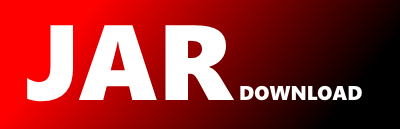
com.gs.collections.impl.bag.mutable.primitive.SynchronizedLongBag Maven / Gradle / Ivy
Show all versions of gs-collections Show documentation
/*
* Copyright 2014 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.bag.mutable.primitive;
import java.util.Collection;
import java.util.Collections;
import com.gs.collections.api.LongIterable;
import com.gs.collections.api.LazyLongIterable;
import com.gs.collections.api.bag.MutableBag;
import com.gs.collections.api.bag.primitive.ImmutableLongBag;
import com.gs.collections.api.bag.primitive.MutableLongBag;
import com.gs.collections.api.block.function.primitive.LongToObjectFunction;
import com.gs.collections.api.block.predicate.primitive.LongPredicate;
import com.gs.collections.api.block.procedure.primitive.LongIntProcedure;
import com.gs.collections.api.iterator.LongIterator;
import com.gs.collections.impl.collection.mutable.primitive.AbstractSynchronizedLongCollection;
import com.gs.collections.impl.factory.primitive.LongBags;
import com.gs.collections.impl.lazy.primitive.LazyLongIterableAdapter;
import net.jcip.annotations.GuardedBy;
import net.jcip.annotations.ThreadSafe;
/**
* A synchronized view of a {@link MutableLongBag}. It is imperative that the user manually synchronize on the collection when iterating over it using the
* {@link LongIterator}, as per {@link Collections#synchronizedCollection(Collection)}.
*
* This file was automatically generated from template file synchronizedPrimitiveBag.stg.
*
* @see MutableLongBag#asSynchronized()
* @see MutableBag#asSynchronized()
* @since 3.1.
*/
@ThreadSafe
public final class SynchronizedLongBag
extends AbstractSynchronizedLongCollection
implements MutableLongBag
{
private static final long serialVersionUID = 1L;
SynchronizedLongBag(MutableLongBag bag)
{
super(bag);
}
SynchronizedLongBag(MutableLongBag bag, Object newLock)
{
super(bag, newLock);
}
@GuardedBy("getLock()")
private MutableLongBag getMutableLongBag()
{
return (MutableLongBag) this.getLongCollection();
}
@Override
public SynchronizedLongBag with(long element)
{
synchronized (this.getLock())
{
this.getMutableLongBag().add(element);
}
return this;
}
@Override
public SynchronizedLongBag without(long element)
{
synchronized (this.getLock())
{
this.getMutableLongBag().remove(element);
}
return this;
}
@Override
public SynchronizedLongBag withAll(LongIterable elements)
{
synchronized (this.getLock())
{
this.getMutableLongBag().addAll(elements.toArray());
}
return this;
}
@Override
public SynchronizedLongBag withoutAll(LongIterable elements)
{
synchronized (this.getLock())
{
this.getMutableLongBag().removeAll(elements);
}
return this;
}
public void addOccurrences(long item, int occurrences)
{
synchronized (this.getLock())
{
this.getMutableLongBag().addOccurrences(item, occurrences);
}
}
public boolean removeOccurrences(long item, int occurrences)
{
synchronized (this.getLock())
{
return this.getMutableLongBag().removeOccurrences(item, occurrences);
}
}
public int sizeDistinct()
{
synchronized (this.getLock())
{
return this.getMutableLongBag().sizeDistinct();
}
}
public int occurrencesOf(long item)
{
synchronized (this.getLock())
{
return this.getMutableLongBag().occurrencesOf(item);
}
}
public void forEachWithOccurrences(LongIntProcedure procedure)
{
synchronized (this.getLock())
{
this.getMutableLongBag().forEachWithOccurrences(procedure);
}
}
@Override
public MutableLongBag select(LongPredicate predicate)
{
synchronized (this.getLock())
{
return this.getMutableLongBag().select(predicate);
}
}
@Override
public MutableLongBag reject(LongPredicate predicate)
{
synchronized (this.getLock())
{
return this.getMutableLongBag().reject(predicate);
}
}
@Override
public MutableBag collect(LongToObjectFunction extends V> function)
{
synchronized (this.getLock())
{
return this.getMutableLongBag().collect(function);
}
}
@Override
public boolean equals(Object otherBag)
{
synchronized (this.getLock())
{
return this.getMutableLongBag().equals(otherBag);
}
}
@Override
public int hashCode()
{
synchronized (this.getLock())
{
return this.getMutableLongBag().hashCode();
}
}
@Override
public LazyLongIterable asLazy()
{
synchronized (this.getLock())
{
return new LazyLongIterableAdapter(this);
}
}
@Override
public MutableLongBag asUnmodifiable()
{
return new UnmodifiableLongBag(this);
}
@Override
public MutableLongBag asSynchronized()
{
return this;
}
@Override
public ImmutableLongBag toImmutable()
{
return LongBags.immutable.withAll(this);
}
}