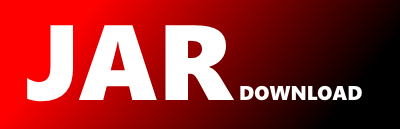
com.gs.collections.impl.list.mutable.UnmodifiableMutableList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gs-collections Show documentation
Show all versions of gs-collections Show documentation
GS Collections is a collections framework for Java. It has JDK-compatible List, Set and Map
implementations with a rich API and set of utility classes that work with any JDK compatible Collections,
Arrays, Maps or Strings. The iteration protocol was inspired by the Smalltalk collection framework.
/*
* Copyright 2015 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.list.mutable;
import java.io.Serializable;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import java.util.ListIterator;
import java.util.Random;
import java.util.RandomAccess;
import java.util.concurrent.ExecutorService;
import com.gs.collections.api.LazyIterable;
import com.gs.collections.api.block.HashingStrategy;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.function.Function2;
import com.gs.collections.api.block.function.primitive.BooleanFunction;
import com.gs.collections.api.block.function.primitive.ByteFunction;
import com.gs.collections.api.block.function.primitive.CharFunction;
import com.gs.collections.api.block.function.primitive.DoubleFunction;
import com.gs.collections.api.block.function.primitive.FloatFunction;
import com.gs.collections.api.block.function.primitive.IntFunction;
import com.gs.collections.api.block.function.primitive.LongFunction;
import com.gs.collections.api.block.function.primitive.ShortFunction;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.api.block.procedure.primitive.ObjectIntProcedure;
import com.gs.collections.api.list.ImmutableList;
import com.gs.collections.api.list.MutableList;
import com.gs.collections.api.list.ParallelListIterable;
import com.gs.collections.api.list.primitive.MutableBooleanList;
import com.gs.collections.api.list.primitive.MutableByteList;
import com.gs.collections.api.list.primitive.MutableCharList;
import com.gs.collections.api.list.primitive.MutableDoubleList;
import com.gs.collections.api.list.primitive.MutableFloatList;
import com.gs.collections.api.list.primitive.MutableIntList;
import com.gs.collections.api.list.primitive.MutableLongList;
import com.gs.collections.api.list.primitive.MutableShortList;
import com.gs.collections.api.multimap.list.MutableListMultimap;
import com.gs.collections.api.ordered.OrderedIterable;
import com.gs.collections.api.partition.list.PartitionMutableList;
import com.gs.collections.api.stack.MutableStack;
import com.gs.collections.api.tuple.Pair;
import com.gs.collections.impl.block.factory.HashingStrategies;
import com.gs.collections.impl.collection.mutable.AbstractUnmodifiableMutableCollection;
import com.gs.collections.impl.collection.mutable.UnmodifiableCollectionSerializationProxy;
import com.gs.collections.impl.lazy.ReverseIterable;
import com.gs.collections.impl.stack.mutable.ArrayStack;
/**
* An unmodifiable view of a list.
*
* @see MutableList#asUnmodifiable()
*/
public class UnmodifiableMutableList
extends AbstractUnmodifiableMutableCollection
implements MutableList, Serializable
{
UnmodifiableMutableList(MutableList extends T> mutableList)
{
super(mutableList);
}
/**
* This method will take a MutableList and wrap it directly in a UnmodifiableMutableList. It will
* take any other non-GS-list and first adapt it will a ListAdapter, and then return a
* UnmodifiableMutableList that wraps the adapter.
*/
public static > UnmodifiableMutableList of(L list)
{
if (list == null)
{
throw new IllegalArgumentException("cannot create an UnmodifiableMutableList for null");
}
if (list instanceof RandomAccess)
{
return new RandomAccessUnmodifiableMutableList(RandomAccessListAdapter.adapt(list));
}
return new UnmodifiableMutableList(ListAdapter.adapt(list));
}
private MutableList getMutableList()
{
return (MutableList) this.getMutableCollection();
}
@Override
public boolean equals(Object obj)
{
return this.getMutableList().equals(obj);
}
@Override
public int hashCode()
{
return this.getMutableList().hashCode();
}
@Override
public MutableList asUnmodifiable()
{
return this;
}
@Override
public ImmutableList toImmutable()
{
return this.getMutableList().toImmutable();
}
@Override
public MutableList asSynchronized()
{
return SynchronizedMutableList.of(this);
}
@Override
public UnmodifiableMutableList clone()
{
return this;
}
@Override
public MutableList newEmpty()
{
return this.getMutableList().newEmpty();
}
@Override
public MutableList tap(Procedure super T> procedure)
{
this.forEach(procedure);
return this;
}
public boolean corresponds(OrderedIterable other, Predicate2 super T, ? super S> predicate)
{
return this.getMutableList().corresponds(other, predicate);
}
public void forEach(int fromIndex, int toIndex, Procedure super T> procedure)
{
this.getMutableList().forEach(fromIndex, toIndex, procedure);
}
public void reverseForEach(Procedure super T> procedure)
{
this.getMutableList().reverseForEach(procedure);
}
public void forEachWithIndex(int fromIndex, int toIndex, ObjectIntProcedure super T> objectIntProcedure)
{
this.getMutableList().forEachWithIndex(fromIndex, toIndex, objectIntProcedure);
}
public UnmodifiableMutableList sortThis(Comparator super T> comparator)
{
throw new UnsupportedOperationException("Cannot call sortThis() on " + this.getClass().getSimpleName());
}
public UnmodifiableMutableList sortThis()
{
throw new UnsupportedOperationException("Cannot call sortThis() on " + this.getClass().getSimpleName());
}
public MutableList toReversed()
{
return this.getMutableList().toReversed();
}
public MutableList reverseThis()
{
throw new UnsupportedOperationException("Cannot call reverseThis() on " + this.getClass().getSimpleName());
}
public MutableList shuffleThis()
{
throw new UnsupportedOperationException("Cannot call shuffleThis() on " + this.getClass().getSimpleName());
}
public MutableList shuffleThis(Random rnd)
{
throw new UnsupportedOperationException("Cannot call shuffleThis() on " + this.getClass().getSimpleName());
}
public MutableStack toStack()
{
return ArrayStack.newStack(this.getMutableList());
}
public > MutableList sortThisBy(Function super T, ? extends V> function)
{
throw new UnsupportedOperationException("Cannot call sortThisBy() on " + this.getClass().getSimpleName());
}
public MutableList sortThisByInt(IntFunction super T> function)
{
throw new UnsupportedOperationException("Cannot call sortThisByInt() on " + this.getClass().getSimpleName());
}
public MutableList sortThisByBoolean(BooleanFunction super T> function)
{
throw new UnsupportedOperationException("Cannot call sortThisByBoolean() on " + this.getClass().getSimpleName());
}
public MutableList sortThisByChar(CharFunction super T> function)
{
throw new UnsupportedOperationException("Cannot call sortThisByChar() on " + this.getClass().getSimpleName());
}
public MutableList sortThisByByte(ByteFunction super T> function)
{
throw new UnsupportedOperationException("Cannot call sortThisByByte() on " + this.getClass().getSimpleName());
}
public MutableList sortThisByShort(ShortFunction super T> function)
{
throw new UnsupportedOperationException("Cannot call sortThisByShort() on " + this.getClass().getSimpleName());
}
public MutableList sortThisByFloat(FloatFunction super T> function)
{
throw new UnsupportedOperationException("Cannot call sortThisByFloat() on " + this.getClass().getSimpleName());
}
public MutableList sortThisByLong(LongFunction super T> function)
{
throw new UnsupportedOperationException("Cannot call sortThisByLong() on " + this.getClass().getSimpleName());
}
public MutableList sortThisByDouble(DoubleFunction super T> function)
{
throw new UnsupportedOperationException("Cannot call sortThisByDouble() on " + this.getClass().getSimpleName());
}
public boolean addAll(int index, Collection extends T> collection)
{
throw new UnsupportedOperationException("Cannot call addAll() on " + this.getClass().getSimpleName());
}
public T get(int index)
{
return this.getMutableList().get(index);
}
public T set(int index, T element)
{
throw new UnsupportedOperationException("Cannot call set() on " + this.getClass().getSimpleName());
}
public void add(int index, T element)
{
throw new UnsupportedOperationException("Cannot call add() on " + this.getClass().getSimpleName());
}
public T remove(int index)
{
throw new UnsupportedOperationException("Cannot call remove() on " + this.getClass().getSimpleName());
}
public int indexOf(Object o)
{
return this.getMutableList().indexOf(o);
}
public int lastIndexOf(Object o)
{
return this.getMutableList().lastIndexOf(o);
}
public ListIterator listIterator()
{
return this.listIterator(0);
}
public ListIterator listIterator(int index)
{
return new UnmodifiableListIteratorAdapter(this.getMutableList().listIterator(index));
}
public UnmodifiableMutableList subList(int fromIndex, int toIndex)
{
MutableList subList = this.getMutableList().subList(fromIndex, toIndex);
return UnmodifiableMutableList.of(subList);
}
@Override
public
© 2015 - 2025 Weber Informatics LLC | Privacy Policy