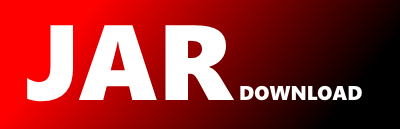
com.gs.collections.impl.bag.mutable.primitive.UnmodifiableBooleanBag Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gs-collections Show documentation
Show all versions of gs-collections Show documentation
GS Collections is a collections framework for Java. It has JDK-compatible List, Set and Map
implementations with a rich API and set of utility classes that work with any JDK compatible Collections,
Arrays, Maps or Strings. The iteration protocol was inspired by the Smalltalk collection framework.
/*
* Copyright 2014 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.bag.mutable.primitive;
import com.gs.collections.api.BooleanIterable;
import com.gs.collections.api.LazyBooleanIterable;
import com.gs.collections.api.bag.MutableBag;
import com.gs.collections.api.bag.primitive.ImmutableBooleanBag;
import com.gs.collections.api.bag.primitive.MutableBooleanBag;
import com.gs.collections.api.block.function.primitive.BooleanToObjectFunction;
import com.gs.collections.api.block.predicate.primitive.BooleanPredicate;
import com.gs.collections.api.block.procedure.primitive.BooleanIntProcedure;
import com.gs.collections.impl.collection.mutable.primitive.AbstractUnmodifiableBooleanCollection;
import com.gs.collections.impl.factory.primitive.BooleanBags;
import com.gs.collections.impl.lazy.primitive.LazyBooleanIterableAdapter;
import net.jcip.annotations.NotThreadSafe;
/**
* This file was automatically generated from template file unmodifiablePrimitiveBag.stg.
*
* @since 3.1.
*/
@NotThreadSafe
public final class UnmodifiableBooleanBag
extends AbstractUnmodifiableBooleanCollection
implements MutableBooleanBag
{
private static final long serialVersionUID = 1L;
UnmodifiableBooleanBag(MutableBooleanBag bag)
{
super(bag);
}
private MutableBooleanBag getMutableBooleanBag()
{
return (MutableBooleanBag) this.getBooleanCollection();
}
@Override
public UnmodifiableBooleanBag with(boolean element)
{
throw new UnsupportedOperationException("Cannot call with() on " + this.getClass().getSimpleName());
}
@Override
public UnmodifiableBooleanBag without(boolean element)
{
throw new UnsupportedOperationException("Cannot call without() on " + this.getClass().getSimpleName());
}
@Override
public UnmodifiableBooleanBag withAll(BooleanIterable elements)
{
throw new UnsupportedOperationException("Cannot call withAll() on " + this.getClass().getSimpleName());
}
@Override
public UnmodifiableBooleanBag withoutAll(BooleanIterable elements)
{
throw new UnsupportedOperationException("Cannot call withoutAll() on " + this.getClass().getSimpleName());
}
public void addOccurrences(boolean item, int occurrences)
{
throw new UnsupportedOperationException("Cannot call addOccurrences() on " + this.getClass().getSimpleName());
}
public boolean removeOccurrences(boolean item, int occurrences)
{
throw new UnsupportedOperationException("Cannot call removeOccurrences() on " + this.getClass().getSimpleName());
}
public int sizeDistinct()
{
return this.getMutableBooleanBag().sizeDistinct();
}
public int occurrencesOf(boolean item)
{
return this.getMutableBooleanBag().occurrencesOf(item);
}
public void forEachWithOccurrences(BooleanIntProcedure procedure)
{
this.getMutableBooleanBag().forEachWithOccurrences(procedure);
}
@Override
public MutableBooleanBag select(BooleanPredicate predicate)
{
return this.getMutableBooleanBag().select(predicate);
}
@Override
public MutableBooleanBag reject(BooleanPredicate predicate)
{
return this.getMutableBooleanBag().reject(predicate);
}
@Override
public MutableBag collect(BooleanToObjectFunction extends V> function)
{
return this.getMutableBooleanBag().collect(function);
}
@Override
public boolean equals(Object otherBag)
{
return this.getMutableBooleanBag().equals(otherBag);
}
@Override
public int hashCode()
{
return this.getMutableBooleanBag().hashCode();
}
@Override
public MutableBooleanBag asUnmodifiable()
{
return this;
}
@Override
public MutableBooleanBag asSynchronized()
{
return new SynchronizedBooleanBag(this);
}
@Override
public ImmutableBooleanBag toImmutable()
{
return BooleanBags.immutable.withAll(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy