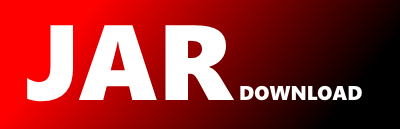
com.gs.collections.impl.parallel.ObjectIntProcedureFJTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gs-collections Show documentation
Show all versions of gs-collections Show documentation
GS Collections is a collections framework for Java. It has JDK-compatible List, Set and Map
implementations with a rich API and set of utility classes that work with any JDK compatible Collections,
Arrays, Maps or Strings. The iteration protocol was inspired by the Smalltalk collection framework.
/*
* Copyright 2011 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.parallel;
import java.util.ArrayList;
import java.util.List;
import com.gs.collections.api.block.procedure.primitive.ObjectIntProcedure;
import com.gs.collections.api.list.MutableList;
import com.gs.collections.impl.utility.ArrayListIterate;
import com.gs.collections.impl.utility.ListIterate;
public final class ObjectIntProcedureFJTask> implements Runnable
{
private final ObjectIntProcedureFactory procedureFactory;
private BT procedure;
private final List list;
private final int start;
private final int end;
private final ObjectIntProcedureFJTaskRunner taskRunner;
/**
* Creates an array of ProcedureFJTasks wrapping Procedures created by the specified ProcedureFactory.
*/
public ObjectIntProcedureFJTask(
ObjectIntProcedureFJTaskRunner newFJTaskRunner,
ObjectIntProcedureFactory newProcedureFactory,
List list,
int index,
int sectionSize,
boolean isLast)
{
this.taskRunner = newFJTaskRunner;
this.procedureFactory = newProcedureFactory;
this.list = list;
this.start = index * sectionSize;
this.end = isLast ? this.list.size() : this.start + sectionSize;
}
public void run()
{
try
{
this.procedure = this.procedureFactory.create();
int stop = this.end - 1;
if (this.list instanceof MutableList)
{
((MutableList) this.list).forEachWithIndex(this.start, stop, this.procedure);
}
else if (this.list instanceof ArrayList)
{
ArrayListIterate.forEachWithIndex((ArrayList) this.list, this.start, stop, this.procedure);
}
else
{
ListIterate.forEachWithIndex(this.list, this.start, stop, this.procedure);
}
}
catch (Throwable newError)
{
this.taskRunner.setFailed(newError);
}
finally
{
this.taskRunner.taskCompleted(this);
}
}
public BT getProcedure()
{
return this.procedure;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy