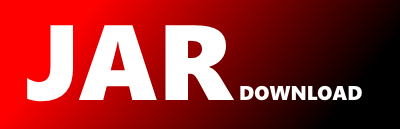
com.gs.collections.impl.parallel.ParallelMapIterate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gs-collections Show documentation
Show all versions of gs-collections Show documentation
GS Collections is a collections framework for Java. It has JDK-compatible List, Set and Map
implementations with a rich API and set of utility classes that work with any JDK compatible Collections,
Arrays, Maps or Strings. The iteration protocol was inspired by the Smalltalk collection framework.
/*
* Copyright 2011 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.parallel;
import java.util.Map;
import java.util.concurrent.Executor;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.api.block.procedure.Procedure2;
import com.gs.collections.api.tuple.Pair;
import com.gs.collections.impl.utility.MapIterate;
/**
* The ParallelMapIterate class contains parallel algorithms that work with Maps.
*
* The forEachEtry algorithm employs a batching fork and join approach approach which does
* not yet allow for specification of a Factory for the blocks or a Combiner for the results.
* This means that forEachKeyValue can only support pure forking or forking with a shared
* thread-safe data structure collecting results.
*/
public final class ParallelMapIterate
{
private ParallelMapIterate()
{
throw new AssertionError("Suppress default constructor for noninstantiability");
}
/**
* A parallel form of forEachKeyValue.
*
* @see MapIterate#forEachKeyValue(Map, Procedure2)
* @see ParallelIterate
*/
public static void forEachKeyValue(Map map, Procedure2 super K, ? super V> procedure2)
{
ParallelMapIterate.forEachKeyValue(map, procedure2, 2, map.size());
}
/**
* A parallel form of forEachKeyValue.
*
* @see MapIterate#forEachKeyValue(Map, Procedure2)
* @see ParallelIterate
*/
public static void forEachKeyValue(
Map map,
Procedure2 super K, ? super V> procedure,
Executor executor)
{
ParallelMapIterate.forEachKeyValue(map, procedure, 2, map.size(), executor);
}
/**
* A parallel form of forEachKeyValue.
*
* @see MapIterate#forEachKeyValue(Map, Procedure2)
* @see ParallelIterate
*/
public static void forEachKeyValue(
Map map,
Procedure2 super K, ? super V> procedure,
int minForkSize,
int taskCount)
{
if (map.size() > minForkSize)
{
Procedure> pairProcedure = new PairProcedure(procedure);
ParallelIterate.forEach(MapIterate.toListOfPairs(map), new PassThruProcedureFactory>>(pairProcedure), new PassThruCombiner>>(), minForkSize, taskCount);
}
else
{
MapIterate.forEachKeyValue(map, procedure);
}
}
/**
* A parallel form of forEachKeyValue.
*
* @see MapIterate#forEachKeyValue(Map, Procedure2)
* @see ParallelIterate
*/
public static void forEachKeyValue(
Map map,
Procedure2 super K, ? super V> procedure,
int minForkSize,
int taskCount,
Executor executor)
{
if (map.size() > minForkSize)
{
Procedure> pairProcedure = new PairProcedure(procedure);
ParallelIterate.forEachInListOnExecutor(
MapIterate.toListOfPairs(map),
new PassThruProcedureFactory>>(pairProcedure),
new PassThruCombiner>>(),
minForkSize,
taskCount,
executor);
}
else
{
MapIterate.forEachKeyValue(map, procedure);
}
}
private static final class PairProcedure implements Procedure>
{
private static final long serialVersionUID = 1L;
private final Procedure2 super T1, ? super T2> procedure;
private PairProcedure(Procedure2 super T1, ? super T2> procedure)
{
this.procedure = procedure;
}
public void value(Pair pair)
{
this.procedure.value(pair.getOne(), pair.getTwo());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy