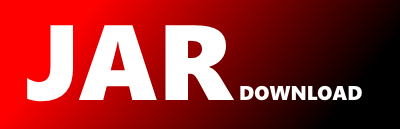
com.gs.collections.impl.lazy.parallel.ParallelSelectIterable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gs-collections Show documentation
Show all versions of gs-collections Show documentation
GS Collections is a collections framework for Java. It has JDK-compatible List, Set and Map
implementations with a rich API and set of utility classes that work with any JDK compatible Collections,
Arrays, Maps or Strings. The iteration protocol was inspired by the Smalltalk collection framework.
/*
* Copyright 2015 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.lazy.parallel;
import java.util.concurrent.ExecutorService;
import com.gs.collections.api.LazyIterable;
import com.gs.collections.api.annotation.Beta;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.impl.block.factory.Predicates;
import com.gs.collections.impl.block.procedure.IfProcedure;
@Beta
public class ParallelSelectIterable extends AbstractParallelIterableImpl>
{
private final AbstractParallelIterable> parallelIterable;
private final Predicate super T> predicate;
public ParallelSelectIterable(AbstractParallelIterable> parallelIterable, Predicate super T> predicate)
{
this.parallelIterable = parallelIterable;
this.predicate = predicate;
}
@Override
public ExecutorService getExecutorService()
{
return this.parallelIterable.getExecutorService();
}
@Override
public int getBatchSize()
{
return this.parallelIterable.getBatchSize();
}
@Override
public LazyIterable> split()
{
return this.parallelIterable.split().collect(new Function, Batch>()
{
public Batch valueOf(Batch eachBatch)
{
return eachBatch.select(ParallelSelectIterable.this.predicate);
}
});
}
public void forEach(Procedure super T> procedure)
{
this.parallelIterable.forEach(new IfProcedure(this.predicate, procedure));
}
public boolean anySatisfy(Predicate super T> predicate)
{
return this.parallelIterable.anySatisfy(Predicates.and(this.predicate, predicate));
}
public boolean allSatisfy(Predicate super T> predicate)
{
return this.parallelIterable.allSatisfy(new SelectAllSatisfyPredicate(this.predicate, predicate));
}
public T detect(Predicate super T> predicate)
{
return this.parallelIterable.detect(Predicates.and(this.predicate, predicate));
}
@Override
public Object[] toArray()
{
// TODO: Implement in parallel
return this.parallelIterable.toList().select(this.predicate).toArray();
}
@Override
public E[] toArray(E[] array)
{
// TODO: Implement in parallel
return this.parallelIterable.toList().select(this.predicate).toArray(array);
}
private static final class SelectAllSatisfyPredicate implements Predicate
{
private final Predicate super T> left;
private final Predicate super T> right;
private SelectAllSatisfyPredicate(Predicate super T> left, Predicate super T> right)
{
this.left = left;
this.right = right;
}
public boolean accept(T each)
{
boolean leftResult = this.left.accept(each);
return !leftResult || this.right.accept(each);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy