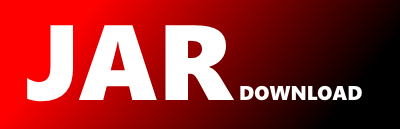
com.gs.collections.impl.map.immutable.ImmutableMapFactoryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gs-collections Show documentation
Show all versions of gs-collections Show documentation
GS Collections is a collections framework for Java. It has JDK-compatible List, Set and Map
implementations with a rich API and set of utility classes that work with any JDK compatible Collections,
Arrays, Maps or Strings. The iteration protocol was inspired by the Smalltalk collection framework.
/*
* Copyright 2014 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.map.immutable;
import java.util.Map;
import com.gs.collections.api.factory.map.ImmutableMapFactory;
import com.gs.collections.api.map.ImmutableMap;
import com.gs.collections.impl.block.factory.Comparators;
import net.jcip.annotations.Immutable;
@Immutable
public final class ImmutableMapFactoryImpl implements ImmutableMapFactory
{
public ImmutableMap empty()
{
return (ImmutableMap) ImmutableEmptyMap.INSTANCE;
}
public ImmutableMap of()
{
return this.empty();
}
public ImmutableMap with()
{
return this.empty();
}
public ImmutableMap of(K key, V value)
{
return this.with(key, value);
}
public ImmutableMap with(K key, V value)
{
return new ImmutableSingletonMap(key, value);
}
public ImmutableMap of(K key1, V value1, K key2, V value2)
{
return this.with(key1, value1, key2, value2);
}
public ImmutableMap with(K key1, V value1, K key2, V value2)
{
if (Comparators.nullSafeEquals(key1, key2))
{
return this.of(key1, value2);
}
return new ImmutableDoubletonMap(key1, value1, key2, value2);
}
public ImmutableMap of(K key1, V value1, K key2, V value2, K key3, V value3)
{
return this.with(key1, value1, key2, value2, key3, value3);
}
public ImmutableMap with(K key1, V value1, K key2, V value2, K key3, V value3)
{
if (Comparators.nullSafeEquals(key1, key2) && Comparators.nullSafeEquals(key2, key3))
{
return this.of(key1, value3);
}
if (Comparators.nullSafeEquals(key1, key2))
{
return this.of(key1, value2, key3, value3);
}
if (Comparators.nullSafeEquals(key1, key3))
{
return this.of(key2, value2, key1, value3);
}
if (Comparators.nullSafeEquals(key2, key3))
{
return this.of(key1, value1, key2, value3);
}
return new ImmutableTripletonMap(key1, value1, key2, value2, key3, value3);
}
public ImmutableMap of(K key1, V value1, K key2, V value2, K key3, V value3, K key4, V value4)
{
return this.with(key1, value1, key2, value2, key3, value3, key4, value4);
}
public ImmutableMap with(K key1, V value1, K key2, V value2, K key3, V value3, K key4, V value4)
{
if (Comparators.nullSafeEquals(key1, key2))
{
return this.of(key1, value2, key3, value3, key4, value4);
}
if (Comparators.nullSafeEquals(key1, key3))
{
return this.of(key2, value2, key1, value3, key4, value4);
}
if (Comparators.nullSafeEquals(key1, key4))
{
return this.of(key2, value2, key3, value3, key1, value4);
}
if (Comparators.nullSafeEquals(key2, key3))
{
return this.of(key1, value1, key2, value3, key4, value4);
}
if (Comparators.nullSafeEquals(key2, key4))
{
return this.of(key1, value1, key3, value3, key2, value4);
}
if (Comparators.nullSafeEquals(key3, key4))
{
return this.of(key1, value1, key2, value2, key3, value4);
}
return new ImmutableQuadrupletonMap(key1, value1, key2, value2, key3, value3, key4, value4);
}
/**
* @deprecated use {@link #ofAll(Map)} instead (inlineable)
*/
@Deprecated
public ImmutableMap ofMap(Map map)
{
return this.ofAll(map);
}
public ImmutableMap ofAll(Map map)
{
return this.withAll(map);
}
public ImmutableMap withAll(Map map)
{
if (map.isEmpty())
{
return this.of();
}
if (map.size() > 4)
{
return new ImmutableUnifiedMap(map);
}
Map.Entry[] entries = map.entrySet().toArray(new Map.Entry[map.entrySet().size()]);
switch (entries.length)
{
case 1:
return this.of(entries[0].getKey(), entries[0].getValue());
case 2:
return this.of(
entries[0].getKey(), entries[0].getValue(),
entries[1].getKey(), entries[1].getValue());
case 3:
return this.of(
entries[0].getKey(), entries[0].getValue(),
entries[1].getKey(), entries[1].getValue(),
entries[2].getKey(), entries[2].getValue());
case 4:
return this.of(
entries[0].getKey(), entries[0].getValue(),
entries[1].getKey(), entries[1].getValue(),
entries[2].getKey(), entries[2].getValue(),
entries[3].getKey(), entries[3].getValue());
default:
throw new AssertionError();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy