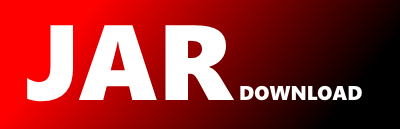
com.gs.collections.impl.utility.internal.ReflectionHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gs-collections Show documentation
Show all versions of gs-collections Show documentation
GS Collections is a collections framework for Java. It has JDK-compatible List, Set and Map
implementations with a rich API and set of utility classes that work with any JDK compatible Collections,
Arrays, Maps or Strings. The iteration protocol was inspired by the Smalltalk collection framework.
/*
* Copyright 2011 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.utility.internal;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Modifier;
import com.gs.collections.api.map.ImmutableMap;
import com.gs.collections.impl.map.mutable.UnifiedMap;
import com.gs.collections.impl.tuple.Tuples;
import com.gs.collections.impl.utility.MapIterate;
/**
* A utility/helper class for working with Classes and Reflection.
*/
public final class ReflectionHelper
{
/**
* @deprecated in 2.0. Will become private in a future version.
*/
@SuppressWarnings("rawtypes")
@Deprecated
public static final Class[] EMPTY_CLASS_ARRAY = {};
/**
* Mapping of iterator wrapper classes to iterator types
*/
private static final ImmutableMap, Class>> WRAPPER_TO_PRIMATIVES = UnifiedMap.newMapWith(
Tuples.>twin(Short.class, short.class),
Tuples.>twin(Boolean.class, boolean.class),
Tuples.>twin(Byte.class, byte.class),
Tuples.>twin(Character.class, char.class),
Tuples.>twin(Integer.class, int.class),
Tuples.>twin(Float.class, float.class),
Tuples.>twin(Long.class, long.class),
Tuples.>twin(Double.class, double.class)).toImmutable();
private static final ImmutableMap, Class>> PRIMATIVES_TO_WRAPPERS = MapIterate.reverseMapping(WRAPPER_TO_PRIMATIVES.castToMap()).toImmutable();
private ReflectionHelper()
{
throw new AssertionError("Suppress default constructor for noninstantiability");
}
// These are special methods that will not produce error messages if the getter method is not found
public static Constructor getConstructor(Class instantiable, Class>... constructorParameterTypes)
{
try
{
return instantiable.getConstructor(constructorParameterTypes);
}
catch (NoSuchMethodException ignored)
{
return ReflectionHelper.searchForConstructor(instantiable, constructorParameterTypes);
}
}
private static Constructor searchForConstructor(Class instantiable, Class>... constructorParameterTypes)
{
Constructor>[] candidates = instantiable.getConstructors();
for (Constructor> candidate : candidates)
{
if (ReflectionHelper.parameterTypesMatch(candidate.getParameterTypes(), constructorParameterTypes))
{
return (Constructor) candidate;
}
}
return null;
}
public static boolean parameterTypesMatch(Class>[] candidateParamTypes, Class>... desiredParameterTypes)
{
boolean match = candidateParamTypes.length == desiredParameterTypes.length;
for (int i = 0; i < candidateParamTypes.length && match; i++)
{
Class> candidateType = candidateParamTypes[i].isPrimitive() && !desiredParameterTypes[i].isPrimitive()
? PRIMATIVES_TO_WRAPPERS.get(candidateParamTypes[i])
: candidateParamTypes[i];
match = candidateType.isAssignableFrom(desiredParameterTypes[i]);
}
return match;
}
public static T newInstance(Constructor constructor, Object... constructorArguments)
{
try
{
return constructor.newInstance(constructorArguments);
}
catch (InstantiationException e)
{
throw new RuntimeException(e);
}
catch (IllegalAccessException e)
{
throw new RuntimeException(e);
}
catch (InvocationTargetException e)
{
throw new RuntimeException(e);
}
}
/**
* This method may return null if the call to create a newInstance() fails.
*/
public static T newInstance(Class aClass)
{
try
{
return aClass.getConstructor().newInstance();
}
catch (InstantiationException e)
{
throw new RuntimeException(e);
}
catch (IllegalAccessException e)
{
throw new RuntimeException(e);
}
catch (NoSuchMethodException e)
{
throw new RuntimeException(e);
}
catch (InvocationTargetException e)
{
throw new RuntimeException(e);
}
}
public static boolean hasDefaultConstructor(Class> aClass)
{
try
{
Constructor> constructor = aClass.getDeclaredConstructor(EMPTY_CLASS_ARRAY);
return Modifier.isPublic(constructor.getModifiers());
}
catch (NoSuchMethodException ignored)
{
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy