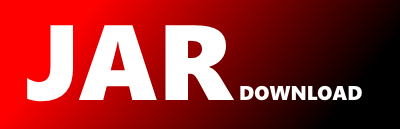
com.gs.collections.impl.list.fixed.AbstractArrayAdapter Maven / Gradle / Ivy
Show all versions of gs-collections Show documentation
/*
* Copyright 2014 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.list.fixed;
import java.io.IOException;
import java.lang.reflect.Array;
import java.util.Arrays;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
import java.util.RandomAccess;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.function.Function0;
import com.gs.collections.api.block.function.Function2;
import com.gs.collections.api.block.function.Function3;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.api.block.procedure.Procedure2;
import com.gs.collections.api.block.procedure.primitive.ObjectIntProcedure;
import com.gs.collections.api.list.MutableList;
import com.gs.collections.api.tuple.Twin;
import com.gs.collections.impl.block.factory.Comparators;
import com.gs.collections.impl.block.factory.Predicates;
import com.gs.collections.impl.list.mutable.AbstractMutableList;
import com.gs.collections.impl.list.mutable.FastList;
import com.gs.collections.impl.list.mutable.ListAdapter;
import com.gs.collections.impl.utility.ArrayIterate;
import com.gs.collections.impl.utility.Iterate;
import com.gs.collections.impl.utility.internal.IterableIterate;
public abstract class AbstractArrayAdapter
extends AbstractMutableList
implements RandomAccess
{
protected T[] items;
/**
* This method must be here so subclasses can be serializable.
*/
protected AbstractArrayAdapter()
{
}
protected AbstractArrayAdapter(T[] newElements)
{
this.items = newElements;
}
@Override
public boolean notEmpty()
{
return ArrayIterate.notEmpty(this.items);
}
@Override
public T getFirst()
{
return ArrayIterate.getFirst(this.items);
}
@Override
public T getLast()
{
return ArrayIterate.getLast(this.items);
}
@Override
public void forEach(Procedure super T> procedure)
{
int size = this.size();
for (int i = 0; i < size; i++)
{
procedure.value(this.items[i]);
}
}
@Override
public void forEachWithIndex(ObjectIntProcedure super T> objectIntProcedure)
{
int size = this.items.length;
for (int i = 0; i < size; i++)
{
objectIntProcedure.value(this.items[i], i);
}
}
@Override
public void forEachWithIndex(int fromIndex, int toIndex, ObjectIntProcedure super T> objectIntProcedure)
{
ArrayIterate.forEachWithIndex(this.items, fromIndex, toIndex, objectIntProcedure);
}
@Override
public void removeIf(Predicate super T> predicate)
{
throw new UnsupportedOperationException("Cannot call removeIf() on " + this.getClass().getSimpleName());
}
@Override
public void removeIfWith(Predicate2 super T, ? super P> predicate, P parameter)
{
throw new UnsupportedOperationException("Cannot call removeIfWith() on " + this.getClass().getSimpleName());
}
@Override
public T detect(Predicate super T> predicate)
{
return ArrayIterate.detect(this.items, predicate);
}
@Override
public T detectIfNone(Predicate super T> predicate, Function0 extends T> function)
{
T result = this.detect(predicate);
return result == null ? function.value() : result;
}
@Override
public int count(Predicate super T> predicate)
{
return ArrayIterate.count(this.items, predicate);
}
@Override
public boolean anySatisfy(Predicate super T> predicate)
{
return ArrayIterate.anySatisfy(this.items, predicate);
}
@Override
public boolean allSatisfy(Predicate super T> predicate)
{
return ArrayIterate.allSatisfy(this.items, predicate);
}
@Override
public boolean noneSatisfy(Predicate super T> predicate)
{
return ArrayIterate.noneSatisfy(this.items, predicate);
}
@Override
public IV injectInto(IV injectedValue, Function2 super IV, ? super T, ? extends IV> function)
{
return ArrayIterate.injectInto(injectedValue, this.items, function);
}
@Override
public MutableList select(Predicate super T> predicate)
{
return this.select(predicate, FastList.newList());
}
@Override
public > R select(Predicate super T> predicate, R target)
{
return ArrayIterate.select(this.items, predicate, target);
}
@Override
public MutableList reject(Predicate super T> predicate)
{
return this.reject(predicate, FastList.newList());
}
@Override
public > R reject(Predicate super T> predicate, R target)
{
return ArrayIterate.reject(this.items, predicate, target);
}
@Override
public MutableList collect(Function super T, ? extends V> function)
{
return this.collect(function, FastList.newList(this.size()));
}
@Override
public > R collect(Function super T, ? extends V> function, R target)
{
return ArrayIterate.collect(this.items, function, target);
}
@Override
public MutableList collectIf(
Predicate super T> predicate,
Function super T, ? extends V> function)
{
return this.collectIf(predicate, function, FastList.newList());
}
@Override
public > R collectIf(
Predicate super T> predicate, Function super T, ? extends V> function, R target)
{
return ArrayIterate.collectIf(this.items, predicate, function, target);
}
@Override
public MutableList flatCollect(Function super T, ? extends Iterable> function)
{
return ArrayIterate.flatCollect(this.items, function);
}
@Override
public > R flatCollect(Function super T, ? extends Iterable> function, R target)
{
return ArrayIterate.flatCollect(this.items, function, target);
}
@Override
public Twin> selectAndRejectWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
return ArrayIterate.selectAndRejectWith(this.items, predicate, parameter);
}
public int size()
{
return this.items.length;
}
@Override
public boolean isEmpty()
{
return ArrayIterate.isEmpty(this.items);
}
@Override
public boolean contains(Object o)
{
return this.anySatisfy(Predicates.equal(o));
}
@Override
public Iterator iterator()
{
return Arrays.asList(this.items).iterator();
}
@Override
public Object[] toArray()
{
return this.items.clone();
}
@Override
public E[] toArray(E[] array)
{
int size = this.size();
E[] result = array.length < size
? (E[]) Array.newInstance(array.getClass().getComponentType(), size)
: array;
System.arraycopy(this.items, 0, result, 0, size);
if (result.length > size)
{
result[size] = null;
}
return result;
}
@Override
public boolean add(T item)
{
throw new UnsupportedOperationException("Cannot call add() on " + this.getClass().getSimpleName());
}
@Override
public boolean remove(Object o)
{
throw new UnsupportedOperationException("Cannot call remove() on " + this.getClass().getSimpleName());
}
@Override
public boolean containsAll(Collection> collection)
{
return Iterate.allSatisfy(collection, Predicates.in(this.items));
}
@Override
public boolean addAll(Collection extends T> collection)
{
throw new UnsupportedOperationException("Cannot call addAll() on " + this.getClass().getSimpleName());
}
@Override
public boolean addAllIterable(Iterable extends T> iterable)
{
throw new UnsupportedOperationException("Cannot call addAllIterable() on " + this.getClass().getSimpleName());
}
@Override
public boolean removeAll(Collection> collection)
{
throw new UnsupportedOperationException("Cannot call removeAll() on " + this.getClass().getSimpleName());
}
@Override
public boolean removeAllIterable(Iterable> iterable)
{
throw new UnsupportedOperationException("Cannot call removeAllIterable() on " + this.getClass().getSimpleName());
}
@Override
public boolean retainAll(Collection> collection)
{
throw new UnsupportedOperationException("Cannot call retainAll() on " + this.getClass().getSimpleName());
}
@Override
public boolean retainAllIterable(Iterable> iterable)
{
throw new UnsupportedOperationException("Cannot call retainAllIterable() on " + this.getClass().getSimpleName());
}
public void clear()
{
throw new UnsupportedOperationException("Cannot call clear() on " + this.getClass().getSimpleName());
}
public boolean addAll(int index, Collection extends T> collection)
{
throw new UnsupportedOperationException("Cannot call addAll() on " + this.getClass().getSimpleName());
}
public T get(int index)
{
if (index >= this.size())
{
throw new IndexOutOfBoundsException("Index: " + index + ", Size: " + this.size());
}
return this.items[index];
}
public void add(int index, T element)
{
throw new UnsupportedOperationException("Cannot call add() on " + this.getClass().getSimpleName());
}
public T remove(int index)
{
throw new UnsupportedOperationException("Cannot call remove() on " + this.getClass().getSimpleName());
}
@Override
public int indexOf(Object item)
{
return ArrayIterate.indexOf(this.items, item);
}
@Override
public int lastIndexOf(Object item)
{
return Arrays.asList(this.items).lastIndexOf(item);
}
@Override
public ListIterator listIterator(int index)
{
return Arrays.asList(this.items).listIterator(index);
}
@Override
public MutableList subList(int fromIndex, int toIndex)
{
return ListAdapter.adapt(Arrays.asList(this.items).subList(fromIndex, toIndex));
}
@Override
public boolean equals(Object otherList)
{
if (otherList == this)
{
return true;
}
if (!(otherList instanceof List))
{
return false;
}
List> list = (List>) otherList;
if (otherList instanceof AbstractArrayAdapter)
{
return this.abstractArrayAdapterEquals((AbstractArrayAdapter>) otherList);
}
if (otherList instanceof RandomAccess)
{
return this.randomAccessListEquals(list);
}
return this.regularListEquals(list);
}
public boolean abstractArrayAdapterEquals(AbstractArrayAdapter> otherList)
{
return Arrays.equals(this.items, otherList.items);
}
private boolean regularListEquals(List> otherList)
{
Iterator> iterator = otherList.iterator();
for (int i = 0; i < this.size(); i++)
{
T one = this.items[i];
if (!iterator.hasNext())
{
return false;
}
Object two = iterator.next();
if (!Comparators.nullSafeEquals(one, two))
{
return false;
}
}
return !iterator.hasNext();
}
private boolean randomAccessListEquals(List> otherList)
{
if (this.size() != otherList.size())
{
return false;
}
int n = this.items.length;
for (int i = 0; i < n; i++)
{
Object one = this.items[i];
Object two = otherList.get(i);
if (!Comparators.nullSafeEquals(one, two))
{
return false;
}
}
return true;
}
@Override
public int hashCode()
{
return Arrays.hashCode(this.items);
}
@Override
public void forEachWith(Procedure2 super T, ? super P> procedure, P parameter)
{
ArrayIterate.forEachWith(this.items, procedure, parameter);
}
@Override
public
MutableList selectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return this.selectWith(predicate, parameter, FastList.newList());
}
@Override
public > R selectWith(
Predicate2 super T, ? super P> predicate,
P parameter,
R targetCollection)
{
return ArrayIterate.selectWith(this.items, predicate, parameter, targetCollection);
}
@Override
public
MutableList rejectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return this.rejectWith(predicate, parameter, FastList.newList());
}
@Override
public > R rejectWith(
Predicate2 super T, ? super P> predicate,
P parameter,
R targetCollection)
{
return ArrayIterate.rejectWith(this.items, predicate, parameter, targetCollection);
}
@Override
public
MutableList collectWith(Function2 super T, ? super P, ? extends A> function, P parameter)
{
return this.collectWith(function, parameter, FastList.newList());
}
@Override
public > R collectWith(
Function2 super T, ? super P, ? extends A> function, P parameter, R targetCollection)
{
return ArrayIterate.collectWith(this.items, function, parameter, targetCollection);
}
@Override
public IV injectIntoWith(
IV injectValue, Function3 super IV, ? super T, ? super P, ? extends IV> function, P parameter)
{
return ArrayIterate.injectIntoWith(injectValue, this.items, function, parameter);
}
@Override
public void forEach(int fromIndex, int toIndex, Procedure super T> procedure)
{
ArrayIterate.forEach(this.items, fromIndex, toIndex, procedure);
}
@Override
public T detectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return ArrayIterate.detectWith(this.items, predicate, parameter);
}
@Override
public
T detectWithIfNone(
Predicate2 super T, ? super P> predicate,
P parameter,
Function0 extends T> function)
{
T result = this.detectWith(predicate, parameter);
return result == null ? function.value() : result;
}
@Override
public
int countWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return ArrayIterate.countWith(this.items, predicate, parameter);
}
@Override
public
boolean anySatisfyWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return ArrayIterate.anySatisfyWith(this.items, predicate, parameter);
}
@Override
public
boolean allSatisfyWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return ArrayIterate.allSatisfyWith(this.items, predicate, parameter);
}
@Override
public
boolean noneSatisfyWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return ArrayIterate.noneSatisfyWith(this.items, predicate, parameter);
}
@Override
public MutableList distinct()
{
return ArrayIterate.distinct(this.items, FastList.newList());
}
@Override
public void appendString(Appendable appendable, String start, String separator, String end)
{
try
{
appendable.append(start);
if (this.notEmpty())
{
appendable.append(IterableIterate.stringValueOfItem(this, this.items[0]));
int size = this.size();
for (int i = 1; i < size; i++)
{
appendable.append(separator);
appendable.append(IterableIterate.stringValueOfItem(this, this.items[i]));
}
}
appendable.append(end);
}
catch (IOException e)
{
throw new RuntimeException(e);
}
}
}