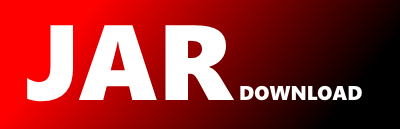
com.gs.collections.impl.bag.mutable.SynchronizedBag Maven / Gradle / Ivy
Show all versions of gs-collections Show documentation
/*
* Copyright 2013 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.bag.mutable;
import java.io.Serializable;
import java.util.Collection;
import java.util.Collections;
import com.gs.collections.api.bag.ImmutableBag;
import com.gs.collections.api.bag.MutableBag;
import com.gs.collections.api.bag.primitive.MutableBooleanBag;
import com.gs.collections.api.bag.primitive.MutableByteBag;
import com.gs.collections.api.bag.primitive.MutableCharBag;
import com.gs.collections.api.bag.primitive.MutableDoubleBag;
import com.gs.collections.api.bag.primitive.MutableFloatBag;
import com.gs.collections.api.bag.primitive.MutableIntBag;
import com.gs.collections.api.bag.primitive.MutableLongBag;
import com.gs.collections.api.bag.primitive.MutableShortBag;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.function.Function2;
import com.gs.collections.api.block.function.primitive.BooleanFunction;
import com.gs.collections.api.block.function.primitive.ByteFunction;
import com.gs.collections.api.block.function.primitive.CharFunction;
import com.gs.collections.api.block.function.primitive.DoubleFunction;
import com.gs.collections.api.block.function.primitive.FloatFunction;
import com.gs.collections.api.block.function.primitive.IntFunction;
import com.gs.collections.api.block.function.primitive.LongFunction;
import com.gs.collections.api.block.function.primitive.ShortFunction;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.block.predicate.primitive.IntPredicate;
import com.gs.collections.api.block.procedure.primitive.ObjectIntProcedure;
import com.gs.collections.api.map.MapIterable;
import com.gs.collections.api.multimap.bag.MutableBagMultimap;
import com.gs.collections.api.partition.bag.PartitionMutableBag;
import com.gs.collections.api.set.MutableSet;
import com.gs.collections.api.tuple.Pair;
import com.gs.collections.impl.collection.mutable.AbstractSynchronizedMutableCollection;
import com.gs.collections.impl.collection.mutable.SynchronizedCollectionSerializationProxy;
import com.gs.collections.impl.factory.Bags;
import net.jcip.annotations.GuardedBy;
/**
* A synchronized view of a {@link MutableBag}. It is imperative that the user manually synchronize on the collection when iterating over it using the
* standard JDK iterator or JDK 5 for loop, as per {@link Collections#synchronizedCollection(Collection)}.
*
* @see MutableBag#asSynchronized()
* @since 1.0
*/
public class SynchronizedBag
extends AbstractSynchronizedMutableCollection
implements MutableBag, Serializable
{
SynchronizedBag(MutableBag bag)
{
super(bag);
}
public SynchronizedBag(MutableBag bag, Object newLock)
{
super(bag, newLock);
}
/**
* This method will take a MutableBag and wrap it directly in a SynchronizedBag.
*/
public static > SynchronizedBag of(B bag)
{
return new SynchronizedBag(bag);
}
@GuardedBy("getLock()")
private MutableBag getMutableBag()
{
return (MutableBag) this.getCollection();
}
@Override
public MutableBag asUnmodifiable()
{
synchronized (this.getLock())
{
return UnmodifiableBag.of(this);
}
}
@Override
public ImmutableBag toImmutable()
{
return Bags.immutable.ofAll(this);
}
@Override
public MutableBag asSynchronized()
{
return this;
}
@Override
public MutableBag collect(Function super T, ? extends V> function)
{
synchronized (this.getLock())
{
return this.getMutableBag().collect(function);
}
}
@Override
public MutableBooleanBag collectBoolean(BooleanFunction super T> booleanFunction)
{
synchronized (this.getLock())
{
return this.getMutableBag().collectBoolean(booleanFunction);
}
}
@Override
public MutableByteBag collectByte(ByteFunction super T> byteFunction)
{
synchronized (this.getLock())
{
return this.getMutableBag().collectByte(byteFunction);
}
}
@Override
public MutableCharBag collectChar(CharFunction super T> charFunction)
{
synchronized (this.getLock())
{
return this.getMutableBag().collectChar(charFunction);
}
}
@Override
public MutableDoubleBag collectDouble(DoubleFunction super T> doubleFunction)
{
synchronized (this.getLock())
{
return this.getMutableBag().collectDouble(doubleFunction);
}
}
@Override
public MutableFloatBag collectFloat(FloatFunction super T> floatFunction)
{
synchronized (this.getLock())
{
return this.getMutableBag().collectFloat(floatFunction);
}
}
@Override
public MutableIntBag collectInt(IntFunction super T> function)
{
synchronized (this.getLock())
{
return this.getMutableBag().collectInt(function);
}
}
@Override
public MutableLongBag collectLong(LongFunction super T> longFunction)
{
synchronized (this.getLock())
{
return this.getMutableBag().collectLong(longFunction);
}
}
@Override
public MutableShortBag collectShort(ShortFunction super T> shortFunction)
{
synchronized (this.getLock())
{
return this.getMutableBag().collectShort(shortFunction);
}
}
@Override
public MutableBag flatCollect(Function super T, ? extends Iterable> function)
{
synchronized (this.getLock())
{
return this.getMutableBag().flatCollect(function);
}
}
@Override
public MutableBag collectIf(
Predicate super T> predicate,
Function super T, ? extends V> function)
{
synchronized (this.getLock())
{
return this.getMutableBag().collectIf(predicate, function);
}
}
@Override
public MutableBag collectWith(Function2 super T, ? super P, ? extends V> function, P parameter)
{
synchronized (this.getLock())
{
return this.getMutableBag().collectWith(function, parameter);
}
}
@Override
public MutableBagMultimap groupBy(Function super T, ? extends V> function)
{
synchronized (this.getLock())
{
return this.getMutableBag().groupBy(function);
}
}
@Override
public MutableBagMultimap groupByEach(Function super T, ? extends Iterable> function)
{
synchronized (this.getLock())
{
return this.getMutableBag().groupByEach(function);
}
}
@Override
public MutableBag newEmpty()
{
synchronized (this.getLock())
{
return this.getMutableBag().newEmpty();
}
}
@Override
public MutableBag reject(Predicate super T> predicate)
{
synchronized (this.getLock())
{
return this.getMutableBag().reject(predicate);
}
}
@Override
public MutableBag rejectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.getLock())
{
return this.getMutableBag().rejectWith(predicate, parameter);
}
}
public MutableBag selectByOccurrences(IntPredicate predicate)
{
synchronized (this.getLock())
{
return this.getMutableBag().selectByOccurrences(predicate);
}
}
@Override
public MutableBag select(Predicate super T> predicate)
{
synchronized (this.getLock())
{
return this.getMutableBag().select(predicate);
}
}
@Override
public MutableBag selectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.getLock())
{
return this.getMutableBag().selectWith(predicate, parameter);
}
}
@Override
public PartitionMutableBag partition(Predicate super T> predicate)
{
synchronized (this.getLock())
{
return this.getMutableBag().partition(predicate);
}
}
@Override
public PartitionMutableBag partitionWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.getLock())
{
return this.getMutableBag().partitionWith(predicate, parameter);
}
}
@Override
public MutableBag selectInstancesOf(Class clazz)
{
synchronized (this.getLock())
{
return this.getMutableBag().selectInstancesOf(clazz);
}
}
@Override
public boolean equals(Object obj)
{
synchronized (this.getLock())
{
return this.getMutableBag().equals(obj);
}
}
@Override
public int hashCode()
{
synchronized (this.getLock())
{
return this.getMutableBag().hashCode();
}
}
public void addOccurrences(T item, int occurrences)
{
synchronized (this.getLock())
{
this.getMutableBag().addOccurrences(item, occurrences);
}
}
public boolean removeOccurrences(Object item, int occurrences)
{
synchronized (this.getLock())
{
return this.getMutableBag().removeOccurrences(item, occurrences);
}
}
public boolean setOccurrences(T item, int occurrences)
{
synchronized (this.getLock())
{
return this.getMutableBag().setOccurrences(item, occurrences);
}
}
public int sizeDistinct()
{
synchronized (this.getLock())
{
return this.getMutableBag().sizeDistinct();
}
}
public int occurrencesOf(Object item)
{
synchronized (this.getLock())
{
return this.getMutableBag().occurrencesOf(item);
}
}
public void forEachWithOccurrences(ObjectIntProcedure super T> objectIntProcedure)
{
synchronized (this.getLock())
{
this.getMutableBag().forEachWithOccurrences(objectIntProcedure);
}
}
public MapIterable toMapOfItemToCount()
{
synchronized (this.getLock())
{
return this.getMutableBag().toMapOfItemToCount();
}
}
@Override
public MutableBag> zip(Iterable that)
{
synchronized (this.getLock())
{
return this.getMutableBag().zip(that);
}
}
@Override
public >> R zip(Iterable that, R target)
{
synchronized (this.getLock())
{
return this.getMutableBag().zip(that, target);
}
}
@Override
public MutableSet> zipWithIndex()
{
synchronized (this.getLock())
{
return this.getMutableBag().zipWithIndex();
}
}
public String toStringOfItemToCount()
{
synchronized (this.getLock())
{
return this.getMutableBag().toStringOfItemToCount();
}
}
@Override
public >> R zipWithIndex(R target)
{
synchronized (this.getLock())
{
return this.getMutableBag().zipWithIndex(target);
}
}
@Override
public MutableBag with(T element)
{
this.add(element);
return this;
}
@Override
public MutableBag without(T element)
{
this.remove(element);
return this;
}
@Override
public MutableBag withAll(Iterable extends T> elements)
{
this.addAllIterable(elements);
return this;
}
@Override
public MutableBag withoutAll(Iterable extends T> elements)
{
this.removeAllIterable(elements);
return this;
}
protected Object writeReplace()
{
return new SynchronizedCollectionSerializationProxy(this.getMutableBag());
}
}