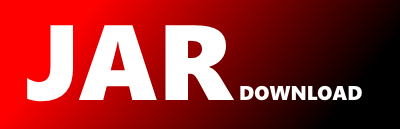
com.gs.collections.impl.list.mutable.MultiReaderFastList Maven / Gradle / Ivy
Show all versions of gs-collections Show documentation
/*
* Copyright 2014 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.list.mutable;
import java.io.Externalizable;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
import java.util.RandomAccess;
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
import com.gs.collections.api.LazyIterable;
import com.gs.collections.api.RichIterable;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.function.Function2;
import com.gs.collections.api.block.function.primitive.BooleanFunction;
import com.gs.collections.api.block.function.primitive.ByteFunction;
import com.gs.collections.api.block.function.primitive.CharFunction;
import com.gs.collections.api.block.function.primitive.DoubleFunction;
import com.gs.collections.api.block.function.primitive.FloatFunction;
import com.gs.collections.api.block.function.primitive.IntFunction;
import com.gs.collections.api.block.function.primitive.LongFunction;
import com.gs.collections.api.block.function.primitive.ShortFunction;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.api.block.procedure.primitive.ObjectIntProcedure;
import com.gs.collections.api.collection.primitive.MutableBooleanCollection;
import com.gs.collections.api.collection.primitive.MutableByteCollection;
import com.gs.collections.api.collection.primitive.MutableCharCollection;
import com.gs.collections.api.collection.primitive.MutableDoubleCollection;
import com.gs.collections.api.collection.primitive.MutableFloatCollection;
import com.gs.collections.api.collection.primitive.MutableIntCollection;
import com.gs.collections.api.collection.primitive.MutableLongCollection;
import com.gs.collections.api.collection.primitive.MutableShortCollection;
import com.gs.collections.api.list.ImmutableList;
import com.gs.collections.api.list.MutableList;
import com.gs.collections.api.list.primitive.MutableBooleanList;
import com.gs.collections.api.list.primitive.MutableByteList;
import com.gs.collections.api.list.primitive.MutableCharList;
import com.gs.collections.api.list.primitive.MutableDoubleList;
import com.gs.collections.api.list.primitive.MutableFloatList;
import com.gs.collections.api.list.primitive.MutableIntList;
import com.gs.collections.api.list.primitive.MutableLongList;
import com.gs.collections.api.list.primitive.MutableShortList;
import com.gs.collections.api.map.MutableMap;
import com.gs.collections.api.multimap.list.MutableListMultimap;
import com.gs.collections.api.partition.list.PartitionMutableList;
import com.gs.collections.api.stack.MutableStack;
import com.gs.collections.api.tuple.Pair;
import com.gs.collections.impl.collection.mutable.AbstractMultiReaderMutableCollection;
import com.gs.collections.impl.factory.Lists;
import com.gs.collections.impl.lazy.ReverseIterable;
import com.gs.collections.impl.stack.mutable.ArrayStack;
import com.gs.collections.impl.utility.LazyIterate;
import static com.gs.collections.impl.factory.Iterables.*;
/**
* MultiReadFastList provides a thread-safe wrapper around a FastList, using a ReentrantReadWriteLock. In order to
* provide true thread-safety, MultiReaderFastList does not implement iterator(), listIterator(), listIterator(int), or
* get(int), as all of these methods require an external lock to be taken to provide thread-safe iteration. All of
* these methods are available however, if you use the withReadLockAndDelegate() or withWriteLockAndDelegate() methods.
* Both of these methods take a parameter of type Procedure, and a wrapped version of the underlying
* FastList is returned. This wrapper guarantees that no external pointer can ever reference the underlying FastList
* outside of a locked procedure. In the case of the read lock method, an Unmodifiable version of the collection is
* offered, which will throw UnsupportedOperationExceptions on any write methods like add or remove.
*/
public final class MultiReaderFastList
extends AbstractMultiReaderMutableCollection
implements RandomAccess, Externalizable, MutableList
{
private static final long serialVersionUID = 1L;
private transient ReadWriteLock lock;
private MutableList delegate;
@SuppressWarnings("UnusedDeclaration")
public MultiReaderFastList()
{
// For Externalizable use only
}
private MultiReaderFastList(MutableList newDelegate)
{
this(newDelegate, new ReentrantReadWriteLock());
}
private MultiReaderFastList(MutableList newDelegate, ReadWriteLock newLock)
{
this.lock = newLock;
this.delegate = newDelegate;
}
public static MultiReaderFastList newList()
{
return new MultiReaderFastList(FastList.newList());
}
public static MultiReaderFastList newList(int capacity)
{
return new MultiReaderFastList(FastList.newList(capacity));
}
public static MultiReaderFastList newList(Iterable iterable)
{
return new MultiReaderFastList(FastList.newList(iterable));
}
public static MultiReaderFastList newListWith(T... elements)
{
return new MultiReaderFastList(FastList.newListWith(elements));
}
@Override
protected MutableList getDelegate()
{
return this.delegate;
}
@Override
protected ReadWriteLock getLock()
{
return this.lock;
}
UntouchableMutableList asReadUntouchable()
{
return new UntouchableMutableList(this.delegate.asUnmodifiable());
}
UntouchableMutableList asWriteUntouchable()
{
return new UntouchableMutableList(this.delegate);
}
public void withReadLockAndDelegate(Procedure> procedure)
{
this.acquireReadLock();
try
{
UntouchableMutableList list = this.asReadUntouchable();
procedure.value(list);
list.becomeUseless();
}
finally
{
this.unlockReadLock();
}
}
public void withWriteLockAndDelegate(Procedure> procedure)
{
this.acquireWriteLock();
try
{
UntouchableMutableList list = this.asWriteUntouchable();
procedure.value(list);
list.becomeUseless();
}
finally
{
this.unlockWriteLock();
}
}
public MutableList asSynchronized()
{
this.acquireReadLock();
try
{
return SynchronizedMutableList.of(this);
}
finally
{
this.unlockReadLock();
}
}
public MutableList asUnmodifiable()
{
this.acquireReadLock();
try
{
return UnmodifiableMutableList.of(this);
}
finally
{
this.unlockReadLock();
}
}
public ImmutableList toImmutable()
{
this.acquireReadLock();
try
{
return Lists.immutable.ofAll(this.delegate);
}
finally
{
this.unlockReadLock();
}
}
@Override
public MutableList clone()
{
this.acquireReadLock();
try
{
return new MultiReaderFastList(this.delegate.clone());
}
finally
{
this.unlockReadLock();
}
}
public MutableList collect(Function super T, ? extends V> function)
{
this.acquireReadLock();
try
{
return this.delegate.collect(function);
}
finally
{
this.unlockReadLock();
}
}
public MutableBooleanList collectBoolean(BooleanFunction super T> booleanFunction)
{
this.acquireReadLock();
try
{
return this.delegate.collectBoolean(booleanFunction);
}
finally
{
this.unlockReadLock();
}
}
public MutableByteList collectByte(ByteFunction super T> byteFunction)
{
this.acquireReadLock();
try
{
return this.delegate.collectByte(byteFunction);
}
finally
{
this.unlockReadLock();
}
}
public MutableCharList collectChar(CharFunction super T> charFunction)
{
this.acquireReadLock();
try
{
return this.delegate.collectChar(charFunction);
}
finally
{
this.unlockReadLock();
}
}
public MutableDoubleList collectDouble(DoubleFunction super T> doubleFunction)
{
this.acquireReadLock();
try
{
return this.delegate.collectDouble(doubleFunction);
}
finally
{
this.unlockReadLock();
}
}
public MutableFloatList collectFloat(FloatFunction super T> floatFunction)
{
this.acquireReadLock();
try
{
return this.delegate.collectFloat(floatFunction);
}
finally
{
this.unlockReadLock();
}
}
public MutableIntList collectInt(IntFunction super T> intFunction)
{
this.acquireReadLock();
try
{
return this.delegate.collectInt(intFunction);
}
finally
{
this.unlockReadLock();
}
}
public MutableLongList collectLong(LongFunction super T> longFunction)
{
this.acquireReadLock();
try
{
return this.delegate.collectLong(longFunction);
}
finally
{
this.unlockReadLock();
}
}
public MutableShortList collectShort(ShortFunction super T> shortFunction)
{
this.acquireReadLock();
try
{
return this.delegate.collectShort(shortFunction);
}
finally
{
this.unlockReadLock();
}
}
public MutableList flatCollect(
Function super T, ? extends Iterable> function)
{
this.acquireReadLock();
try
{
return this.delegate.flatCollect(function);
}
finally
{
this.unlockReadLock();
}
}
public MutableList collectIf(
Predicate super T> predicate,
Function super T, ? extends V> function)
{
this.acquireReadLock();
try
{
return this.delegate.collectIf(predicate, function);
}
finally
{
this.unlockReadLock();
}
}
public MutableList collectWith(
Function2 super T, ? super P, ? extends V> function,
P parameter)
{
this.acquireReadLock();
try
{
return this.delegate.collectWith(function, parameter);
}
finally
{
this.unlockReadLock();
}
}
public MutableList newEmpty()
{
return MultiReaderFastList.newList();
}
public MutableList reject(Predicate super T> predicate)
{
this.acquireReadLock();
try
{
return this.delegate.reject(predicate);
}
finally
{
this.unlockReadLock();
}
}
public MutableList rejectWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
this.acquireReadLock();
try
{
return this.delegate.rejectWith(predicate, parameter);
}
finally
{
this.unlockReadLock();
}
}
public MutableList select(Predicate super T> predicate)
{
this.acquireReadLock();
try
{
return this.delegate.select(predicate);
}
finally
{
this.unlockReadLock();
}
}
public MutableList selectWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
this.acquireReadLock();
try
{
return this.delegate.selectWith(predicate, parameter);
}
finally
{
this.unlockReadLock();
}
}
public PartitionMutableList partition(Predicate super T> predicate)
{
this.acquireReadLock();
try
{
return this.delegate.partition(predicate);
}
finally
{
this.unlockReadLock();
}
}
public PartitionMutableList partitionWith(Predicate2 super T, ? super P> predicate, P parameter)
{
this.acquireReadLock();
try
{
return this.delegate.partitionWith(predicate, parameter);
}
finally
{
this.unlockReadLock();
}
}
public MutableList selectInstancesOf(Class clazz)
{
this.acquireReadLock();
try
{
return this.delegate.selectInstancesOf(clazz);
}
finally
{
this.unlockReadLock();
}
}
public MutableList distinct()
{
this.acquireReadLock();
try
{
return this.delegate.distinct();
}
finally
{
this.unlockReadLock();
}
}
public MutableList sortThis()
{
this.acquireWriteLock();
try
{
this.delegate.sortThis();
return this;
}
finally
{
this.unlockWriteLock();
}
}
public MutableList sortThis(Comparator super T> comparator)
{
this.acquireWriteLock();
try
{
this.delegate.sortThis(comparator);
return this;
}
finally
{
this.unlockWriteLock();
}
}
public > MutableList sortThisBy(
Function super T, ? extends V> function)
{
this.acquireWriteLock();
try
{
this.delegate.sortThisBy(function);
return this;
}
finally
{
this.unlockWriteLock();
}
}
public MutableList subList(int fromIndex, int toIndex)
{
this.acquireReadLock();
try
{
return new MultiReaderFastList(this.delegate.subList(fromIndex, toIndex), this.lock);
}
finally
{
this.unlockReadLock();
}
}
@Override
public boolean equals(Object o)
{
this.acquireReadLock();
try
{
return this.delegate.equals(o);
}
finally
{
this.unlockReadLock();
}
}
@Override
public int hashCode()
{
this.acquireReadLock();
try
{
return this.delegate.hashCode();
}
finally
{
this.unlockReadLock();
}
}
public T get(int index)
{
this.acquireReadLock();
try
{
return this.delegate.get(index);
}
finally
{
this.unlockReadLock();
}
}
public int indexOf(Object o)
{
this.acquireReadLock();
try
{
return this.delegate.indexOf(o);
}
finally
{
this.unlockReadLock();
}
}
public int lastIndexOf(Object o)
{
this.acquireReadLock();
try
{
return this.delegate.lastIndexOf(o);
}
finally
{
this.unlockReadLock();
}
}
public MutableList with(T element)
{
this.add(element);
return this;
}
public MutableList without(T element)
{
this.remove(element);
return this;
}
public MutableList withAll(Iterable extends T> elements)
{
this.addAllIterable(elements);
return this;
}
public MutableList withoutAll(Iterable extends T> elements)
{
this.removeAllIterable(elements);
return this;
}
/**
* This method is not supported directly on a MultiReaderFastList. If you would like to use a ListIterator with
* MultiReaderFastList, then you must do the following:
*
*
* multiReaderList.withReadLockAndDelegate(new Procedure>()
* {
* public void value(MutableList people)
* {
* Iterator it = people.listIterator();
* ....
* }
* });
*
*/
public ListIterator listIterator()
{
throw new UnsupportedOperationException(
"ListIterator is not supported for MultiReaderFastList. "
+ "If you would like to use a ListIterator, you must either use withReadLockAndDelegate() or withWriteLockAndDelegate().");
}
/**
* This method is not supported directly on a MultiReaderFastList. If you would like to use a ListIterator with
* MultiReaderFastList, then you must do the following:
*
*
* multiReaderList.withReadLockAndDelegate(new Procedure>()
* {
* public void value(MutableList people)
* {
* Iterator it = people.listIterator(0);
* ....
* }
* });
*
*/
public ListIterator listIterator(int index)
{
throw new UnsupportedOperationException(
"ListIterator is not supported for MultiReaderFastList. "
+ "If you would like to use a ListIterator, you must either use withReadLockAndDelegate() or withWriteLockAndDelegate().");
}
public T remove(int index)
{
this.acquireWriteLock();
try
{
return this.delegate.remove(index);
}
finally
{
this.unlockWriteLock();
}
}
public T set(int index, T element)
{
this.acquireWriteLock();
try
{
return this.delegate.set(index, element);
}
finally
{
this.unlockWriteLock();
}
}
public boolean addAll(int index, Collection extends T> collection)
{
this.acquireWriteLock();
try
{
return this.delegate.addAll(index, collection);
}
finally
{
this.unlockWriteLock();
}
}
public void add(int index, T element)
{
this.acquireWriteLock();
try
{
this.delegate.add(index, element);
}
finally
{
this.unlockWriteLock();
}
}
public void forEach(int startIndex, int endIndex, Procedure super T> procedure)
{
this.acquireReadLock();
try
{
this.delegate.forEach(startIndex, endIndex, procedure);
}
finally
{
this.unlockReadLock();
}
}
public int binarySearch(T key, Comparator super T> comparator)
{
this.acquireReadLock();
try
{
return Collections.binarySearch(this, key, comparator);
}
finally
{
this.unlockReadLock();
}
}
public int binarySearch(T key)
{
this.acquireReadLock();
try
{
return Collections.binarySearch((List extends Comparable super T>>) this, key);
}
finally
{
this.unlockReadLock();
}
}
public void reverseForEach(final Procedure super T> procedure)
{
this.withReadLockRun(new Runnable()
{
public void run()
{
MultiReaderFastList.this.getDelegate().reverseForEach(procedure);
}
});
}
public void forEachWithIndex(final int fromIndex, final int toIndex, final ObjectIntProcedure super T> objectIntProcedure)
{
this.withReadLockRun(new Runnable()
{
public void run()
{
MultiReaderFastList.this.getDelegate().forEachWithIndex(fromIndex, toIndex, objectIntProcedure);
}
});
}
public void writeExternal(ObjectOutput out) throws IOException
{
out.writeObject(this.delegate);
}
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException
{
this.delegate = (MutableList) in.readObject();
this.lock = new ReentrantReadWriteLock();
}
// Exposed for testing
static final class UntouchableMutableList
extends UntouchableMutableCollection
implements MutableList
{
private final MutableList> requestedIterators = mList();
private final MutableList> requestedSubLists = mList();
private UntouchableMutableList(MutableList delegate)
{
this.delegate = delegate;
}
public MutableList with(T element)
{
this.add(element);
return this;
}
public MutableList without(T element)
{
this.remove(element);
return this;
}
public MutableList withAll(Iterable extends T> elements)
{
this.addAllIterable(elements);
return this;
}
public MutableList withoutAll(Iterable extends T> elements)
{
this.removeAllIterable(elements);
return this;
}
public MutableList asSynchronized()
{
throw new UnsupportedOperationException("Cannot call asSynchronized() on " + this.getClass().getSimpleName());
}
public MutableList asUnmodifiable()
{
throw new UnsupportedOperationException("Cannot call asUnmodifiable() on " + this.getClass().getSimpleName());
}
public LazyIterable asLazy()
{
return LazyIterate.adapt(this);
}
public ImmutableList toImmutable()
{
return Lists.immutable.ofAll(this.getDelegate());
}
@Override
public MutableList clone()
{
return this.getDelegate().clone();
}
public MutableList collect(Function super T, ? extends V> function)
{
return this.getDelegate().collect(function);
}
public MutableBooleanList collectBoolean(BooleanFunction super T> booleanFunction)
{
return this.getDelegate().collectBoolean(booleanFunction);
}
public R collectBoolean(BooleanFunction super T> booleanFunction, R target)
{
return this.getDelegate().collectBoolean(booleanFunction, target);
}
public MutableByteList collectByte(ByteFunction super T> byteFunction)
{
return this.getDelegate().collectByte(byteFunction);
}
public R collectByte(ByteFunction super T> byteFunction, R target)
{
return this.getDelegate().collectByte(byteFunction, target);
}
public MutableCharList collectChar(CharFunction super T> charFunction)
{
return this.getDelegate().collectChar(charFunction);
}
public R collectChar(CharFunction super T> charFunction, R target)
{
return this.getDelegate().collectChar(charFunction, target);
}
public MutableDoubleList collectDouble(DoubleFunction super T> doubleFunction)
{
return this.getDelegate().collectDouble(doubleFunction);
}
public R collectDouble(DoubleFunction super T> doubleFunction, R target)
{
return this.getDelegate().collectDouble(doubleFunction, target);
}
public MutableFloatList collectFloat(FloatFunction super T> floatFunction)
{
return this.getDelegate().collectFloat(floatFunction);
}
public R collectFloat(FloatFunction super T> floatFunction, R target)
{
return this.getDelegate().collectFloat(floatFunction, target);
}
public MutableIntList collectInt(IntFunction super T> intFunction)
{
return this.getDelegate().collectInt(intFunction);
}
public R collectInt(IntFunction super T> intFunction, R target)
{
return this.getDelegate().collectInt(intFunction, target);
}
public MutableLongList collectLong(LongFunction super T> longFunction)
{
return this.getDelegate().collectLong(longFunction);
}
public R collectLong(LongFunction super T> longFunction, R target)
{
return this.getDelegate().collectLong(longFunction, target);
}
public MutableShortList collectShort(ShortFunction super T> shortFunction)
{
return this.getDelegate().collectShort(shortFunction);
}
public R collectShort(ShortFunction super T> shortFunction, R target)
{
return this.getDelegate().collectShort(shortFunction, target);
}
public MutableList flatCollect(Function super T, ? extends Iterable> function)
{
return this.getDelegate().flatCollect(function);
}
public MutableList collectIf(
Predicate super T> predicate,
Function super T, ? extends V> function)
{
return this.getDelegate().collectIf(predicate, function);
}
public MutableList collectWith(
Function2 super T, ? super P, ? extends V> function,
P parameter)
{
return this.getDelegate().collectWith(function, parameter);
}
public MutableListMultimap groupBy(Function super T, ? extends V> function)
{
return this.getDelegate().groupBy(function);
}
public MutableListMultimap groupByEach(Function super T, ? extends Iterable> function)
{
return this.getDelegate().groupByEach(function);
}
public MutableMap groupByUniqueKey(Function super T, ? extends V> function)
{
return this.getDelegate().groupByUniqueKey(function);
}
public void forEach(int fromIndex, int toIndex, Procedure super T> procedure)
{
this.getDelegate().forEach(fromIndex, toIndex, procedure);
}
public void reverseForEach(Procedure super T> procedure)
{
this.getDelegate().reverseForEach(procedure);
}
public void forEachWithIndex(int fromIndex, int toIndex, ObjectIntProcedure super T> objectIntProcedure)
{
this.getDelegate().forEachWithIndex(fromIndex, toIndex, objectIntProcedure);
}
public MutableList newEmpty()
{
return this.getDelegate().newEmpty();
}
public MutableList reject(Predicate super T> predicate)
{
return this.getDelegate().reject(predicate);
}
public MutableList distinct()
{
return this.getDelegate().distinct();
}
public MutableList rejectWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
return this.getDelegate().rejectWith(predicate, parameter);
}
public MutableList select(Predicate super T> predicate)
{
return this.getDelegate().select(predicate);
}
public MutableList selectWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
return this.getDelegate().selectWith(predicate, parameter);
}
public PartitionMutableList partition(Predicate super T> predicate)
{
return this.getDelegate().partition(predicate);
}
public PartitionMutableList partitionWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return this.getDelegate().partitionWith(predicate, parameter);
}
public MutableList selectInstancesOf(Class clazz)
{
return this.getDelegate().selectInstancesOf(clazz);
}
public MutableList sortThis()
{
this.getDelegate().sortThis();
return this;
}
public MutableList sortThis(Comparator super T> comparator)
{
this.getDelegate().sortThis(comparator);
return this;
}
public MutableList toReversed()
{
return this.getDelegate().toReversed();
}
public MutableList reverseThis()
{
this.getDelegate().reverseThis();
return this;
}
public MutableStack toStack()
{
return ArrayStack.newStack(this.delegate);
}
public > MutableList sortThisBy(Function super T, ? extends V> function)
{
this.getDelegate().sortThisBy(function);
return this;
}
public MutableList takeWhile(Predicate super T> predicate)
{
return this.getDelegate().takeWhile(predicate);
}
public MutableList dropWhile(Predicate super T> predicate)
{
return this.getDelegate().dropWhile(predicate);
}
public PartitionMutableList partitionWhile(Predicate super T> predicate)
{
return this.getDelegate().partitionWhile(predicate);
}
public MutableList subList(int fromIndex, int toIndex)
{
UntouchableMutableList subList = new UntouchableMutableList(
this.getDelegate().subList(fromIndex, toIndex));
this.requestedSubLists.add(subList);
return subList;
}
public Iterator iterator()
{
UntouchableListIterator iterator = new UntouchableListIterator(this.delegate.iterator());
this.requestedIterators.add(iterator);
return iterator;
}
public void add(int index, T element)
{
this.getDelegate().add(index, element);
}
public boolean addAll(int index, Collection extends T> collection)
{
return this.getDelegate().addAll(index, collection);
}
public T get(int index)
{
return this.getDelegate().get(index);
}
public int indexOf(Object o)
{
return this.getDelegate().indexOf(o);
}
public int lastIndexOf(Object o)
{
return this.getDelegate().lastIndexOf(o);
}
public ListIterator listIterator()
{
UntouchableListIterator iterator = new UntouchableListIterator(this.getDelegate().listIterator());
this.requestedIterators.add(iterator);
return iterator;
}
public ListIterator listIterator(int index)
{
UntouchableListIterator iterator = new UntouchableListIterator(this.getDelegate().listIterator(index));
this.requestedIterators.add(iterator);
return iterator;
}
public T remove(int index)
{
return this.getDelegate().remove(index);
}
public T set(int index, T element)
{
return this.getDelegate().set(index, element);
}
public MutableList> zip(Iterable that)
{
return this.getDelegate().zip(that);
}
public MutableList> zipWithIndex()
{
return this.getDelegate().zipWithIndex();
}
public LazyIterable asReversed()
{
return ReverseIterable.adapt(this);
}
public int binarySearch(T key, Comparator super T> comparator)
{
return Collections.binarySearch(this, key, comparator);
}
public int binarySearch(T key)
{
return Collections.binarySearch((List extends Comparable super T>>) this, key);
}
public void becomeUseless()
{
this.delegate = null;
this.requestedSubLists.forEach(new Procedure>()
{
public void value(UntouchableMutableList each)
{
each.becomeUseless();
}
});
this.requestedIterators.forEach(new Procedure>()
{
public void value(UntouchableListIterator each)
{
each.becomeUseless();
}
});
}
private MutableList getDelegate()
{
return (MutableList) this.delegate;
}
}
private static final class UntouchableListIterator
implements ListIterator
{
private Iterator delegate;
private UntouchableListIterator(Iterator newDelegate)
{
this.delegate = newDelegate;
}
public void add(T o)
{
((ListIterator) this.delegate).add(o);
}
public boolean hasNext()
{
return this.delegate.hasNext();
}
public boolean hasPrevious()
{
return ((ListIterator) this.delegate).hasPrevious();
}
public T next()
{
return this.delegate.next();
}
public int nextIndex()
{
return ((ListIterator) this.delegate).nextIndex();
}
public T previous()
{
return ((ListIterator) this.delegate).previous();
}
public int previousIndex()
{
return ((ListIterator) this.delegate).previousIndex();
}
public void remove()
{
this.delegate.remove();
}
public void set(T o)
{
((ListIterator) this.delegate).set(o);
}
public void becomeUseless()
{
this.delegate = null;
}
}
public MutableListMultimap groupBy(Function super T, ? extends V> function)
{
this.acquireReadLock();
try
{
return this.delegate.groupBy(function);
}
finally
{
this.unlockReadLock();
}
}
public MutableListMultimap groupByEach(Function super T, ? extends Iterable> function)
{
this.acquireReadLock();
try
{
return this.delegate.groupByEach(function);
}
finally
{
this.unlockReadLock();
}
}
public MutableMap groupByUniqueKey(Function super T, ? extends V> function)
{
this.acquireReadLock();
try
{
return this.delegate.groupByUniqueKey(function);
}
finally
{
this.unlockReadLock();
}
}
public MutableList> zip(Iterable that)
{
this.acquireReadLock();
try
{
return this.delegate.zip(that);
}
finally
{
this.unlockReadLock();
}
}
public MutableList> zipWithIndex()
{
this.acquireReadLock();
try
{
return this.delegate.zipWithIndex();
}
finally
{
this.unlockReadLock();
}
}
public MutableList toReversed()
{
this.acquireReadLock();
try
{
return this.delegate.toReversed();
}
finally
{
this.unlockReadLock();
}
}
public MutableList reverseThis()
{
this.acquireWriteLock();
try
{
this.delegate.reverseThis();
return this;
}
finally
{
this.unlockWriteLock();
}
}
public MutableStack toStack()
{
this.acquireReadLock();
try
{
return this.delegate.toStack();
}
finally
{
this.unlockReadLock();
}
}
public RichIterable> chunk(int size)
{
this.acquireReadLock();
try
{
return this.delegate.chunk(size);
}
finally
{
this.unlockReadLock();
}
}
public MutableList takeWhile(Predicate super T> predicate)
{
this.acquireReadLock();
try
{
return this.delegate.takeWhile(predicate);
}
finally
{
this.unlockReadLock();
}
}
public MutableList dropWhile(Predicate super T> predicate)
{
this.acquireReadLock();
try
{
return this.delegate.dropWhile(predicate);
}
finally
{
this.unlockReadLock();
}
}
public PartitionMutableList partitionWhile(Predicate super T> predicate)
{
this.acquireReadLock();
try
{
return this.delegate.partitionWhile(predicate);
}
finally
{
this.unlockReadLock();
}
}
public LazyIterable asReversed()
{
this.acquireReadLock();
try
{
return ReverseIterable.adapt(this);
}
finally
{
this.unlockReadLock();
}
}
}