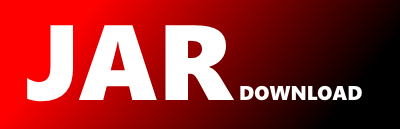
com.gs.collections.api.map.OrderedMap Maven / Gradle / Ivy
/*
* Copyright 2015 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.api.map;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.function.Function0;
import com.gs.collections.api.block.function.Function2;
import com.gs.collections.api.block.function.primitive.BooleanFunction;
import com.gs.collections.api.block.function.primitive.ByteFunction;
import com.gs.collections.api.block.function.primitive.CharFunction;
import com.gs.collections.api.block.function.primitive.DoubleFunction;
import com.gs.collections.api.block.function.primitive.FloatFunction;
import com.gs.collections.api.block.function.primitive.IntFunction;
import com.gs.collections.api.block.function.primitive.LongFunction;
import com.gs.collections.api.block.function.primitive.ShortFunction;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.api.block.procedure.Procedure2;
import com.gs.collections.api.list.ListIterable;
import com.gs.collections.api.list.primitive.BooleanList;
import com.gs.collections.api.list.primitive.ByteList;
import com.gs.collections.api.list.primitive.CharList;
import com.gs.collections.api.list.primitive.DoubleList;
import com.gs.collections.api.list.primitive.FloatList;
import com.gs.collections.api.list.primitive.IntList;
import com.gs.collections.api.list.primitive.LongList;
import com.gs.collections.api.list.primitive.ShortList;
import com.gs.collections.api.multimap.list.ListMultimap;
import com.gs.collections.api.ordered.ReversibleIterable;
import com.gs.collections.api.partition.list.PartitionList;
import com.gs.collections.api.tuple.Pair;
/**
* A map whose keys are ordered but not necessarily sorted, for example a linked hash map.
*/
public interface OrderedMap
extends MapIterable, ReversibleIterable
{
OrderedMap tap(Procedure super V> procedure);
OrderedMap flipUniqueValues();
ListMultimap flip();
OrderedMap select(Predicate2 super K, ? super V> predicate);
OrderedMap reject(Predicate2 super K, ? super V> predicate);
OrderedMap collect(Function2 super K, ? super V, Pair> function);
OrderedMap collectValues(Function2 super K, ? super V, ? extends R> function);
ImmutableOrderedMap toImmutable();
OrderedMap toReversed();
OrderedMap take(int count);
OrderedMap takeWhile(Predicate super V> predicate);
OrderedMap drop(int count);
OrderedMap dropWhile(Predicate super V> predicate);
PartitionList partitionWhile(Predicate super V> predicate);
ListIterable distinct();
ListIterable select(Predicate super V> predicate);
ListIterable selectWith(Predicate2 super V, ? super P> predicate, P parameter);
ListIterable reject(Predicate super V> predicate);
ListIterable rejectWith(Predicate2 super V, ? super P> predicate, P parameter);
PartitionList partition(Predicate super V> predicate);
PartitionList partitionWith(Predicate2 super V, ? super P> predicate, P parameter);
BooleanList collectBoolean(BooleanFunction super V> booleanFunction);
ByteList collectByte(ByteFunction super V> byteFunction);
CharList collectChar(CharFunction super V> charFunction);
DoubleList collectDouble(DoubleFunction super V> doubleFunction);
FloatList collectFloat(FloatFunction super V> floatFunction);
IntList collectInt(IntFunction super V> intFunction);
LongList collectLong(LongFunction super V> longFunction);
ShortList collectShort(ShortFunction super V> shortFunction);
ListIterable> zip(Iterable that);
ListIterable> zipWithIndex();
ListIterable collectWith(Function2 super V, ? super P, ? extends V1> function, P parameter);
ListIterable collectIf(Predicate super V> predicate, Function super V, ? extends V1> function);
ListIterable selectInstancesOf(Class clazz);
ListIterable flatCollect(Function super V, ? extends Iterable> function);
ListMultimap groupBy(Function super V, ? extends V1> function);
ListMultimap groupByEach(Function super V, ? extends Iterable> function);
OrderedMap groupByUniqueKey(Function super V, ? extends V1> function);
OrderedMap aggregateInPlaceBy(Function super V, ? extends KK> groupBy, Function0 extends VV> zeroValueFactory, Procedure2 super VV, ? super V> mutatingAggregator);
OrderedMap aggregateBy(Function super V, ? extends KK> groupBy, Function0 extends VV> zeroValueFactory, Function2 super VV, ? super V, ? extends VV> nonMutatingAggregator);
}