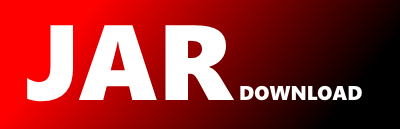
com.gs.collections.impl.bag.mutable.HashBag Maven / Gradle / Ivy
Show all versions of gs-collections Show documentation
/*
* Copyright 2015 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.bag.mutable;
import java.io.Externalizable;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.Arrays;
import java.util.Collection;
import java.util.Iterator;
import com.gs.collections.api.RichIterable;
import com.gs.collections.api.bag.Bag;
import com.gs.collections.api.bag.MutableBag;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.block.predicate.primitive.IntPredicate;
import com.gs.collections.api.block.predicate.primitive.ObjectIntPredicate;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.api.block.procedure.Procedure2;
import com.gs.collections.api.block.procedure.primitive.ObjectIntProcedure;
import com.gs.collections.api.map.MutableMap;
import com.gs.collections.api.map.primitive.MutableObjectIntMap;
import com.gs.collections.api.tuple.primitive.ObjectIntPair;
import com.gs.collections.impl.Counter;
import com.gs.collections.impl.block.factory.primitive.IntToIntFunctions;
import com.gs.collections.impl.map.mutable.UnifiedMap;
import com.gs.collections.impl.map.mutable.primitive.ObjectIntHashMap;
import com.gs.collections.impl.multimap.bag.HashBagMultimap;
import com.gs.collections.impl.utility.ArrayIterate;
import com.gs.collections.impl.utility.Iterate;
/**
* A HashBag is a MutableBag which uses a Map as its underlying data store. Each key in the Map represents some item,
* and the value in the map represents the current number of occurrences of that item.
*
* @since 1.0
*/
public class HashBag
extends AbstractMutableBag
implements Externalizable
{
private static final long serialVersionUID = 1L;
private MutableObjectIntMap items;
private int size;
public HashBag()
{
this.items = ObjectIntHashMap.newMap();
}
public HashBag(int size)
{
this.items = new ObjectIntHashMap(size);
}
private HashBag(MutableObjectIntMap map)
{
this.items = map;
this.size = (int) map.sum();
}
public static HashBag newBag()
{
return new HashBag();
}
public static HashBag newBag(int size)
{
return new HashBag(size);
}
public static HashBag newBag(Bag extends E> source)
{
HashBag result = HashBag.newBag(source.sizeDistinct());
result.addAllBag(source);
return result;
}
public static HashBag newBag(Iterable extends E> source)
{
if (source instanceof Bag)
{
return HashBag.newBag((Bag) source);
}
return HashBag.newBagWith((E[]) Iterate.toArray(source));
}
public static HashBag newBagWith(E... elements)
{
HashBag result = HashBag.newBag();
ArrayIterate.addAllTo(elements, result);
return result;
}
@Override
public boolean addAll(Collection extends T> source)
{
if (source instanceof Bag)
{
return this.addAllBag((Bag) source);
}
return super.addAll(source);
}
private boolean addAllBag(Bag extends T> source)
{
source.forEachWithOccurrences(new ObjectIntProcedure()
{
public void value(T each, int occurrences)
{
HashBag.this.addOccurrences(each, occurrences);
}
});
return source.notEmpty();
}
public void addOccurrences(T item, int occurrences)
{
if (occurrences < 0)
{
throw new IllegalArgumentException("Cannot add a negative number of occurrences");
}
if (occurrences > 0)
{
this.items.updateValue(item, 0, IntToIntFunctions.add(occurrences));
this.size += occurrences;
}
}
@Override
public boolean equals(Object other)
{
if (this == other)
{
return true;
}
if (!(other instanceof Bag))
{
return false;
}
final Bag> bag = (Bag>) other;
if (this.sizeDistinct() != bag.sizeDistinct())
{
return false;
}
return this.items.keyValuesView().allSatisfy(new Predicate>()
{
public boolean accept(ObjectIntPair each)
{
return bag.occurrencesOf(each.getOne()) == each.getTwo();
}
});
}
@Override
public int hashCode()
{
final Counter counter = new Counter();
this.items.forEachKeyValue(new ObjectIntProcedure()
{
public void value(T item, int count)
{
counter.add((item == null ? 0 : item.hashCode()) ^ count);
}
});
return counter.getCount();
}
@Override
protected RichIterable getKeysView()
{
return this.items.keysView();
}
public int sizeDistinct()
{
return this.items.size();
}
public int occurrencesOf(Object item)
{
return this.items.get(item);
}
public void forEachWithOccurrences(ObjectIntProcedure super T> objectIntProcedure)
{
this.items.forEachKeyValue(objectIntProcedure);
}
public MutableBag selectByOccurrences(final IntPredicate predicate)
{
MutableObjectIntMap map = this.items.select(new ObjectIntPredicate()
{
public boolean accept(T each, int occurrences)
{
return predicate.accept(occurrences);
}
});
return new HashBag(map);
}
public MutableMap toMapOfItemToCount()
{
final MutableMap map = UnifiedMap.newMap(this.items.size());
this.forEachWithOccurrences(new ObjectIntProcedure()
{
public void value(T item, int count)
{
map.put(item, count);
}
});
return map;
}
public boolean remove(Object item)
{
int newValue = this.items.updateValue((T) item, 0, IntToIntFunctions.decrement());
if (newValue <= 0)
{
this.items.removeKey((T) item);
if (newValue == -1)
{
return false;
}
}
this.size--;
return true;
}
public void clear()
{
this.items.clear();
this.size = 0;
}
@Override
public boolean isEmpty()
{
return this.items.isEmpty();
}
public void writeExternal(ObjectOutput out) throws IOException
{
((ObjectIntHashMap) this.items).writeExternal(out);
}
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException
{
this.items = new ObjectIntHashMap();
((ObjectIntHashMap) this.items).readExternal(in);
this.size = (int) this.items.sum();
}
public void each(final Procedure super T> procedure)
{
this.items.forEachKeyValue(new ObjectIntProcedure()
{
public void value(T key, int count)
{
for (int i = 0; i < count; i++)
{
procedure.value(key);
}
}
});
}
@Override
public void forEachWithIndex(final ObjectIntProcedure super T> objectIntProcedure)
{
final Counter index = new Counter();
this.items.forEachKeyValue(new ObjectIntProcedure()
{
public void value(T key, int count)
{
for (int i = 0; i < count; i++)
{
objectIntProcedure.value(key, index.getCount());
index.increment();
}
}
});
}
@Override
public void forEachWith(final Procedure2 super T, ? super P> procedure, final P parameter)
{
this.items.forEachKeyValue(new ObjectIntProcedure()
{
public void value(T key, int count)
{
for (int i = 0; i < count; i++)
{
procedure.value(key, parameter);
}
}
});
}
public Iterator iterator()
{
return new InternalIterator();
}
public boolean removeOccurrences(Object item, int occurrences)
{
if (occurrences < 0)
{
throw new IllegalArgumentException("Cannot remove a negative number of occurrences");
}
if (occurrences == 0)
{
return false;
}
int newValue = this.items.updateValue((T) item, 0, IntToIntFunctions.subtract(occurrences));
if (newValue <= 0)
{
this.size -= occurrences + newValue;
this.items.remove(item);
return newValue + occurrences != 0;
}
this.size -= occurrences;
return true;
}
public boolean setOccurrences(T item, int occurrences)
{
if (occurrences < 0)
{
throw new IllegalArgumentException("Cannot set a negative number of occurrences");
}
int originalOccurrences = this.items.get(item);
if (originalOccurrences == occurrences)
{
return false;
}
if (occurrences == 0)
{
this.items.remove(item);
}
else
{
this.items.put(item, occurrences);
}
this.size -= originalOccurrences - occurrences;
return true;
}
public HashBag without(T element)
{
this.remove(element);
return this;
}
public MutableBag newEmpty()
{
return HashBag.newBag();
}
public HashBag with(T element)
{
this.add(element);
return this;
}
public HashBag withAll(Iterable extends T> iterable)
{
this.addAllIterable(iterable);
return this;
}
public HashBag withoutAll(Iterable extends T> iterable)
{
this.removeAllIterable(iterable);
return this;
}
public boolean removeIf(Predicate super T> predicate)
{
boolean changed = false;
for (Iterator iterator = this.items.keySet().iterator(); iterator.hasNext(); )
{
T key = iterator.next();
if (predicate.accept(key))
{
this.size -= this.items.get(key);
iterator.remove();
changed = true;
}
}
return changed;
}
public boolean removeIfWith(Predicate2 super T, ? super P> predicate, P parameter)
{
boolean changed = false;
for (Iterator iterator = this.items.keySet().iterator(); iterator.hasNext(); )
{
T key = iterator.next();
if (predicate.accept(key, parameter))
{
this.size -= this.items.get(key);
iterator.remove();
changed = true;
}
}
return changed;
}
public boolean removeAllIterable(Iterable> iterable)
{
int oldSize = this.size;
if (iterable instanceof Bag)
{
Bag> source = (Bag>) iterable;
source.forEachWithOccurrences(new ObjectIntProcedure