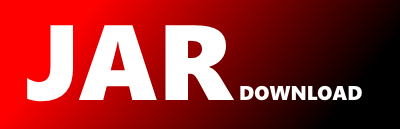
com.gs.collections.impl.lazy.parallel.AbstractParallelIterableImpl Maven / Gradle / Ivy
Show all versions of gs-collections Show documentation
/*
* Copyright 2015 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.lazy.parallel;
import com.gs.collections.api.ParallelIterable;
import com.gs.collections.api.annotation.Beta;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.function.Function2;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.api.multimap.bag.MutableBagMultimap;
import com.gs.collections.api.multimap.bag.UnsortedBagMultimap;
import com.gs.collections.api.set.ParallelUnsortedSetIterable;
import com.gs.collections.impl.block.factory.Functions;
import com.gs.collections.impl.block.factory.Predicates;
import com.gs.collections.impl.lazy.parallel.set.ParallelCollectIterable;
import com.gs.collections.impl.lazy.parallel.set.ParallelFlatCollectIterable;
import com.gs.collections.impl.multimap.bag.SynchronizedPutHashBagMultimap;
@Beta
public abstract class AbstractParallelIterableImpl> extends AbstractParallelIterable
{
@Override
protected boolean isOrdered()
{
return false;
}
public ParallelUnsortedSetIterable asUnique()
{
return new ParallelDistinctIterable(this);
}
public ParallelIterable select(Predicate super T> predicate)
{
return new ParallelSelectIterable(this, predicate);
}
public ParallelIterable selectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return this.select(Predicates.bind(predicate, parameter));
}
public ParallelIterable selectInstancesOf(Class clazz)
{
return (ParallelIterable) this.select(Predicates.instanceOf(clazz));
}
public ParallelIterable reject(Predicate super T> predicate)
{
return this.select(Predicates.not(predicate));
}
public ParallelIterable rejectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return this.reject(Predicates.bind(predicate, parameter));
}
public ParallelIterable collect(Function super T, ? extends V> function)
{
return new ParallelCollectIterable(this, function);
}
public ParallelIterable collectWith(Function2 super T, ? super P, ? extends V> function, P parameter)
{
return this.collect(Functions.bind(function, parameter));
}
public ParallelIterable collectIf(Predicate super T> predicate, Function super T, ? extends V> function)
{
return this.select(predicate).collect(function);
}
public ParallelIterable flatCollect(Function super T, ? extends Iterable> function)
{
return new ParallelFlatCollectIterable(this, function);
}
public UnsortedBagMultimap groupBy(final Function super T, ? extends V> function)
{
final MutableBagMultimap result = SynchronizedPutHashBagMultimap.newMultimap();
this.forEach(new Procedure()
{
public void value(T each)
{
V key = function.valueOf(each);
result.put(key, each);
}
});
return result;
}
public UnsortedBagMultimap groupByEach(final Function super T, ? extends Iterable> function)
{
final MutableBagMultimap result = SynchronizedPutHashBagMultimap.newMultimap();
this.forEach(new Procedure()
{
public void value(T each)
{
Iterable keys = function.valueOf(each);
for (V key : keys)
{
result.put(key, each);
}
}
});
return result;
}
}