeName("lastUpdateTime");
finderMethodMap.addNormalAttributeName("entity");
finderMethodMap.addNormalAttributeName("lastUpdateUserId");
finderMethodMap.addNormalAttributeName("tranId");
finderMethodMap.addNormalAttributeName("sourceId");
}
public static Attribute[] allPersistentAttributes()
{
return finder.getPersistentAttributes();
}
public static List allRelatedFinders()
{
return finder.getRelationshipFinders();
}
public static List allDependentRelatedFinders()
{
return finder.getDependentRelationshipFinders();
}
public static ConstantStringSet zGetConstantStringSet(int index)
{
return constantStringSets[index];
}
public static ConstantIntSet zGetConstantIntSet(int index)
{
return constantIntSets[index];
}
public static ConstantShortSet zGetConstantShortSet(int index)
{
return constantShortSets[index];
}
public static SourceAttributeType getSourceAttributeType()
{
return RunsMasterQueueFinder.zSourceAttributeType;
}
public static RunsMasterQueue findOne(com.gs.fw.finder.Operation operation)
{
return findOne(operation, false);
}
public static RunsMasterQueue findOneBypassCache(com.gs.fw.finder.Operation operation)
{
return findOne(operation, true);
}
public static RunsMasterQueueList findMany(com.gs.fw.finder.Operation operation)
{
return (RunsMasterQueueList) finder.findMany(operation);
}
public static RunsMasterQueueList findManyBypassCache(com.gs.fw.finder.Operation operation)
{
return (RunsMasterQueueList) finder.findManyBypassCache(operation);
}
private static RunsMasterQueue findOne(com.gs.fw.finder.Operation operation, boolean bypassCache)
{
List found = getMithraObjectPortal().find((Operation) operation, bypassCache);
return (RunsMasterQueue) FinderUtils.findOne(found);
}
public static RunsMasterQueue findByPrimaryKey(int eventId, int sourceId)
{
return finder.findByPrimaryKey(eventId, sourceId);
}
private static final RelationshipHashStrategy forPrimaryKey = new PrimaryKeyRhs();
private static final class PrimaryKeyRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
I3O3L3 _bean = (I3O3L3) _srcData;
RunsMasterQueueData _castedTargetData = (RunsMasterQueueData) _targetData;
if (_bean.getI1AsInteger() == _castedTargetData.getEventId() && _bean.getI2AsInteger() == _castedTargetData.getSourceId())
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
I3O3L3 _bean = (I3O3L3) _srcData;
return HashUtil.combineHashes(HashUtil.hash(_bean.getI1AsInteger()),HashUtil.hash(_bean.getI2AsInteger()));
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
I3O3L3 _bean = (I3O3L3) _srcData;
return HashUtil.combineHashes(HashUtil.hash(_bean.getI1AsInteger()),HashUtil.hash(_bean.getI2AsInteger()));
}
}
public static RunsMasterQueue zFindOneForRelationship(Operation operation)
{
List found = getMithraObjectPortal().findAsCachedQuery(operation, null, false, true, 0).getResult();
return (RunsMasterQueue) FinderUtils.findOne(found);
}
public static MithraObjectPortal getMithraObjectPortal()
{
return objectPortal.getInitializedPortal();
}
public static void clearQueryCache()
{
objectPortal.clearQueryCache();
}
public static void reloadCache()
{
objectPortal.reloadCache();
}
public static class RunsMasterQueueRelatedFinder extends AbstractRelatedFinder
{
private List relationshipFinders;
private List dependentRelationshipFinders;
public static SourceAttributeType zGetSourceAttributeType()
{
return RunsMasterQueueFinder.zSourceAttributeType;
}
private IntegerAttribute eventId;
private TimestampAttribute lastUpdateTime;
private StringAttribute entity;
private StringAttribute lastUpdateUserId;
private ByteArrayAttribute tranId;
private IntegerAttribute sourceId;
public RunsMasterQueueRelatedFinder()
{
super();
}
public RunsMasterQueueRelatedFinder(Mapper mapper)
{
super(mapper);
}
public String getFinderClassName()
{
return "com.gs.fw.common.mithra.notification.RunsMasterQueueFinder";
}
public RelatedFinder getRelationshipFinderByName(String relationshipName)
{
return RunsMasterQueueFinder.finderMethodMap.getRelationshipFinderByName(relationshipName, this);
}
public Attribute getAttributeByName(String attributeName)
{
return RunsMasterQueueFinder.finderMethodMap.getAttributeByName(attributeName, this);
}
public com.gs.collections.api.block.function.Function getAttributeOrRelationshipSelector(String attributeName)
{
return RunsMasterQueueFinder.finderMethodMap.getAttributeOrRelationshipSelectorFunction(attributeName, this);
}
public Attribute[] getPersistentAttributes()
{
Attribute[] attributes = new Attribute[5];
attributes[0] = this.eventId();
attributes[1] = this.lastUpdateTime();
attributes[2] = this.entity();
attributes[3] = this.lastUpdateUserId();
attributes[4] = this.tranId();
return attributes;
}
public List getRelationshipFinders()
{
if (relationshipFinders == null)
{
List relatedFinders = new ArrayList(0);
relationshipFinders = Collections.unmodifiableList(relatedFinders);
}
return relationshipFinders;
}
public List getDependentRelationshipFinders()
{
if (dependentRelationshipFinders == null)
{
List dependentRelatedFinders = new ArrayList(0);
dependentRelationshipFinders = Collections.unmodifiableList(dependentRelatedFinders);
}
return dependentRelationshipFinders;
}
public RunsMasterQueue findOne(com.gs.fw.finder.Operation operation)
{
return RunsMasterQueueFinder.findOne(operation, false);
}
public RunsMasterQueue findOneBypassCache(com.gs.fw.finder.Operation operation)
{
return RunsMasterQueueFinder.findOne(operation, true);
}
public MithraList extends RunsMasterQueue> findMany(com.gs.fw.finder.Operation operation)
{
return new RunsMasterQueueList((Operation) operation);
}
public MithraList extends RunsMasterQueue> findManyBypassCache(com.gs.fw.finder.Operation operation)
{
RunsMasterQueueList result = (RunsMasterQueueList) this.findMany(operation);
result.setBypassCache(true);
return result;
}
public MithraList extends RunsMasterQueue> constructEmptyList()
{
return new RunsMasterQueueList();
}
public int getSerialVersionId()
{
return -2114672526;
}
public boolean isPure()
{
return false;
}
public boolean isTemporary()
{
return false;
}
public int getHierarchyDepth()
{
return 0;
}
public SourceAttributeType getSourceAttributeType()
{
return RunsMasterQueueFinder.zSourceAttributeType;
}
public IntegerAttribute eventId()
{
IntegerAttribute result = this.eventId;
if (result == null)
{
result = mapper == null ? SingleColumnIntegerAttribute.generate("event_seq_no","","eventId",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,false,false,this,null,true,false,false,-1,-1,-1,false, false) :
new MappedIntegerAttribute(RunsMasterQueueFinder.eventId(), this.mapper, this.zGetValueSelector());
this.eventId = result;
}
return result;
}
public TimestampAttribute lastUpdateTime()
{
TimestampAttribute result = this.lastUpdateTime;
if (result == null)
{
result = mapper == null ? SingleColumnTimestampAttribute.generate("last_update_time","","lastUpdateTime",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,true,false,this,null,true,false,-1,-1,-1,TimestampAttribute.CONVERT_NONE,false,false,null, (byte) 0, false) :
new MappedTimestampAttribute(RunsMasterQueueFinder.lastUpdateTime(), this.mapper, this.zGetValueSelector());
this.lastUpdateTime = result;
}
return result;
}
public StringAttribute entity()
{
StringAttribute result = this.entity;
if (result == null)
{
result = mapper == null ? SingleColumnStringAttribute.generate("entity_n","","entity",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,true,false,this,null,true,false,-1,-1,-1,64,true, false) :
new MappedStringAttribute(RunsMasterQueueFinder.entity(), this.mapper, this.zGetValueSelector());
this.entity = result;
}
return result;
}
public StringAttribute lastUpdateUserId()
{
StringAttribute result = this.lastUpdateUserId;
if (result == null)
{
result = mapper == null ? SingleColumnStringAttribute.generate("last_update_userid","","lastUpdateUserId",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,true,false,this,null,true,false,-1,-1,-1,30,true, false) :
new MappedStringAttribute(RunsMasterQueueFinder.lastUpdateUserId(), this.mapper, this.zGetValueSelector());
this.lastUpdateUserId = result;
}
return result;
}
public ByteArrayAttribute tranId()
{
ByteArrayAttribute result = this.tranId;
if (result == null)
{
result = mapper == null ? SingleColumnByteArrayAttribute.generate("rs_origin_xact_id","","tranId",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,true,false,this,null,true,false,-1,-1,-1,120, false) :
new MappedByteArrayAttribute(RunsMasterQueueFinder.tranId(), this.mapper, this.zGetValueSelector());
this.tranId = result;
}
return result;
}
public IntegerAttribute sourceId()
{
IntegerAttribute result = this.sourceId;
if (result == null)
{
result = mapper == null ? SingleColumnIntegerAttribute.generate(null,"","sourceId",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,false,false,this,null,true,false,false,-1,-1,-1,false, false) :
new MappedIntegerAttribute(RunsMasterQueueFinder.sourceId(), this.mapper, this.zGetValueSelector());
this.sourceId = result;
}
return result;
}
public Attribute getSourceAttribute()
{
return RunsMasterQueueFinder.sourceId();
}
private Mapper combineWithMapperIfExists(Mapper newMapper)
{
if (this.mapper != null)
{
return new LinkedMapper(this.mapper, newMapper);
}
return newMapper;
}
public Attribute[] getPrimaryKeyAttributes()
{
return RunsMasterQueueFinder.getPrimaryKeyAttributes();
}
public VersionAttribute getVersionAttribute()
{
return null;
}
public MithraObjectPortal getMithraObjectPortal()
{
return RunsMasterQueueFinder.getMithraObjectPortal();
}
}
public static class RunsMasterQueueCollectionFinder extends RunsMasterQueueRelatedFinder
{
public RunsMasterQueueCollectionFinder(Mapper mapper)
{
super(mapper);
}
}
public static abstract class RunsMasterQueueCollectionFinderForRelatedClasses
extends RunsMasterQueueCollectionFinder
implements DeepRelationshipAttribute
{
public RunsMasterQueueCollectionFinderForRelatedClasses(Mapper mapper)
{
super(mapper);
}
protected NormalAndListValueSelector zGetValueSelector()
{
return this;
}
}
public static class RunsMasterQueueSingleFinder extends RunsMasterQueueRelatedFinder
implements ToOneFinder
{
public RunsMasterQueueSingleFinder(Mapper mapper)
{
super(mapper);
}
public RunsMasterQueueSingleFinder()
{
super(null);
}
public Operation eq(RunsMasterQueue other)
{
return this.eventId().eq(other.getEventId())
.and(this.sourceId().eq(other.getSourceId()))
;
}
// this implementation uses private API. Do NOT copy to application code. Application code must use normal operations for lookups.
public RunsMasterQueue findByPrimaryKey(int eventId, int sourceId)
{
RunsMasterQueue _result = null;
Operation _op = null;
Object _related = null;
{
I3O3L3 _bean = I3O3L3.POOL.getOrConstruct();
_bean.setI1AsInteger(eventId);
_bean.setI2AsInteger(sourceId);
MithraObjectPortal _portal = this.getMithraObjectPortal();
_related = _portal.getAsOneFromCacheForFind(_bean, _bean, forPrimaryKey, null, null);
_bean.release();
}
if (!(_related instanceof NulledRelation)) _result = (RunsMasterQueue) _related;
if (_related == null)
{
_op = this.eventId().eq(eventId).and(this.sourceId().eq(sourceId));
}
if (_op != null)
{
_result = this.findOne(_op);
}
return _result;
}
}
public static abstract class RunsMasterQueueSingleFinderForRelatedClasses extends RunsMasterQueueSingleFinder implements DeepRelationshipAttribute
{
public RunsMasterQueueSingleFinderForRelatedClasses(Mapper mapper)
{
super(mapper);
}
protected NormalAndListValueSelector zGetValueSelector()
{
return this;
}
}
/** maps to ap_UPD_QUEUE.event_seq_no **/
public static IntegerAttribute eventId()
{
return finder.eventId();
}
/** maps to ap_UPD_QUEUE.last_update_time **/
public static TimestampAttribute lastUpdateTime()
{
return finder.lastUpdateTime();
}
/** maps to ap_UPD_QUEUE.entity_n **/
public static StringAttribute entity()
{
return finder.entity();
}
/** maps to ap_UPD_QUEUE.last_update_userid **/
public static StringAttribute lastUpdateUserId()
{
return finder.lastUpdateUserId();
}
/** maps to ap_UPD_QUEUE.rs_origin_xact_id **/
public static ByteArrayAttribute tranId()
{
return finder.tranId();
}
public static IntegerAttribute sourceId()
{
return finder.sourceId();
}
public static Operation eq(RunsMasterQueue other)
{
return finder.eq(other);
}
public static Operation all()
{
return new All(eventId());
}
public static RunsMasterQueueSingleFinder getFinderInstance()
{
return finder;
}
public static Attribute[] getPrimaryKeyAttributes()
{
return new Attribute[]
{
eventId()
, sourceId()
}
;
}
public static Attribute[] getImmutableAttributes()
{
return new Attribute[]
{
eventId()
, eventId()
, sourceId()
}
;
}
public static AsOfAttribute[] getAsOfAttributes()
{
return null;
}
protected static void initializeIndicies(Cache cache)
{
}
protected static void initializePortal(MithraObjectDeserializer objectFactory, Cache cache,
MithraConfigurationManager.Config config)
{
initializeIndicies(cache);
isFullCache = cache.isFullCache();
isOffHeap = cache.isOffHeap();
MithraObjectPortal portal;
if (config.isParticipatingInTx())
{
portal = new MithraTransactionalPortal(objectFactory, cache, getFinderInstance(),
config.getRelationshipCacheSize(), config.getMinQueriesToKeep(), null,
null, null, 0,
(MithraObjectPersister) objectFactory);
}
else
{
portal = new MithraReadOnlyPortal(objectFactory, cache, getFinderInstance(),
config.getRelationshipCacheSize(), config.getMinQueriesToKeep(), null,
null, null, 0,
(MithraObjectPersister) objectFactory);
}
portal.setIndependent(true);
config.initializePortal(portal);
objectPortal.destroy();
objectPortal = portal;
}
protected static void initializeClientPortal(MithraObjectDeserializer objectFactory, Cache cache,
MithraConfigurationManager.Config config)
{
initializeIndicies(cache);
isFullCache = cache.isFullCache();
isOffHeap = cache.isOffHeap();
MithraObjectPortal portal;
if (config.isParticipatingInTx())
{
portal = new MithraTransactionalPortal(objectFactory, cache, getFinderInstance(),
config.getRelationshipCacheSize(), config.getMinQueriesToKeep(),
null, null,
null, 0,
new RemoteMithraObjectPersister(config.getRemoteMithraService(), getFinderInstance(), false));
}
else
{
portal = new MithraReadOnlyPortal(objectFactory, cache, getFinderInstance(),
config.getRelationshipCacheSize(), config.getMinQueriesToKeep(),
null, null,
null, 0,
new RemoteMithraObjectPersister(config.getRemoteMithraService(), getFinderInstance(), false));
}
portal.setIndependent(true);
config.initializePortal(portal);
objectPortal.destroy();
objectPortal = portal;
}
public static boolean isFullCache()
{
return isFullCache;
}
public static boolean isOffHeap()
{
return isOffHeap;
}
public static Attribute getAttributeByName(String attributeName)
{
return finder.getAttributeByName(attributeName);
}
public static com.gs.collections.api.block.function.Function getAttributeOrRelationshipSelector(String attributeName)
{
return finder.getAttributeOrRelationshipSelector(attributeName);
}
public static RelatedFinder getRelatedFinderByName(String relationshipName)
{
return finder.getRelationshipFinderByName(relationshipName);
}
public static DoubleAttribute[] zGetDoubleAttributes()
{
DoubleAttribute[] result = new DoubleAttribute[0];
return result;
}
public static BigDecimalAttribute[] zGetBigDecimalAttributes()
{
BigDecimalAttribute[] result = new BigDecimalAttribute[0];
return result;
}
public static void zResetPortal()
{
objectPortal.destroy();
objectPortal = new UninitializedPortal("com.gs.fw.common.mithra.notification.RunsMasterQueue");
isFullCache = false;
isOffHeap = false;
}
public static void setTransactionModeFullTransactionParticipation(MithraTransaction tx)
{
tx.setTxParticipationMode(objectPortal, FullTransactionalParticipationMode.getInstance());
}
public static void setTransactionModeReadCacheUpdateCausesRefreshAndLock(MithraTransaction tx)
{
tx.setTxParticipationMode(objectPortal, ReadCacheUpdateCausesRefreshAndLockTxParticipationMode.getInstance());
}
public static void registerForNotification(int sourceId, MithraApplicationClassLevelNotificationListener listener)
{
Set sourceValueSet = new HashSet();
sourceValueSet.add(sourceId);
getMithraObjectPortal().registerForApplicationClassLevelNotification(sourceValueSet, listener);
}
public static void registerForNotification(Set sourceIdSet, MithraApplicationClassLevelNotificationListener listener)
{
getMithraObjectPortal().registerForApplicationClassLevelNotification(sourceIdSet, listener);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy