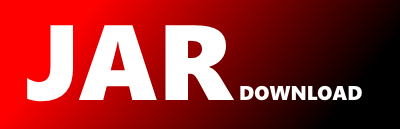
com.gs.collections.impl.set.mutable.SynchronizedMutableSet Maven / Gradle / Ivy
Show all versions of gs-collections Show documentation
/*
* Copyright 2011 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.set.mutable;
import java.io.Serializable;
import java.util.Collection;
import java.util.Collections;
import java.util.Set;
import com.gs.collections.api.LazyIterable;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.function.Function2;
import com.gs.collections.api.block.function.primitive.BooleanFunction;
import com.gs.collections.api.block.function.primitive.ByteFunction;
import com.gs.collections.api.block.function.primitive.CharFunction;
import com.gs.collections.api.block.function.primitive.DoubleFunction;
import com.gs.collections.api.block.function.primitive.FloatFunction;
import com.gs.collections.api.block.function.primitive.IntFunction;
import com.gs.collections.api.block.function.primitive.LongFunction;
import com.gs.collections.api.block.function.primitive.ShortFunction;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.multimap.set.MutableSetMultimap;
import com.gs.collections.api.partition.set.PartitionMutableSet;
import com.gs.collections.api.set.ImmutableSet;
import com.gs.collections.api.set.MutableSet;
import com.gs.collections.api.set.SetIterable;
import com.gs.collections.api.set.UnsortedSetIterable;
import com.gs.collections.api.set.primitive.MutableBooleanSet;
import com.gs.collections.api.set.primitive.MutableByteSet;
import com.gs.collections.api.set.primitive.MutableCharSet;
import com.gs.collections.api.set.primitive.MutableDoubleSet;
import com.gs.collections.api.set.primitive.MutableFloatSet;
import com.gs.collections.api.set.primitive.MutableIntSet;
import com.gs.collections.api.set.primitive.MutableLongSet;
import com.gs.collections.api.set.primitive.MutableShortSet;
import com.gs.collections.api.tuple.Pair;
import com.gs.collections.impl.collection.mutable.AbstractSynchronizedMutableCollection;
import com.gs.collections.impl.collection.mutable.SynchronizedCollectionSerializationProxy;
import com.gs.collections.impl.factory.Sets;
import net.jcip.annotations.GuardedBy;
import net.jcip.annotations.ThreadSafe;
/**
* A synchronized view of a {@link MutableSet}. It is imperative that the user manually synchronize on the collection when iterating over it using the
* standard JDK iterator or JDK 5 for loop, as per {@link Collections#synchronizedCollection(Collection)}.
*
* @see MutableSet#asSynchronized()
*/
@ThreadSafe
public class SynchronizedMutableSet
extends AbstractSynchronizedMutableCollection
implements MutableSet, Serializable
{
SynchronizedMutableSet(MutableSet set)
{
super(set);
}
SynchronizedMutableSet(MutableSet set, Object newLock)
{
super(set, newLock);
}
/**
* This method will take a MutableSet and wrap it directly in a SynchronizedMutableSet. It will
* take any other non-GS-collection and first adapt it will a SetAdapter, and then return a
* SynchronizedMutableSet that wraps the adapter.
*/
public static > SynchronizedMutableSet of(S set)
{
return new SynchronizedMutableSet(SetAdapter.adapt(set));
}
/**
* This method will take a MutableSet and wrap it directly in a SynchronizedMutableSet. It will
* take any other non-GS-collection and first adapt it will a SetAdapter, and then return a
* SynchronizedMutableSet that wraps the adapter. Additionally, a developer specifies which lock to use
* with the collection.
*/
public static > SynchronizedMutableSet of(S set, Object lock)
{
return new SynchronizedMutableSet(SetAdapter.adapt(set), lock);
}
@GuardedBy("getLock()")
private MutableSet getMutableSet()
{
return (MutableSet) this.getCollection();
}
@Override
public MutableSet asUnmodifiable()
{
synchronized (this.getLock())
{
return UnmodifiableMutableSet.of(this);
}
}
@Override
public ImmutableSet toImmutable()
{
synchronized (this.getLock())
{
return Sets.immutable.ofAll(this.getMutableSet());
}
}
@Override
public MutableSet asSynchronized()
{
return this;
}
@Override
public MutableSet clone()
{
synchronized (this.getLock())
{
return of(this.getMutableSet().clone());
}
}
@Override
public MutableSet collect(Function super T, ? extends V> function)
{
synchronized (this.getLock())
{
return this.getMutableSet().collect(function);
}
}
@Override
public MutableBooleanSet collectBoolean(BooleanFunction super T> booleanFunction)
{
synchronized (this.getLock())
{
return this.getMutableSet().collectBoolean(booleanFunction);
}
}
@Override
public MutableByteSet collectByte(ByteFunction super T> byteFunction)
{
synchronized (this.getLock())
{
return this.getMutableSet().collectByte(byteFunction);
}
}
@Override
public MutableCharSet collectChar(CharFunction super T> charFunction)
{
synchronized (this.getLock())
{
return this.getMutableSet().collectChar(charFunction);
}
}
@Override
public MutableDoubleSet collectDouble(DoubleFunction super T> doubleFunction)
{
synchronized (this.getLock())
{
return this.getMutableSet().collectDouble(doubleFunction);
}
}
@Override
public MutableFloatSet collectFloat(FloatFunction super T> floatFunction)
{
synchronized (this.getLock())
{
return this.getMutableSet().collectFloat(floatFunction);
}
}
@Override
public MutableIntSet collectInt(IntFunction super T> intFunction)
{
synchronized (this.getLock())
{
return this.getMutableSet().collectInt(intFunction);
}
}
@Override
public MutableLongSet collectLong(LongFunction super T> longFunction)
{
synchronized (this.getLock())
{
return this.getMutableSet().collectLong(longFunction);
}
}
@Override
public MutableShortSet collectShort(ShortFunction super T> shortFunction)
{
synchronized (this.getLock())
{
return this.getMutableSet().collectShort(shortFunction);
}
}
@Override
public MutableSet flatCollect(Function super T, ? extends Iterable> function)
{
synchronized (this.getLock())
{
return this.getMutableSet().flatCollect(function);
}
}
@Override
public MutableSet collectIf(
Predicate super T> predicate,
Function super T, ? extends V> function)
{
synchronized (this.getLock())
{
return this.getMutableSet().collectIf(predicate, function);
}
}
@Override
public MutableSet collectWith(Function2 super T, ? super P, ? extends V> function, P parameter)
{
synchronized (this.getLock())
{
return this.getMutableSet().collectWith(function, parameter);
}
}
@Override
public MutableSetMultimap groupBy(Function super T, ? extends V> function)
{
synchronized (this.getLock())
{
return this.getMutableSet().groupBy(function);
}
}
@Override
public MutableSetMultimap groupByEach(Function super T, ? extends Iterable> function)
{
synchronized (this.getLock())
{
return this.getMutableSet().groupByEach(function);
}
}
@Override
public MutableSet newEmpty()
{
synchronized (this.getLock())
{
return this.getMutableSet().newEmpty();
}
}
@Override
public MutableSet reject(Predicate super T> predicate)
{
synchronized (this.getLock())
{
return this.getMutableSet().reject(predicate);
}
}
@Override
public MutableSet rejectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.getLock())
{
return this.getMutableSet().rejectWith(predicate, parameter);
}
}
@Override
public MutableSet select(Predicate super T> predicate)
{
synchronized (this.getLock())
{
return this.getMutableSet().select(predicate);
}
}
@Override
public MutableSet selectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.getLock())
{
return this.getMutableSet().selectWith(predicate, parameter);
}
}
@Override
public PartitionMutableSet partition(Predicate super T> predicate)
{
synchronized (this.getLock())
{
return this.getMutableSet().partition(predicate);
}
}
@Override
public PartitionMutableSet partitionWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.getLock())
{
return this.getMutableSet().partitionWith(predicate, parameter);
}
}
@Override
public MutableSet selectInstancesOf(Class clazz)
{
synchronized (this.getLock())
{
return this.getMutableSet().selectInstancesOf(clazz);
}
}
@Override
public boolean equals(Object obj)
{
synchronized (this.getLock())
{
return this.getMutableSet().equals(obj);
}
}
@Override
public int hashCode()
{
synchronized (this.getLock())
{
return this.getMutableSet().hashCode();
}
}
@Override
public MutableSet> zip(Iterable that)
{
synchronized (this.getLock())
{
return this.getMutableSet().zip(that);
}
}
@Override
public >> R zip(Iterable that, R target)
{
synchronized (this.getLock())
{
return this.getMutableSet().zip(that, target);
}
}
@Override
public MutableSet> zipWithIndex()
{
synchronized (this.getLock())
{
return this.getMutableSet().zipWithIndex();
}
}
@Override
public >> R zipWithIndex(R target)
{
synchronized (this.getLock())
{
return this.getMutableSet().zipWithIndex(target);
}
}
public MutableSet union(SetIterable extends T> set)
{
synchronized (this.getLock())
{
return this.getMutableSet().union(set);
}
}
public > R unionInto(SetIterable extends T> set, R targetSet)
{
synchronized (this.getLock())
{
return this.getMutableSet().unionInto(set, targetSet);
}
}
public MutableSet intersect(SetIterable extends T> set)
{
synchronized (this.getLock())
{
return this.getMutableSet().intersect(set);
}
}
public > R intersectInto(SetIterable extends T> set, R targetSet)
{
synchronized (this.getLock())
{
return this.getMutableSet().intersectInto(set, targetSet);
}
}
public MutableSet difference(SetIterable extends T> subtrahendSet)
{
synchronized (this.getLock())
{
return this.getMutableSet().difference(subtrahendSet);
}
}
public > R differenceInto(SetIterable extends T> subtrahendSet, R targetSet)
{
synchronized (this.getLock())
{
return this.getMutableSet().differenceInto(subtrahendSet, targetSet);
}
}
public MutableSet symmetricDifference(SetIterable extends T> setB)
{
synchronized (this.getLock())
{
return this.getMutableSet().symmetricDifference(setB);
}
}
public > R symmetricDifferenceInto(SetIterable extends T> set, R targetSet)
{
synchronized (this.getLock())
{
return this.getMutableSet().symmetricDifferenceInto(set, targetSet);
}
}
public boolean isSubsetOf(SetIterable extends T> candidateSuperset)
{
synchronized (this.getLock())
{
return this.getMutableSet().isSubsetOf(candidateSuperset);
}
}
public boolean isProperSubsetOf(SetIterable extends T> candidateSuperset)
{
synchronized (this.getLock())
{
return this.getMutableSet().isProperSubsetOf(candidateSuperset);
}
}
public MutableSet> powerSet()
{
synchronized (this.getLock())
{
return this.getMutableSet().powerSet();
}
}
public LazyIterable> cartesianProduct(SetIterable set)
{
synchronized (this.getLock())
{
return this.getMutableSet().cartesianProduct(set);
}
}
@Override
public MutableSet with(T element)
{
this.add(element);
return this;
}
@Override
public MutableSet without(T element)
{
this.remove(element);
return this;
}
@Override
public MutableSet withAll(Iterable extends T> elements)
{
this.addAllIterable(elements);
return this;
}
@Override
public MutableSet withoutAll(Iterable extends T> elements)
{
this.removeAllIterable(elements);
return this;
}
protected Object writeReplace()
{
return new SynchronizedCollectionSerializationProxy(this.getMutableSet());
}
}