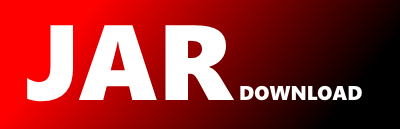
com.gs.collections.impl.lazy.parallel.SynchronizedParallelIterable Maven / Gradle / Ivy
Show all versions of gs-collections Show documentation
/*
* Copyright 2014 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.lazy.parallel;
import com.gs.collections.api.ParallelIterable;
import com.gs.collections.api.annotation.Beta;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.function.Function2;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.multimap.Multimap;
@Beta
public final class SynchronizedParallelIterable extends AbstractSynchronizedParallelIterable>
{
public SynchronizedParallelIterable(ParallelIterable delegate, Object lock)
{
super(delegate, lock);
}
public ParallelIterable asUnique()
{
return this.wrap(this.delegate.asUnique());
}
public ParallelIterable select(Predicate super T> predicate)
{
return this.wrap(this.delegate.select(predicate));
}
public ParallelIterable selectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return this.wrap(this.delegate.selectWith(predicate, parameter));
}
public ParallelIterable reject(Predicate super T> predicate)
{
return this.wrap(this.delegate.reject(predicate));
}
public ParallelIterable rejectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return this.wrap(this.delegate.rejectWith(predicate, parameter));
}
public ParallelIterable selectInstancesOf(Class clazz)
{
return this.wrap(this.delegate.selectInstancesOf(clazz));
}
public ParallelIterable collect(Function super T, ? extends V> function)
{
return this.wrap(this.delegate.collect(function));
}
public ParallelIterable collectWith(Function2 super T, ? super P, ? extends V> function, P parameter)
{
return this.wrap(this.delegate.collectWith(function, parameter));
}
public ParallelIterable collectIf(Predicate super T> predicate, Function super T, ? extends V> function)
{
return this.wrap(this.delegate.collectIf(predicate, function));
}
public ParallelIterable flatCollect(Function super T, ? extends Iterable> function)
{
return this.wrap(this.delegate.flatCollect(function));
}
public Multimap groupBy(Function super T, ? extends V> function)
{
synchronized (this.lock)
{
return this.delegate.groupBy(function);
}
}
public Multimap groupByEach(Function super T, ? extends Iterable> function)
{
synchronized (this.lock)
{
return this.delegate.groupByEach(function);
}
}
}