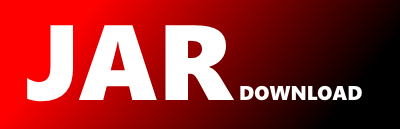
com.gs.collections.impl.map.mutable.primitive.ShortByteHashMap Maven / Gradle / Ivy
Show all versions of gs-collections Show documentation
/*
* Copyright 2014 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.map.mutable.primitive;
import java.io.Externalizable;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.Arrays;
import java.util.Iterator;
import java.util.NoSuchElementException;
import com.gs.collections.api.ShortIterable;
import com.gs.collections.api.LazyShortIterable;
import com.gs.collections.api.LazyByteIterable;
import com.gs.collections.api.ByteIterable;
import com.gs.collections.api.LazyIterable;
import com.gs.collections.api.RichIterable;
import com.gs.collections.api.bag.primitive.MutableShortBag;
import com.gs.collections.api.bag.primitive.MutableByteBag;
import com.gs.collections.api.block.function.primitive.ShortToObjectFunction;
import com.gs.collections.api.block.function.primitive.ShortToByteFunction;
import com.gs.collections.api.block.function.primitive.ByteFunction;
import com.gs.collections.api.block.function.primitive.ByteFunction0;
import com.gs.collections.api.block.function.primitive.ByteToByteFunction;
import com.gs.collections.api.block.function.primitive.ByteToObjectFunction;
import com.gs.collections.api.block.function.primitive.ObjectByteToObjectFunction;
import com.gs.collections.api.block.function.primitive.ObjectShortToObjectFunction;
import com.gs.collections.api.block.predicate.primitive.ShortBytePredicate;
import com.gs.collections.api.block.predicate.primitive.ShortPredicate;
import com.gs.collections.api.block.predicate.primitive.BytePredicate;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.api.block.procedure.Procedure2;
import com.gs.collections.api.block.procedure.primitive.ShortProcedure;
import com.gs.collections.api.block.procedure.primitive.ShortByteProcedure;
import com.gs.collections.api.block.procedure.primitive.ByteProcedure;
import com.gs.collections.api.block.procedure.primitive.ObjectIntProcedure;
import com.gs.collections.api.collection.MutableCollection;
import com.gs.collections.api.collection.primitive.ImmutableByteCollection;
import com.gs.collections.api.collection.primitive.MutableByteCollection;
import com.gs.collections.api.iterator.ShortIterator;
import com.gs.collections.api.iterator.ByteIterator;
import com.gs.collections.api.list.primitive.MutableShortList;
import com.gs.collections.api.list.primitive.MutableByteList;
import com.gs.collections.api.map.primitive.ShortByteMap;
import com.gs.collections.api.map.primitive.ImmutableShortByteMap;
import com.gs.collections.api.map.primitive.MutableShortByteMap;
import com.gs.collections.api.set.MutableSet;
import com.gs.collections.api.set.primitive.ShortSet;
import com.gs.collections.api.set.primitive.ByteSet;
import com.gs.collections.api.set.primitive.ImmutableShortSet;
import com.gs.collections.api.set.primitive.MutableShortSet;
import com.gs.collections.api.tuple.primitive.ShortBytePair;
import com.gs.collections.api.set.primitive.MutableByteSet;
import com.gs.collections.impl.bag.mutable.primitive.ShortHashBag;
import com.gs.collections.impl.bag.mutable.primitive.ByteHashBag;
import com.gs.collections.impl.block.factory.primitive.ShortPredicates;
import com.gs.collections.impl.collection.mutable.primitive.SynchronizedByteCollection;
import com.gs.collections.impl.collection.mutable.primitive.UnmodifiableByteCollection;
import com.gs.collections.impl.factory.Sets;
import com.gs.collections.impl.factory.primitive.ByteLists;
import com.gs.collections.impl.factory.primitive.ShortByteMaps;
import com.gs.collections.impl.factory.primitive.ShortSets;
import com.gs.collections.impl.lazy.AbstractLazyIterable;
import com.gs.collections.impl.lazy.primitive.AbstractLazyShortIterable;
import com.gs.collections.impl.lazy.primitive.LazyByteIterableAdapter;
import com.gs.collections.impl.lazy.primitive.LazyShortIterableAdapter;
import com.gs.collections.impl.list.mutable.FastList;
import com.gs.collections.impl.list.mutable.primitive.ShortArrayList;
import com.gs.collections.impl.list.mutable.primitive.ByteArrayList;
import com.gs.collections.impl.set.mutable.primitive.ShortHashSet;
import com.gs.collections.impl.set.mutable.primitive.SynchronizedShortSet;
import com.gs.collections.impl.set.mutable.primitive.UnmodifiableShortSet;
import com.gs.collections.impl.set.mutable.primitive.ByteHashSet;
import com.gs.collections.impl.tuple.primitive.PrimitiveTuples;
/**
* This file was automatically generated from template file primitivePrimitiveHashMap.stg.
*
* @since 3.0.
*/
public class ShortByteHashMap implements MutableShortByteMap, Externalizable
{
static final byte EMPTY_VALUE = (byte) 0;
private static final long serialVersionUID = 1L;
private static final short EMPTY_KEY = (short) 0;
private static final short REMOVED_KEY = (short) 1;
private static final int OCCUPIED_DATA_RATIO = 2;
private static final int OCCUPIED_SENTINEL_RATIO = 4;
private static final int DEFAULT_INITIAL_CAPACITY = 8;
private short[] keys;
private byte[] values;
private int occupiedWithData;
private int occupiedWithSentinels;
private SentinelValues sentinelValues;
public ShortByteHashMap()
{
this.allocateTable(DEFAULT_INITIAL_CAPACITY << 1);
}
public ShortByteHashMap(int initialCapacity)
{
if (initialCapacity < 0)
{
throw new IllegalArgumentException("initial capacity cannot be less than 0");
}
int capacity = this.smallestPowerOfTwoGreaterThan(this.fastCeil(initialCapacity * OCCUPIED_DATA_RATIO));
this.allocateTable(capacity);
}
private int smallestPowerOfTwoGreaterThan(int n)
{
return n > 1 ? Integer.highestOneBit(n - 1) << 1 : 1;
}
public ShortByteHashMap(ShortByteMap map)
{
this(Math.max(map.size(), DEFAULT_INITIAL_CAPACITY));
this.putAll(map);
}
private int fastCeil(float v)
{
int possibleResult = (int) v;
if (v - possibleResult > 0.0F)
{
possibleResult++;
}
return possibleResult;
}
public static ShortByteHashMap newWithKeysValues(short key1, byte value1)
{
return new ShortByteHashMap(1).withKeyValue(key1, value1);
}
public static ShortByteHashMap newWithKeysValues(short key1, byte value1, short key2, byte value2)
{
return new ShortByteHashMap(2).withKeysValues(key1, value1, key2, value2);
}
public static ShortByteHashMap newWithKeysValues(short key1, byte value1, short key2, byte value2, short key3, byte value3)
{
return new ShortByteHashMap(3).withKeysValues(key1, value1, key2, value2, key3, value3);
}
public static ShortByteHashMap newWithKeysValues(short key1, byte value1, short key2, byte value2, short key3, byte value3, short key4, byte value4)
{
return new ShortByteHashMap(4).withKeysValues(key1, value1, key2, value2, key3, value3, key4, value4);
}
@Override
public boolean equals(Object obj)
{
if (this == obj)
{
return true;
}
if (!(obj instanceof ShortByteMap))
{
return false;
}
ShortByteMap other = (ShortByteMap) obj;
if (this.size() != other.size())
{
return false;
}
if (this.sentinelValues == null)
{
if (other.containsKey(EMPTY_KEY) || other.containsKey(REMOVED_KEY))
{
return false;
}
}
else
{
if (this.sentinelValues.containsZeroKey && (!other.containsKey(EMPTY_KEY) || this.sentinelValues.zeroValue != other.getOrThrow(EMPTY_KEY)))
{
return false;
}
if (this.sentinelValues.containsOneKey && (!other.containsKey(REMOVED_KEY) || this.sentinelValues.oneValue != other.getOrThrow(REMOVED_KEY)))
{
return false;
}
}
for (int i = 0; i < this.keys.length; i++)
{
short key = this.keys[i];
if (isNonSentinel(key) && (!other.containsKey(key) || this.values[i] != other.getOrThrow(key)))
{
return false;
}
}
return true;
}
@Override
public int hashCode()
{
int result = 0;
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey)
{
result += (int) EMPTY_KEY ^ (int) this.sentinelValues.zeroValue;
}
if (this.sentinelValues.containsOneKey)
{
result += (int) REMOVED_KEY ^ (int) this.sentinelValues.oneValue;
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]))
{
result += (int) this.keys[i] ^ (int) this.values[i];
}
}
return result;
}
@Override
public String toString()
{
StringBuilder appendable = new StringBuilder();
appendable.append("{");
boolean first = true;
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey)
{
appendable.append(String.valueOf(EMPTY_KEY)).append("=").append(String.valueOf(this.sentinelValues.zeroValue));
first = false;
}
if (this.sentinelValues.containsOneKey)
{
if (!first)
{
appendable.append(", ");
}
appendable.append(String.valueOf(REMOVED_KEY)).append("=").append(String.valueOf(this.sentinelValues.oneValue));
first = false;
}
}
for (int i = 0; i < this.keys.length; i++)
{
short key = this.keys[i];
if (isNonSentinel(key))
{
if (!first)
{
appendable.append(", ");
}
appendable.append(String.valueOf(key)).append("=").append(String.valueOf(this.values[i]));
first = false;
}
}
appendable.append("}");
return appendable.toString();
}
public int size()
{
return this.occupiedWithData + (this.sentinelValues == null ? 0 : this.sentinelValues.size());
}
public boolean isEmpty()
{
return this.occupiedWithData == 0 && (this.sentinelValues == null || this.sentinelValues.size() == 0);
}
public boolean notEmpty()
{
return this.occupiedWithData != 0 || (this.sentinelValues != null && this.sentinelValues.size() != 0);
}
public String makeString()
{
return this.makeString(", ");
}
public String makeString(String separator)
{
return this.makeString("", separator, "");
}
public String makeString(String start, String separator, String end)
{
Appendable stringBuilder = new StringBuilder();
this.appendString(stringBuilder, start, separator, end);
return stringBuilder.toString();
}
public void appendString(Appendable appendable)
{
this.appendString(appendable, ", ");
}
public void appendString(Appendable appendable, String separator)
{
this.appendString(appendable, "", separator, "");
}
public void appendString(Appendable appendable, String start, String separator, String end)
{
try
{
appendable.append(start);
boolean first = true;
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey)
{
appendable.append(String.valueOf(this.sentinelValues.zeroValue));
first = false;
}
if (this.sentinelValues.containsOneKey)
{
if (!first)
{
appendable.append(separator);
}
appendable.append(String.valueOf(this.sentinelValues.oneValue));
first = false;
}
}
for (int i = 0; i < this.keys.length; i++)
{
short key = this.keys[i];
if (isNonSentinel(key))
{
if (!first)
{
appendable.append(separator);
}
appendable.append(String.valueOf(this.values[i]));
first = false;
}
}
appendable.append(end);
}
catch (IOException e)
{
throw new RuntimeException(e);
}
}
public ByteIterator byteIterator()
{
return new InternalByteIterator();
}
public byte[] toArray()
{
byte[] array = new byte[this.size()];
int index = 0;
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey)
{
array[index] = this.sentinelValues.zeroValue;
index++;
}
if (this.sentinelValues.containsOneKey)
{
array[index] = this.sentinelValues.oneValue;
index++;
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]))
{
array[index] = this.values[i];
index++;
}
}
return array;
}
public boolean contains(byte value)
{
return this.containsValue(value);
}
public boolean containsAll(byte... source)
{
for (byte each : source)
{
if (!this.contains(each))
{
return false;
}
}
return true;
}
public boolean containsAll(ByteIterable source)
{
return source.allSatisfy(new BytePredicate()
{
public boolean accept(byte value)
{
return ShortByteHashMap.this.contains(value);
}
});
}
public void forEach(ByteProcedure procedure)
{
this.forEachValue(procedure);
}
public MutableByteCollection select(BytePredicate predicate)
{
ByteArrayList result = new ByteArrayList();
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey && predicate.accept(this.sentinelValues.zeroValue))
{
result.add(this.sentinelValues.zeroValue);
}
if (this.sentinelValues.containsOneKey && predicate.accept(this.sentinelValues.oneValue))
{
result.add(this.sentinelValues.oneValue);
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]) && predicate.accept(this.values[i]))
{
result.add(this.values[i]);
}
}
return result;
}
public MutableByteCollection reject(BytePredicate predicate)
{
ByteArrayList result = new ByteArrayList();
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey && !predicate.accept(this.sentinelValues.zeroValue))
{
result.add(this.sentinelValues.zeroValue);
}
if (this.sentinelValues.containsOneKey && !predicate.accept(this.sentinelValues.oneValue))
{
result.add(this.sentinelValues.oneValue);
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]) && !predicate.accept(this.values[i]))
{
result.add(this.values[i]);
}
}
return result;
}
public MutableCollection collect(ByteToObjectFunction extends V> function)
{
FastList target = FastList.newList(this.size());
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey)
{
target.add(function.valueOf(this.sentinelValues.zeroValue));
}
if (this.sentinelValues.containsOneKey)
{
target.add(function.valueOf(this.sentinelValues.oneValue));
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]))
{
target.add(function.valueOf(this.values[i]));
}
}
return target;
}
public byte detectIfNone(BytePredicate predicate, byte value)
{
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey && predicate.accept(this.sentinelValues.zeroValue))
{
return this.sentinelValues.zeroValue;
}
if (this.sentinelValues.containsOneKey && predicate.accept(this.sentinelValues.oneValue))
{
return this.sentinelValues.oneValue;
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]) && predicate.accept(this.values[i]))
{
return this.values[i];
}
}
return value;
}
public int count(BytePredicate predicate)
{
int count = 0;
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey && predicate.accept(this.sentinelValues.zeroValue))
{
count++;
}
if (this.sentinelValues.containsOneKey && predicate.accept(this.sentinelValues.oneValue))
{
count++;
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]) && predicate.accept(this.values[i]))
{
count++;
}
}
return count;
}
public boolean anySatisfy(BytePredicate predicate)
{
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey && predicate.accept(this.sentinelValues.zeroValue))
{
return true;
}
if (this.sentinelValues.containsOneKey && predicate.accept(this.sentinelValues.oneValue))
{
return true;
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]) && predicate.accept(this.values[i]))
{
return true;
}
}
return false;
}
public boolean allSatisfy(BytePredicate predicate)
{
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey && !predicate.accept(this.sentinelValues.zeroValue))
{
return false;
}
if (this.sentinelValues.containsOneKey && !predicate.accept(this.sentinelValues.oneValue))
{
return false;
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]) && !predicate.accept(this.values[i]))
{
return false;
}
}
return true;
}
public boolean noneSatisfy(BytePredicate predicate)
{
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey && predicate.accept(this.sentinelValues.zeroValue))
{
return false;
}
if (this.sentinelValues.containsOneKey && predicate.accept(this.sentinelValues.oneValue))
{
return false;
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]) && predicate.accept(this.values[i]))
{
return false;
}
}
return true;
}
public V injectInto(V injectedValue, ObjectByteToObjectFunction super V, ? extends V> function)
{
V result = injectedValue;
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey)
{
result = function.valueOf(result, this.sentinelValues.zeroValue);
}
if (this.sentinelValues.containsOneKey)
{
result = function.valueOf(result, this.sentinelValues.oneValue);
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]))
{
result = function.valueOf(result, this.values[i]);
}
}
return result;
}
public MutableByteList toList()
{
return ByteArrayList.newList(this);
}
public MutableByteSet toSet()
{
return ByteHashSet.newSet(this);
}
public MutableByteBag toBag()
{
return ByteHashBag.newBag(this);
}
public LazyByteIterable asLazy()
{
return new LazyByteIterableAdapter(this);
}
public void clear()
{
this.sentinelValues = null;
this.occupiedWithData = 0;
this.occupiedWithSentinels = 0;
Arrays.fill(this.keys, EMPTY_KEY);
Arrays.fill(this.values, EMPTY_VALUE);
}
public void put(short key, byte value)
{
if (isEmptyKey(key))
{
if (this.sentinelValues == null)
{
this.sentinelValues = new SentinelValues();
}
this.addEmptyKeyValue(value);
return;
}
if (isRemovedKey(key))
{
if (this.sentinelValues == null)
{
this.sentinelValues = new SentinelValues();
}
this.addRemovedKeyValue(value);
return;
}
int index = this.probe(key);
if (this.keys[index] == key)
{
// key already present in map
this.values[index] = value;
return;
}
this.addKeyValueAtIndex(key, value, index);
}
public void putAll(ShortByteMap map)
{
map.forEachKeyValue(new ShortByteProcedure()
{
public void value(short key, byte value)
{
ShortByteHashMap.this.put(key, value);
}
});
}
public void removeKey(short key)
{
if (isEmptyKey(key))
{
if (this.sentinelValues == null || !this.sentinelValues.containsZeroKey)
{
return;
}
this.removeEmptyKey();
return;
}
if (isRemovedKey(key))
{
if (this.sentinelValues == null || !this.sentinelValues.containsOneKey)
{
return;
}
this.removeRemovedKey();
return;
}
int index = this.probe(key);
if (this.keys[index] == key)
{
this.keys[index] = REMOVED_KEY;
this.values[index] = EMPTY_VALUE;
this.occupiedWithData--;
this.occupiedWithSentinels++;
if (this.occupiedWithSentinels > this.maxOccupiedWithSentinels())
{
this.rehash();
}
}
}
public void remove(short key)
{
this.removeKey(key);
}
public byte removeKeyIfAbsent(short key, byte value)
{
if (isEmptyKey(key))
{
if (this.sentinelValues == null || !this.sentinelValues.containsZeroKey)
{
return value;
}
byte oldValue = this.sentinelValues.zeroValue;
this.removeEmptyKey();
return oldValue;
}
if (isRemovedKey(key))
{
if (this.sentinelValues == null || !this.sentinelValues.containsOneKey)
{
return value;
}
byte oldValue = this.sentinelValues.oneValue;
this.removeRemovedKey();
return oldValue;
}
int index = this.probe(key);
if (this.keys[index] == key)
{
this.keys[index] = REMOVED_KEY;
byte oldValue = this.values[index];
this.values[index] = EMPTY_VALUE;
this.occupiedWithData--;
this.occupiedWithSentinels++;
if (this.occupiedWithSentinels > this.maxOccupiedWithSentinels())
{
this.rehash();
}
return oldValue;
}
return value;
}
public byte getIfAbsentPut(short key, byte value)
{
if (isEmptyKey(key))
{
if (this.sentinelValues == null)
{
this.sentinelValues = new SentinelValues();
this.addEmptyKeyValue(value);
return value;
}
if (this.sentinelValues.containsZeroKey)
{
return this.sentinelValues.zeroValue;
}
this.addEmptyKeyValue(value);
return value;
}
if (isRemovedKey(key))
{
if (this.sentinelValues == null)
{
this.sentinelValues = new SentinelValues();
this.addRemovedKeyValue(value);
return value;
}
if (this.sentinelValues.containsOneKey)
{
return this.sentinelValues.oneValue;
}
this.addRemovedKeyValue(value);
return value;
}
int index = this.probe(key);
if (this.keys[index] == key)
{
return this.values[index];
}
this.addKeyValueAtIndex(key, value, index);
return value;
}
public byte getIfAbsentPut(short key, ByteFunction0 function)
{
if (isEmptyKey(key))
{
if (this.sentinelValues == null)
{
byte value = function.value();
this.sentinelValues = new SentinelValues();
this.addEmptyKeyValue(value);
return value;
}
if (this.sentinelValues.containsZeroKey)
{
return this.sentinelValues.zeroValue;
}
byte value = function.value();
this.addEmptyKeyValue(value);
return value;
}
if (isRemovedKey(key))
{
if (this.sentinelValues == null)
{
byte value = function.value();
this.sentinelValues = new SentinelValues();
this.addRemovedKeyValue(value);
return value;
}
if (this.sentinelValues.containsOneKey)
{
return this.sentinelValues.oneValue;
}
byte value = function.value();
this.addRemovedKeyValue(value);
return value;
}
int index = this.probe(key);
if (this.keys[index] == key)
{
return this.values[index];
}
byte value = function.value();
this.addKeyValueAtIndex(key, value, index);
return value;
}
public byte getIfAbsentPutWith(short key, ByteFunction super P> function, P parameter)
{
if (isEmptyKey(key))
{
if (this.sentinelValues == null)
{
byte value = function.byteValueOf(parameter);
this.sentinelValues = new SentinelValues();
this.addEmptyKeyValue(value);
return value;
}
if (this.sentinelValues.containsZeroKey)
{
return this.sentinelValues.zeroValue;
}
byte value = function.byteValueOf(parameter);
this.addEmptyKeyValue(value);
return value;
}
if (isRemovedKey(key))
{
if (this.sentinelValues == null)
{
byte value = function.byteValueOf(parameter);
this.sentinelValues = new SentinelValues();
this.addRemovedKeyValue(value);
return value;
}
if (this.sentinelValues.containsOneKey)
{
return this.sentinelValues.oneValue;
}
byte value = function.byteValueOf(parameter);
this.addRemovedKeyValue(value);
return value;
}
int index = this.probe(key);
if (this.keys[index] == key)
{
return this.values[index];
}
byte value = function.byteValueOf(parameter);
this.addKeyValueAtIndex(key, value, index);
return value;
}
public byte getIfAbsentPutWithKey(short key, ShortToByteFunction function)
{
if (isEmptyKey(key))
{
if (this.sentinelValues == null)
{
byte value = function.valueOf(key);
this.sentinelValues = new SentinelValues();
this.addEmptyKeyValue(value);
return value;
}
if (this.sentinelValues.containsZeroKey)
{
return this.sentinelValues.zeroValue;
}
byte value = function.valueOf(key);
this.addEmptyKeyValue(value);
return value;
}
if (isRemovedKey(key))
{
if (this.sentinelValues == null)
{
byte value = function.valueOf(key);
this.sentinelValues = new SentinelValues();
this.addRemovedKeyValue(value);
return value;
}
if (this.sentinelValues.containsOneKey)
{
return this.sentinelValues.oneValue;
}
byte value = function.valueOf(key);
this.addRemovedKeyValue(value);
return value;
}
int index = this.probe(key);
if (this.keys[index] == key)
{
return this.values[index];
}
byte value = function.valueOf(key);
this.addKeyValueAtIndex(key, value, index);
return value;
}
public byte addToValue(short key, byte toBeAdded)
{
if (isEmptyKey(key))
{
if (this.sentinelValues == null)
{
this.sentinelValues = new SentinelValues();
this.addEmptyKeyValue(toBeAdded);
}
else if (this.sentinelValues.containsZeroKey)
{
this.sentinelValues.zeroValue += toBeAdded;
}
else
{
this.addEmptyKeyValue(toBeAdded);
}
return this.sentinelValues.zeroValue;
}
if (isRemovedKey(key))
{
if (this.sentinelValues == null)
{
this.sentinelValues = new SentinelValues();
this.addRemovedKeyValue(toBeAdded);
}
else if (this.sentinelValues.containsOneKey)
{
this.sentinelValues.oneValue += toBeAdded;
}
else
{
this.addRemovedKeyValue(toBeAdded);
}
return this.sentinelValues.oneValue;
}
int index = this.probe(key);
if (this.keys[index] == key)
{
this.values[index] += toBeAdded;
return this.values[index];
}
this.addKeyValueAtIndex(key, toBeAdded, index);
return this.values[index];
}
private void addKeyValueAtIndex(short key, byte value, int index)
{
if (this.keys[index] == REMOVED_KEY)
{
this.occupiedWithSentinels--;
}
this.keys[index] = key;
this.values[index] = value;
this.occupiedWithData++;
if (this.occupiedWithData > this.maxOccupiedWithData())
{
this.rehashAndGrow();
}
}
private void addEmptyKeyValue(byte value)
{
this.sentinelValues.containsZeroKey = true;
this.sentinelValues.zeroValue = value;
}
private void removeEmptyKey()
{
if (this.sentinelValues.containsOneKey)
{
this.sentinelValues.containsZeroKey = false;
this.sentinelValues.zeroValue = EMPTY_VALUE;
}
else
{
this.sentinelValues = null;
}
}
private void addRemovedKeyValue(byte value)
{
this.sentinelValues.containsOneKey = true;
this.sentinelValues.oneValue = value;
}
private void removeRemovedKey()
{
if (this.sentinelValues.containsZeroKey)
{
this.sentinelValues.containsOneKey = false;
this.sentinelValues.oneValue = EMPTY_VALUE;
}
else
{
this.sentinelValues = null;
}
}
public byte updateValue(short key, byte initialValueIfAbsent, ByteToByteFunction function)
{
if (isEmptyKey(key))
{
if (this.sentinelValues == null)
{
this.sentinelValues = new SentinelValues();
this.addEmptyKeyValue(function.valueOf(initialValueIfAbsent));
}
else if (this.sentinelValues.containsZeroKey)
{
this.sentinelValues.zeroValue = function.valueOf(this.sentinelValues.zeroValue);
}
else
{
this.addEmptyKeyValue(function.valueOf(initialValueIfAbsent));
}
return this.sentinelValues.zeroValue;
}
if (isRemovedKey(key))
{
if (this.sentinelValues == null)
{
this.sentinelValues = new SentinelValues();
this.addRemovedKeyValue(function.valueOf(initialValueIfAbsent));
}
else if (this.sentinelValues.containsOneKey)
{
this.sentinelValues.oneValue = function.valueOf(this.sentinelValues.oneValue);
}
else
{
this.addRemovedKeyValue(function.valueOf(initialValueIfAbsent));
}
return this.sentinelValues.oneValue;
}
int index = this.probe(key);
if (this.keys[index] == key)
{
this.values[index] = function.valueOf(this.values[index]);
return this.values[index];
}
byte value = function.valueOf(initialValueIfAbsent);
this.addKeyValueAtIndex(key, value, index);
return value;
}
public ShortByteHashMap withKeyValue(short key1, byte value1)
{
this.put(key1, value1);
return this;
}
public ShortByteHashMap withKeysValues(short key1, byte value1, short key2, byte value2)
{
this.put(key1, value1);
this.put(key2, value2);
return this;
}
public ShortByteHashMap withKeysValues(short key1, byte value1, short key2, byte value2, short key3, byte value3)
{
this.put(key1, value1);
this.put(key2, value2);
this.put(key3, value3);
return this;
}
public ShortByteHashMap withKeysValues(short key1, byte value1, short key2, byte value2, short key3, byte value3, short key4, byte value4)
{
this.put(key1, value1);
this.put(key2, value2);
this.put(key3, value3);
this.put(key4, value4);
return this;
}
public ShortByteHashMap withoutKey(short key)
{
this.removeKey(key);
return this;
}
public ShortByteHashMap withoutAllKeys(ShortIterable keys)
{
keys.forEach(new ShortProcedure()
{
public void value(short key)
{
ShortByteHashMap.this.removeKey(key);
}
});
return this;
}
public MutableShortByteMap asUnmodifiable()
{
return new UnmodifiableShortByteMap(this);
}
public MutableShortByteMap asSynchronized()
{
return new SynchronizedShortByteMap(this);
}
public ImmutableShortByteMap toImmutable()
{
return ShortByteMaps.immutable.ofAll(this);
}
public byte get(short key)
{
return this.getIfAbsent(key, EMPTY_VALUE);
}
public byte getIfAbsent(short key, byte ifAbsent)
{
if (isEmptyKey(key))
{
if (this.sentinelValues == null || !this.sentinelValues.containsZeroKey)
{
return ifAbsent;
}
return this.sentinelValues.zeroValue;
}
if (isRemovedKey(key))
{
if (this.sentinelValues == null || !this.sentinelValues.containsOneKey)
{
return ifAbsent;
}
return this.sentinelValues.oneValue;
}
int index = this.probe(key);
if (this.keys[index] == key)
{
return this.values[index];
}
return ifAbsent;
}
public byte getOrThrow(short key)
{
if (isEmptyKey(key))
{
if (this.sentinelValues == null || !this.sentinelValues.containsZeroKey)
{
throw new IllegalStateException("Key " + key + " not present.");
}
return this.sentinelValues.zeroValue;
}
if (isRemovedKey(key))
{
if (this.sentinelValues == null || !this.sentinelValues.containsOneKey)
{
throw new IllegalStateException("Key " + key + " not present.");
}
return this.sentinelValues.oneValue;
}
int index = this.probe(key);
if (isNonSentinel(this.keys[index]))
{
return this.values[index];
}
throw new IllegalStateException("Key " + key + " not present.");
}
public boolean containsKey(short key)
{
if (isEmptyKey(key))
{
return this.sentinelValues != null && this.sentinelValues.containsZeroKey;
}
if (isRemovedKey(key))
{
return this.sentinelValues != null && this.sentinelValues.containsOneKey;
}
return this.keys[this.probe(key)] == key;
}
public boolean containsValue(byte value)
{
if (this.sentinelValues != null && this.sentinelValues.containsValue(value))
{
return true;
}
for (int i = 0; i < this.values.length; i++)
{
if (isNonSentinel(this.keys[i]) && this.values[i] == value)
{
return true;
}
}
return false;
}
public void forEachValue(ByteProcedure procedure)
{
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey)
{
procedure.value(this.sentinelValues.zeroValue);
}
if (this.sentinelValues.containsOneKey)
{
procedure.value(this.sentinelValues.oneValue);
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]))
{
procedure.value(this.values[i]);
}
}
}
public void forEachKey(ShortProcedure procedure)
{
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey)
{
procedure.value(EMPTY_KEY);
}
if (this.sentinelValues.containsOneKey)
{
procedure.value(REMOVED_KEY);
}
}
for (short key : this.keys)
{
if (isNonSentinel(key))
{
procedure.value(key);
}
}
}
public void forEachKeyValue(ShortByteProcedure procedure)
{
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey)
{
procedure.value(EMPTY_KEY, this.sentinelValues.zeroValue);
}
if (this.sentinelValues.containsOneKey)
{
procedure.value(REMOVED_KEY, this.sentinelValues.oneValue);
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]))
{
procedure.value(this.keys[i], this.values[i]);
}
}
}
public LazyShortIterable keysView()
{
return new KeysView();
}
public RichIterable keyValuesView()
{
return new KeyValuesView();
}
public ShortByteHashMap select(ShortBytePredicate predicate)
{
ShortByteHashMap result = new ShortByteHashMap();
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey && predicate.accept(EMPTY_KEY, this.sentinelValues.zeroValue))
{
result.put(EMPTY_KEY, this.sentinelValues.zeroValue);
}
if (this.sentinelValues.containsOneKey && predicate.accept(REMOVED_KEY, this.sentinelValues.oneValue))
{
result.put(REMOVED_KEY, this.sentinelValues.oneValue);
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]) && predicate.accept(this.keys[i], this.values[i]))
{
result.put(this.keys[i], this.values[i]);
}
}
return result;
}
public ShortByteHashMap reject(ShortBytePredicate predicate)
{
ShortByteHashMap result = new ShortByteHashMap();
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey && !predicate.accept(EMPTY_KEY, this.sentinelValues.zeroValue))
{
result.put(EMPTY_KEY, this.sentinelValues.zeroValue);
}
if (this.sentinelValues.containsOneKey && !predicate.accept(REMOVED_KEY, this.sentinelValues.oneValue))
{
result.put(REMOVED_KEY, this.sentinelValues.oneValue);
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]) && !predicate.accept(this.keys[i], this.values[i]))
{
result.put(this.keys[i], this.values[i]);
}
}
return result;
}
public long sum()
{
long result = 0L;
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey)
{
result += this.sentinelValues.zeroValue;
}
if (this.sentinelValues.containsOneKey)
{
result += this.sentinelValues.oneValue;
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]))
{
result += this.values[i];
}
}
return result;
}
public byte max()
{
if (this.isEmpty())
{
throw new NoSuchElementException();
}
ByteIterator iterator = this.byteIterator();
byte max = iterator.next();
while (iterator.hasNext())
{
byte value = iterator.next();
if (max < value)
{
max = value;
}
}
return max;
}
public byte maxIfEmpty(byte defaultValue)
{
if (this.isEmpty())
{
return defaultValue;
}
return this.max();
}
public byte min()
{
if (this.isEmpty())
{
throw new NoSuchElementException();
}
ByteIterator iterator = this.byteIterator();
byte min = iterator.next();
while (iterator.hasNext())
{
byte value = iterator.next();
if (value < min)
{
min = value;
}
}
return min;
}
public byte minIfEmpty(byte defaultValue)
{
if (this.isEmpty())
{
return defaultValue;
}
return this.min();
}
public double average()
{
if (this.isEmpty())
{
throw new ArithmeticException();
}
return (double) this.sum() / (double) this.size();
}
public double median()
{
if (this.isEmpty())
{
throw new ArithmeticException();
}
byte[] sortedArray = this.toSortedArray();
int middleIndex = sortedArray.length >> 1;
if (sortedArray.length > 1 && (sortedArray.length & 1) == 0)
{
byte first = sortedArray[middleIndex];
byte second = sortedArray[middleIndex - 1];
return ((double) first + (double) second) / 2.0;
}
return (double) sortedArray[middleIndex];
}
public byte[] toSortedArray()
{
byte[] array = this.toArray();
Arrays.sort(array);
return array;
}
public MutableByteList toSortedList()
{
return ByteArrayList.newList(this).sortThis();
}
public void writeExternal(ObjectOutput out) throws IOException
{
out.writeInt(this.size());
if (this.sentinelValues != null)
{
if (this.sentinelValues.containsZeroKey)
{
out.writeShort(EMPTY_KEY);
out.writeByte(this.sentinelValues.zeroValue);
}
if (this.sentinelValues.containsOneKey)
{
out.writeShort(REMOVED_KEY);
out.writeByte(this.sentinelValues.oneValue);
}
}
for (int i = 0; i < this.keys.length; i++)
{
if (isNonSentinel(this.keys[i]))
{
out.writeShort(this.keys[i]);
out.writeByte(this.values[i]);
}
}
}
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException
{
int size = in.readInt();
for (int i = 0; i < size; i++)
{
this.put(in.readShort(), in.readByte());
}
}
/**
* Rehashes every element in the set into a new backing table of the smallest possible size and eliminating removed sentinels.
*/
public void compact()
{
this.rehash(this.smallestPowerOfTwoGreaterThan(this.size()));
}
private void rehash()
{
this.rehash(this.keys.length);
}
private void rehashAndGrow()
{
this.rehash(this.keys.length << 1);
}
private void rehash(int newCapacity)
{
int oldLength = this.keys.length;
short[] old = this.keys;
byte[] oldValues = this.values;
this.allocateTable(newCapacity);
this.occupiedWithData = 0;
this.occupiedWithSentinels = 0;
for (int i = 0; i < oldLength; i++)
{
if (isNonSentinel(old[i]))
{
this.put(old[i], oldValues[i]);
}
}
}
// exposed for testing
int probe(short element)
{
int index = this.spread(element);
short keyAtIndex = this.keys[index];
if (keyAtIndex == element || keyAtIndex == EMPTY_KEY)
{
return index;
}
int removedIndex = keyAtIndex == REMOVED_KEY ? index : -1;
int nextIndex = index;
int probe = 17;
// loop until an empty slot is reached
while (true)
{
// Probe algorithm: 17*n*(n+1)/2 where n = number of collisions
nextIndex += probe;
probe += 17;
nextIndex &= this.keys.length - 1;
if (this.keys[nextIndex] == element)
{
return nextIndex;
}
if (this.keys[nextIndex] == REMOVED_KEY)
{
if (removedIndex == -1)
{
removedIndex = nextIndex;
}
}
else if (this.keys[nextIndex] == EMPTY_KEY)
{
return removedIndex == -1 ? nextIndex : removedIndex;
}
}
}
// exposed for testing
int spread(short element)
{
int code = (int) element;
code ^= 61 ^ (code >> 16);
code += code << 3;
code ^= code >> 4;
code *= 0x27d4eb2d;
code ^= code >> 15;
return code & (this.keys.length - 1);
}
private void allocateTable(int sizeToAllocate)
{
this.keys = new short[sizeToAllocate];
this.values = new byte[sizeToAllocate];
}
private static boolean isEmptyKey(short key)
{
return key == EMPTY_KEY;
}
private static boolean isRemovedKey(short key)
{
return key == REMOVED_KEY;
}
private static boolean isNonSentinel(short key)
{
return !isEmptyKey(key) && !isRemovedKey(key);
}
private int maxOccupiedWithData()
{
int capacity = this.keys.length;
// need at least one free slot for open addressing
return Math.min(capacity - 1, capacity / OCCUPIED_DATA_RATIO);
}
private int maxOccupiedWithSentinels()
{
return this.keys.length / OCCUPIED_SENTINEL_RATIO;
}
private static final class SentinelValues
{
private boolean containsZeroKey;
private boolean containsOneKey;
private byte zeroValue;
private byte oneValue;
public int size()
{
return (this.containsZeroKey ? 1 : 0) + (this.containsOneKey ? 1 : 0);
}
public boolean containsValue(byte value)
{
boolean valueEqualsZeroValue = this.containsZeroKey && this.zeroValue == value;
boolean valueEqualsOneValue = this.containsOneKey && this.oneValue == value;
return valueEqualsZeroValue || valueEqualsOneValue;
}
}
private class InternalByteIterator implements ByteIterator
{
private int count;
private int position;
private boolean handledZero;
private boolean handledOne;
public boolean hasNext()
{
return this.count < ShortByteHashMap.this.size();
}
public byte next()
{
if (!this.hasNext())
{
throw new NoSuchElementException("next() called, but the iterator is exhausted");
}
this.count++;
if (!this.handledZero)
{
this.handledZero = true;
if (ShortByteHashMap.this.containsKey(EMPTY_KEY))
{
return ShortByteHashMap.this.get(EMPTY_KEY);
}
}
if (!this.handledOne)
{
this.handledOne = true;
if (ShortByteHashMap.this.containsKey(REMOVED_KEY))
{
return ShortByteHashMap.this.get(REMOVED_KEY);
}
}
short[] keys = ShortByteHashMap.this.keys;
while (!isNonSentinel(keys[this.position]))
{
this.position++;
}
byte result = ShortByteHashMap.this.values[this.position];
this.position++;
return result;
}
}
private class KeysView extends AbstractLazyShortIterable
{
public ShortIterator shortIterator()
{
return new KeySetIterator();
}
public void forEach(ShortProcedure procedure)
{
ShortByteHashMap.this.forEachKey(procedure);
}
}
private class KeySetIterator implements ShortIterator
{
private int count;
private int position;
private boolean handledZero;
private boolean handledOne;
public boolean hasNext()
{
return this.count < ShortByteHashMap.this.size();
}
public short next()
{
if (!this.hasNext())
{
throw new NoSuchElementException("next() called, but the iterator is exhausted");
}
this.count++;
if (!this.handledZero)
{
this.handledZero = true;
if (ShortByteHashMap.this.containsKey(EMPTY_KEY))
{
return EMPTY_KEY;
}
}
if (!this.handledOne)
{
this.handledOne = true;
if (ShortByteHashMap.this.containsKey(REMOVED_KEY))
{
return REMOVED_KEY;
}
}
short[] keys = ShortByteHashMap.this.keys;
while (!isNonSentinel(keys[this.position]))
{
this.position++;
}
short result = keys[this.position];
this.position++;
return result;
}
}
public MutableShortSet keySet()
{
return new KeySet();
}
private class KeySet implements MutableShortSet
{
public ShortIterator shortIterator()
{
return new KeySetIterator();
}
public void forEach(ShortProcedure procedure)
{
ShortByteHashMap.this.forEachKey(procedure);
}
public int count(ShortPredicate predicate)
{
int count = 0;
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey && predicate.accept(EMPTY_KEY))
{
count++;
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey && predicate.accept(REMOVED_KEY))
{
count++;
}
}
for (short key : ShortByteHashMap.this.keys)
{
if (isNonSentinel(key) && predicate.accept(key))
{
count++;
}
}
return count;
}
public boolean anySatisfy(ShortPredicate predicate)
{
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey && predicate.accept(EMPTY_KEY))
{
return true;
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey && predicate.accept(REMOVED_KEY))
{
return true;
}
}
for (short key : ShortByteHashMap.this.keys)
{
if (isNonSentinel(key) && predicate.accept(key))
{
return true;
}
}
return false;
}
public boolean allSatisfy(ShortPredicate predicate)
{
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey && !predicate.accept(EMPTY_KEY))
{
return false;
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey && !predicate.accept(REMOVED_KEY))
{
return false;
}
}
for (short key : ShortByteHashMap.this.keys)
{
if (isNonSentinel(key) && !predicate.accept(key))
{
return false;
}
}
return true;
}
public boolean noneSatisfy(ShortPredicate predicate)
{
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey && predicate.accept(EMPTY_KEY))
{
return false;
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey && predicate.accept(REMOVED_KEY))
{
return false;
}
}
for (short key : ShortByteHashMap.this.keys)
{
if (isNonSentinel(key) && predicate.accept(key))
{
return false;
}
}
return true;
}
public boolean add(short element)
{
throw new UnsupportedOperationException("Cannot call add() on " + this.getClass().getSimpleName());
}
public boolean addAll(short... source)
{
throw new UnsupportedOperationException("Cannot call addAll() on " + this.getClass().getSimpleName());
}
public boolean addAll(ShortIterable source)
{
throw new UnsupportedOperationException("Cannot call addAll() on " + this.getClass().getSimpleName());
}
public boolean remove(short key)
{
int oldSize = ShortByteHashMap.this.size();
ShortByteHashMap.this.removeKey(key);
return oldSize != ShortByteHashMap.this.size();
}
public boolean removeAll(ShortIterable source)
{
int oldSize = ShortByteHashMap.this.size();
ShortIterator iterator = source.shortIterator();
while (iterator.hasNext())
{
ShortByteHashMap.this.removeKey(iterator.next());
}
return oldSize != ShortByteHashMap.this.size();
}
public boolean removeAll(short... source)
{
int oldSize = ShortByteHashMap.this.size();
for (short item : source)
{
ShortByteHashMap.this.removeKey(item);
}
return oldSize != ShortByteHashMap.this.size();
}
public boolean retainAll(ShortIterable source)
{
int oldSize = this.size();
final ShortSet sourceSet = source instanceof ShortSet ? (ShortSet) source : source.toSet();
ShortByteHashMap retained = ShortByteHashMap.this.select(new ShortBytePredicate()
{
public boolean accept(short key, byte value)
{
return sourceSet.contains(key);
}
});
if (retained.size() != oldSize)
{
ShortByteHashMap.this.keys = retained.keys;
ShortByteHashMap.this.values = retained.values;
ShortByteHashMap.this.sentinelValues = retained.sentinelValues;
ShortByteHashMap.this.occupiedWithData = retained.occupiedWithData;
ShortByteHashMap.this.occupiedWithSentinels = retained.occupiedWithSentinels;
return true;
}
return false;
}
public boolean retainAll(short... source)
{
return this.retainAll(ShortHashSet.newSetWith(source));
}
public void clear()
{
ShortByteHashMap.this.clear();
}
public MutableShortSet select(ShortPredicate predicate)
{
MutableShortSet result = new ShortHashSet();
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey && predicate.accept(EMPTY_KEY))
{
result.add(EMPTY_KEY);
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey && predicate.accept(REMOVED_KEY))
{
result.add(REMOVED_KEY);
}
}
for (short key : ShortByteHashMap.this.keys)
{
if (isNonSentinel(key) && predicate.accept(key))
{
result.add(key);
}
}
return result;
}
public MutableShortSet reject(ShortPredicate predicate)
{
MutableShortSet result = new ShortHashSet();
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey && !predicate.accept(EMPTY_KEY))
{
result.add(EMPTY_KEY);
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey && !predicate.accept(REMOVED_KEY))
{
result.add(REMOVED_KEY);
}
}
for (short key : ShortByteHashMap.this.keys)
{
if (isNonSentinel(key) && !predicate.accept(key))
{
result.add(key);
}
}
return result;
}
public MutableShortSet with(short element)
{
throw new UnsupportedOperationException("Cannot call with() on " + this.getClass().getSimpleName());
}
public MutableShortSet without(short element)
{
throw new UnsupportedOperationException("Cannot call without() on " + this.getClass().getSimpleName());
}
public MutableShortSet withAll(ShortIterable elements)
{
throw new UnsupportedOperationException("Cannot call withAll() on " + this.getClass().getSimpleName());
}
public MutableShortSet withoutAll(ShortIterable elements)
{
throw new UnsupportedOperationException("Cannot call withoutAll() on " + this.getClass().getSimpleName());
}
public short detectIfNone(ShortPredicate predicate, short ifNone)
{
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey && predicate.accept(EMPTY_KEY))
{
return EMPTY_KEY;
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey && predicate.accept(REMOVED_KEY))
{
return REMOVED_KEY;
}
}
for (short key : ShortByteHashMap.this.keys)
{
if (isNonSentinel(key) && predicate.accept(key))
{
return key;
}
}
return ifNone;
}
public MutableSet collect(ShortToObjectFunction extends V> function)
{
MutableSet result = Sets.mutable.with();
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey)
{
result.add(function.valueOf(EMPTY_KEY));
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey)
{
result.add(function.valueOf(REMOVED_KEY));
}
}
for (short key : ShortByteHashMap.this.keys)
{
if (isNonSentinel(key))
{
result.add(function.valueOf(key));
}
}
return result;
}
public MutableShortSet asUnmodifiable()
{
return UnmodifiableShortSet.of(this);
}
public MutableShortSet asSynchronized()
{
return SynchronizedShortSet.of(this);
}
public long sum()
{
long sum = 0L;
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey)
{
sum += EMPTY_KEY;
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey)
{
sum += REMOVED_KEY;
}
}
for (short key : ShortByteHashMap.this.keys)
{
if (isNonSentinel(key))
{
sum += key;
}
}
return sum;
}
public short max()
{
if (ShortByteHashMap.this.isEmpty())
{
throw new NoSuchElementException();
}
short max = 0;
boolean isMaxSet = false;
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey)
{
max = EMPTY_KEY;
isMaxSet = true;
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey && (!isMaxSet || max < REMOVED_KEY))
{
max = REMOVED_KEY;
isMaxSet = true;
}
}
for (int i = 0; i < ShortByteHashMap.this.keys.length; i++)
{
if (isNonSentinel(ShortByteHashMap.this.keys[i]) && (!isMaxSet || max < ShortByteHashMap.this.keys[i]))
{
max = ShortByteHashMap.this.keys[i];
isMaxSet = true;
}
}
return max;
}
public short maxIfEmpty(short defaultValue)
{
if (ShortByteHashMap.this.isEmpty())
{
return defaultValue;
}
return this.max();
}
public short min()
{
if (ShortByteHashMap.this.isEmpty())
{
throw new NoSuchElementException();
}
short min = 0;
boolean isMinSet = false;
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey)
{
min = EMPTY_KEY;
isMinSet = true;
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey && (!isMinSet || REMOVED_KEY < min))
{
min = REMOVED_KEY;
isMinSet = true;
}
}
for (int i = 0; i < ShortByteHashMap.this.keys.length; i++)
{
if (isNonSentinel(ShortByteHashMap.this.keys[i]) && (!isMinSet || ShortByteHashMap.this.keys[i] < min))
{
min = ShortByteHashMap.this.keys[i];
isMinSet = true;
}
}
return min;
}
public short minIfEmpty(short defaultValue)
{
if (ShortByteHashMap.this.isEmpty())
{
return defaultValue;
}
return this.min();
}
public double average()
{
if (this.isEmpty())
{
throw new ArithmeticException();
}
return (double) this.sum() / (double) this.size();
}
public double median()
{
if (this.isEmpty())
{
throw new ArithmeticException();
}
short[] sortedArray = this.toSortedArray();
int middleIndex = sortedArray.length >> 1;
if (sortedArray.length > 1 && (sortedArray.length & 1) == 0)
{
short first = sortedArray[middleIndex];
short second = sortedArray[middleIndex - 1];
return ((double) first + (double) second) / 2.0;
}
return (double) sortedArray[middleIndex];
}
public short[] toSortedArray()
{
short[] array = this.toArray();
Arrays.sort(array);
return array;
}
public MutableShortList toSortedList()
{
return ShortArrayList.newList(this).sortThis();
}
public short[] toArray()
{
int size = ShortByteHashMap.this.size();
final short[] result = new short[size];
ShortByteHashMap.this.forEachKey(new ShortProcedure()
{
private int index;
public void value(short each)
{
result[this.index] = each;
this.index++;
}
});
return result;
}
public boolean contains(short value)
{
return ShortByteHashMap.this.containsKey(value);
}
public boolean containsAll(short... source)
{
for (short item : source)
{
if (!ShortByteHashMap.this.containsKey(item))
{
return false;
}
}
return true;
}
public boolean containsAll(ShortIterable source)
{
ShortIterator iterator = source.shortIterator();
while (iterator.hasNext())
{
if (!ShortByteHashMap.this.containsKey(iterator.next()))
{
return false;
}
}
return true;
}
public MutableShortList toList()
{
return ShortArrayList.newList(this);
}
public MutableShortSet toSet()
{
return ShortHashSet.newSet(this);
}
public MutableShortBag toBag()
{
return ShortHashBag.newBag(this);
}
public LazyShortIterable asLazy()
{
return new LazyShortIterableAdapter(this);
}
public T injectInto(T injectedValue, ObjectShortToObjectFunction super T, ? extends T> function)
{
T result = injectedValue;
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey)
{
result = function.valueOf(result, EMPTY_KEY);
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey)
{
result = function.valueOf(result, REMOVED_KEY);
}
}
for (int i = 0; i < ShortByteHashMap.this.keys.length; i++)
{
if (isNonSentinel(ShortByteHashMap.this.keys[i]))
{
result = function.valueOf(result, ShortByteHashMap.this.keys[i]);
}
}
return result;
}
public ShortSet freeze()
{
throw new UnsupportedOperationException(this.getClass().getSimpleName() + ".freeze() not implemented yet");
}
public ImmutableShortSet toImmutable()
{
return ShortSets.immutable.withAll(this);
}
public int size()
{
return ShortByteHashMap.this.size();
}
public boolean isEmpty()
{
return ShortByteHashMap.this.isEmpty();
}
public boolean notEmpty()
{
return ShortByteHashMap.this.notEmpty();
}
@Override
public boolean equals(Object obj)
{
if (this == obj)
{
return true;
}
if (!(obj instanceof ShortSet))
{
return false;
}
ShortSet other = (ShortSet) obj;
return this.size() == other.size() && this.containsAll(other.toArray());
}
@Override
public int hashCode()
{
int result = 0;
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey)
{
result += (int) EMPTY_KEY;
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey)
{
result += (int) REMOVED_KEY;
}
}
for (int i = 0; i < ShortByteHashMap.this.keys.length; i++)
{
if (isNonSentinel(ShortByteHashMap.this.keys[i]))
{
result += (int) ShortByteHashMap.this.keys[i];
}
}
return result;
}
@Override
public String toString()
{
return this.makeString("[", ", ", "]");
}
public String makeString()
{
return this.makeString(", ");
}
public String makeString(String separator)
{
return this.makeString("", separator, "");
}
public String makeString(String start, String separator, String end)
{
Appendable stringBuilder = new StringBuilder();
this.appendString(stringBuilder, start, separator, end);
return stringBuilder.toString();
}
public void appendString(Appendable appendable)
{
this.appendString(appendable, ", ");
}
public void appendString(Appendable appendable, String separator)
{
this.appendString(appendable, "", separator, "");
}
public void appendString(Appendable appendable, String start, String separator, String end)
{
try
{
appendable.append(start);
boolean first = true;
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey)
{
appendable.append(String.valueOf(EMPTY_KEY));
first = false;
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey)
{
if (!first)
{
appendable.append(separator);
}
appendable.append(String.valueOf(REMOVED_KEY));
first = false;
}
}
for (short key : ShortByteHashMap.this.keys)
{
if (isNonSentinel(key))
{
if (!first)
{
appendable.append(separator);
}
appendable.append(String.valueOf(key));
first = false;
}
}
appendable.append(end);
}
catch (IOException e)
{
throw new RuntimeException(e);
}
}
}
public MutableByteCollection values()
{
return new ValuesCollection();
}
private class ValuesCollection implements MutableByteCollection
{
public void clear()
{
ShortByteHashMap.this.clear();
}
public MutableByteCollection select(BytePredicate predicate)
{
return ShortByteHashMap.this.select(predicate);
}
public MutableByteCollection reject(BytePredicate predicate)
{
return ShortByteHashMap.this.reject(predicate);
}
public byte detectIfNone(BytePredicate predicate, byte ifNone)
{
return ShortByteHashMap.this.detectIfNone(predicate, ifNone);
}
public MutableCollection collect(ByteToObjectFunction extends V> function)
{
return ShortByteHashMap.this.collect(function);
}
public T injectInto(T injectedValue, ObjectByteToObjectFunction super T, ? extends T> function)
{
return ShortByteHashMap.this.injectInto(injectedValue, function);
}
public long sum()
{
return ShortByteHashMap.this.sum();
}
public byte max()
{
return ShortByteHashMap.this.max();
}
public byte maxIfEmpty(byte defaultValue)
{
return ShortByteHashMap.this.maxIfEmpty(defaultValue);
}
public byte min()
{
return ShortByteHashMap.this.min();
}
public byte minIfEmpty(byte defaultValue)
{
return ShortByteHashMap.this.minIfEmpty(defaultValue);
}
public double average()
{
return ShortByteHashMap.this.average();
}
public double median()
{
return ShortByteHashMap.this.median();
}
public byte[] toSortedArray()
{
return ShortByteHashMap.this.toSortedArray();
}
public MutableByteList toSortedList()
{
return ShortByteHashMap.this.toSortedList();
}
public MutableByteCollection with(byte element)
{
throw new UnsupportedOperationException("Cannot call with() on " + this.getClass().getSimpleName());
}
public MutableByteCollection without(byte element)
{
throw new UnsupportedOperationException("Cannot call without() on " + this.getClass().getSimpleName());
}
public MutableByteCollection withAll(ByteIterable elements)
{
throw new UnsupportedOperationException("Cannot call withAll() on " + this.getClass().getSimpleName());
}
public MutableByteCollection withoutAll(ByteIterable elements)
{
throw new UnsupportedOperationException("Cannot call withoutAll() on " + this.getClass().getSimpleName());
}
public MutableByteCollection asUnmodifiable()
{
return UnmodifiableByteCollection.of(this);
}
public MutableByteCollection asSynchronized()
{
return SynchronizedByteCollection.of(this);
}
public ImmutableByteCollection toImmutable()
{
return ByteLists.immutable.withAll(this);
}
public boolean contains(byte value)
{
return ShortByteHashMap.this.containsValue(value);
}
public boolean containsAll(byte... source)
{
return ShortByteHashMap.this.containsAll(source);
}
public boolean containsAll(ByteIterable source)
{
return ShortByteHashMap.this.containsAll(source);
}
public MutableByteList toList()
{
return ShortByteHashMap.this.toList();
}
public MutableByteSet toSet()
{
return ShortByteHashMap.this.toSet();
}
public MutableByteBag toBag()
{
return ShortByteHashMap.this.toBag();
}
public LazyByteIterable asLazy()
{
return new LazyByteIterableAdapter(this);
}
public boolean isEmpty()
{
return ShortByteHashMap.this.isEmpty();
}
public boolean notEmpty()
{
return ShortByteHashMap.this.notEmpty();
}
public String makeString()
{
return this.makeString(", ");
}
public String makeString(String separator)
{
return this.makeString("", separator, "");
}
public String makeString(String start, String separator, String end)
{
Appendable stringBuilder = new StringBuilder();
this.appendString(stringBuilder, start, separator, end);
return stringBuilder.toString();
}
public void appendString(Appendable appendable)
{
this.appendString(appendable, ", ");
}
public void appendString(Appendable appendable, String separator)
{
this.appendString(appendable, "", separator, "");
}
public void appendString(Appendable appendable, String start, String separator, String end)
{
try
{
appendable.append(start);
boolean first = true;
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey)
{
appendable.append(String.valueOf(ShortByteHashMap.this.sentinelValues.zeroValue));
first = false;
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey)
{
if (!first)
{
appendable.append(separator);
}
appendable.append(String.valueOf(ShortByteHashMap.this.sentinelValues.oneValue));
first = false;
}
}
for (int i = 0; i < ShortByteHashMap.this.keys.length; i++)
{
short key = ShortByteHashMap.this.keys[i];
if (isNonSentinel(key))
{
if (!first)
{
appendable.append(separator);
}
appendable.append(String.valueOf(ShortByteHashMap.this.values[i]));
first = false;
}
}
appendable.append(end);
}
catch (IOException e)
{
throw new RuntimeException(e);
}
}
public ByteIterator byteIterator()
{
return ShortByteHashMap.this.byteIterator();
}
public void forEach(ByteProcedure procedure)
{
ShortByteHashMap.this.forEach(procedure);
}
public int count(BytePredicate predicate)
{
return ShortByteHashMap.this.count(predicate);
}
public boolean anySatisfy(BytePredicate predicate)
{
return ShortByteHashMap.this.anySatisfy(predicate);
}
public boolean allSatisfy(BytePredicate predicate)
{
return ShortByteHashMap.this.allSatisfy(predicate);
}
public boolean noneSatisfy(BytePredicate predicate)
{
return ShortByteHashMap.this.noneSatisfy(predicate);
}
public boolean add(byte element)
{
throw new UnsupportedOperationException("Cannot call add() on " + this.getClass().getSimpleName());
}
public boolean addAll(byte... source)
{
throw new UnsupportedOperationException("Cannot call addAll() on " + this.getClass().getSimpleName());
}
public boolean addAll(ByteIterable source)
{
throw new UnsupportedOperationException("Cannot call addAll() on " + this.getClass().getSimpleName());
}
public boolean remove(byte item)
{
int oldSize = ShortByteHashMap.this.size();
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey && item == ShortByteHashMap.this.sentinelValues.zeroValue)
{
ShortByteHashMap.this.removeKey(EMPTY_KEY);
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey && item == ShortByteHashMap.this.sentinelValues.oneValue)
{
ShortByteHashMap.this.removeKey(REMOVED_KEY);
}
}
for (int i = 0; i < ShortByteHashMap.this.keys.length; i++)
{
if (isNonSentinel(ShortByteHashMap.this.keys[i]) && item == ShortByteHashMap.this.values[i])
{
ShortByteHashMap.this.removeKey(ShortByteHashMap.this.keys[i]);
}
}
return oldSize != ShortByteHashMap.this.size();
}
public boolean removeAll(ByteIterable source)
{
int oldSize = ShortByteHashMap.this.size();
ByteIterator iterator = source.byteIterator();
while (iterator.hasNext())
{
this.remove(iterator.next());
}
return oldSize != ShortByteHashMap.this.size();
}
public boolean removeAll(byte... source)
{
int oldSize = ShortByteHashMap.this.size();
for (byte item : source)
{
this.remove(item);
}
return oldSize != ShortByteHashMap.this.size();
}
public boolean retainAll(ByteIterable source)
{
int oldSize = this.size();
final ByteSet sourceSet = source instanceof ByteSet ? (ByteSet) source : source.toSet();
ShortByteHashMap retained = ShortByteHashMap.this.select(new ShortBytePredicate()
{
public boolean accept(short key, byte value)
{
return sourceSet.contains(value);
}
});
if (retained.size() != oldSize)
{
ShortByteHashMap.this.keys = retained.keys;
ShortByteHashMap.this.values = retained.values;
ShortByteHashMap.this.sentinelValues = retained.sentinelValues;
ShortByteHashMap.this.occupiedWithData = retained.occupiedWithData;
ShortByteHashMap.this.occupiedWithSentinels = retained.occupiedWithSentinels;
return true;
}
return false;
}
public boolean retainAll(byte... source)
{
return this.retainAll(ByteHashSet.newSetWith(source));
}
public int size()
{
return ShortByteHashMap.this.size();
}
public byte[] toArray()
{
return ShortByteHashMap.this.toArray();
}
}
private class KeyValuesView extends AbstractLazyIterable
{
public void forEach(Procedure super ShortBytePair> procedure)
{
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey)
{
procedure.value(PrimitiveTuples.pair(EMPTY_KEY, ShortByteHashMap.this.sentinelValues.zeroValue));
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey)
{
procedure.value(PrimitiveTuples.pair(REMOVED_KEY, ShortByteHashMap.this.sentinelValues.oneValue));
}
}
for (int i = 0; i < ShortByteHashMap.this.keys.length; i++)
{
if (isNonSentinel(ShortByteHashMap.this.keys[i]))
{
procedure.value(PrimitiveTuples.pair(ShortByteHashMap.this.keys[i], ShortByteHashMap.this.values[i]));
}
}
}
public void forEachWithIndex(ObjectIntProcedure super ShortBytePair> objectIntProcedure)
{
int index = 0;
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey)
{
objectIntProcedure.value(PrimitiveTuples.pair(EMPTY_KEY, ShortByteHashMap.this.sentinelValues.zeroValue), index);
index++;
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey)
{
objectIntProcedure.value(PrimitiveTuples.pair(REMOVED_KEY, ShortByteHashMap.this.sentinelValues.oneValue), index);
index++;
}
}
for (int i = 0; i < ShortByteHashMap.this.keys.length; i++)
{
if (isNonSentinel(ShortByteHashMap.this.keys[i]))
{
objectIntProcedure.value(PrimitiveTuples.pair(ShortByteHashMap.this.keys[i], ShortByteHashMap.this.values[i]), index);
index++;
}
}
}
public void forEachWith(Procedure2 super ShortBytePair, ? super P> procedure, P parameter)
{
if (ShortByteHashMap.this.sentinelValues != null)
{
if (ShortByteHashMap.this.sentinelValues.containsZeroKey)
{
procedure.value(PrimitiveTuples.pair(EMPTY_KEY, ShortByteHashMap.this.sentinelValues.zeroValue), parameter);
}
if (ShortByteHashMap.this.sentinelValues.containsOneKey)
{
procedure.value(PrimitiveTuples.pair(REMOVED_KEY, ShortByteHashMap.this.sentinelValues.oneValue), parameter);
}
}
for (int i = 0; i < ShortByteHashMap.this.keys.length; i++)
{
if (isNonSentinel(ShortByteHashMap.this.keys[i]))
{
procedure.value(PrimitiveTuples.pair(ShortByteHashMap.this.keys[i], ShortByteHashMap.this.values[i]), parameter);
}
}
}
public Iterator iterator()
{
return new InternalKeyValuesIterator();
}
public class InternalKeyValuesIterator implements Iterator
{
private int count;
private int position;
private boolean handledZero;
private boolean handledOne;
public ShortBytePair next()
{
if (!this.hasNext())
{
throw new NoSuchElementException("next() called, but the iterator is exhausted");
}
this.count++;
if (!this.handledZero)
{
this.handledZero = true;
if (ShortByteHashMap.this.containsKey(EMPTY_KEY))
{
return PrimitiveTuples.pair(EMPTY_KEY, ShortByteHashMap.this.sentinelValues.zeroValue);
}
}
if (!this.handledOne)
{
this.handledOne = true;
if (ShortByteHashMap.this.containsKey(REMOVED_KEY))
{
return PrimitiveTuples.pair(REMOVED_KEY, ShortByteHashMap.this.sentinelValues.oneValue);
}
}
short[] keys = ShortByteHashMap.this.keys;
while (!isNonSentinel(keys[this.position]))
{
this.position++;
}
ShortBytePair result = PrimitiveTuples.pair(keys[this.position], ShortByteHashMap.this.values[this.position]);
this.position++;
return result;
}
public void remove()
{
throw new UnsupportedOperationException("Cannot call remove() on " + this.getClass().getSimpleName());
}
public boolean hasNext()
{
return this.count != ShortByteHashMap.this.size();
}
}
}
}