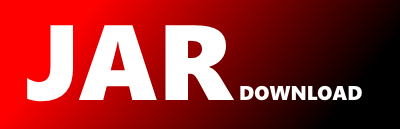
com.gs.collections.impl.map.mutable.AbstractMutableMap Maven / Gradle / Ivy
Show all versions of gs-collections Show documentation
/*
* Copyright 2015 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.map.mutable;
import java.util.Collection;
import com.gs.collections.api.bag.MutableBag;
import com.gs.collections.api.bag.primitive.MutableBooleanBag;
import com.gs.collections.api.bag.primitive.MutableByteBag;
import com.gs.collections.api.bag.primitive.MutableCharBag;
import com.gs.collections.api.bag.primitive.MutableDoubleBag;
import com.gs.collections.api.bag.primitive.MutableFloatBag;
import com.gs.collections.api.bag.primitive.MutableIntBag;
import com.gs.collections.api.bag.primitive.MutableLongBag;
import com.gs.collections.api.bag.primitive.MutableShortBag;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.function.Function2;
import com.gs.collections.api.block.function.primitive.BooleanFunction;
import com.gs.collections.api.block.function.primitive.ByteFunction;
import com.gs.collections.api.block.function.primitive.CharFunction;
import com.gs.collections.api.block.function.primitive.DoubleFunction;
import com.gs.collections.api.block.function.primitive.FloatFunction;
import com.gs.collections.api.block.function.primitive.IntFunction;
import com.gs.collections.api.block.function.primitive.LongFunction;
import com.gs.collections.api.block.function.primitive.ShortFunction;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.api.map.ImmutableMap;
import com.gs.collections.api.map.MutableMap;
import com.gs.collections.api.multimap.bag.MutableBagMultimap;
import com.gs.collections.api.multimap.set.MutableSetMultimap;
import com.gs.collections.api.ordered.OrderedIterable;
import com.gs.collections.api.partition.bag.PartitionMutableBag;
import com.gs.collections.api.set.MutableSet;
import com.gs.collections.api.tuple.Pair;
import com.gs.collections.impl.bag.mutable.HashBag;
import com.gs.collections.impl.bag.mutable.primitive.BooleanHashBag;
import com.gs.collections.impl.bag.mutable.primitive.ByteHashBag;
import com.gs.collections.impl.bag.mutable.primitive.CharHashBag;
import com.gs.collections.impl.bag.mutable.primitive.DoubleHashBag;
import com.gs.collections.impl.bag.mutable.primitive.FloatHashBag;
import com.gs.collections.impl.bag.mutable.primitive.IntHashBag;
import com.gs.collections.impl.bag.mutable.primitive.LongHashBag;
import com.gs.collections.impl.bag.mutable.primitive.ShortHashBag;
import com.gs.collections.impl.block.factory.Functions;
import com.gs.collections.impl.block.factory.Predicates;
import com.gs.collections.impl.block.procedure.PartitionPredicate2Procedure;
import com.gs.collections.impl.block.procedure.PartitionProcedure;
import com.gs.collections.impl.block.procedure.SelectInstancesOfProcedure;
import com.gs.collections.impl.block.procedure.primitive.CollectBooleanProcedure;
import com.gs.collections.impl.block.procedure.primitive.CollectByteProcedure;
import com.gs.collections.impl.block.procedure.primitive.CollectCharProcedure;
import com.gs.collections.impl.block.procedure.primitive.CollectDoubleProcedure;
import com.gs.collections.impl.block.procedure.primitive.CollectFloatProcedure;
import com.gs.collections.impl.block.procedure.primitive.CollectIntProcedure;
import com.gs.collections.impl.block.procedure.primitive.CollectLongProcedure;
import com.gs.collections.impl.block.procedure.primitive.CollectShortProcedure;
import com.gs.collections.impl.factory.Maps;
import com.gs.collections.impl.list.fixed.ArrayAdapter;
import com.gs.collections.impl.multimap.bag.HashBagMultimap;
import com.gs.collections.impl.partition.bag.PartitionHashBag;
import com.gs.collections.impl.set.mutable.UnifiedSet;
import com.gs.collections.impl.utility.MapIterate;
public abstract class AbstractMutableMap extends AbstractMutableMapIterable
implements MutableMap
{
@SuppressWarnings("AbstractMethodOverridesAbstractMethod")
public abstract MutableMap clone();
/**
* Creates a new instance of the same type, using the given capacity and the default growth parameters.
*/
public abstract MutableMap newEmpty(int capacity);
public MutableMap asUnmodifiable()
{
return UnmodifiableMutableMap.of(this);
}
public ImmutableMap toImmutable()
{
return Maps.immutable.withAll(this);
}
public MutableMap asSynchronized()
{
return SynchronizedMutableMap.of(this);
}
public MutableSetMultimap flip()
{
return MapIterate.flip(this);
}
public MutableMap collectValues(Function2 super K, ? super V, ? extends R> function)
{
return MapIterate.collectValues(this, function, this.newEmpty(this.size()));
}
public MutableMap select(Predicate2 super K, ? super V> predicate)
{
return MapIterate.selectMapOnEntry(this, predicate, this.newEmpty());
}
public MutableMap reject(Predicate2 super K, ? super V> predicate)
{
return MapIterate.rejectMapOnEntry(this, predicate, this.newEmpty());
}
public MutableBag collect(Function super V, ? extends R> function)
{
return this.collect(function, HashBag.newBag());
}
public MutableBooleanBag collectBoolean(BooleanFunction super V> booleanFunction)
{
MutableBooleanBag result = new BooleanHashBag();
this.forEach(new CollectBooleanProcedure(booleanFunction, result));
return result;
}
public MutableByteBag collectByte(ByteFunction super V> byteFunction)
{
MutableByteBag result = new ByteHashBag();
this.forEach(new CollectByteProcedure(byteFunction, result));
return result;
}
public MutableCharBag collectChar(CharFunction super V> charFunction)
{
MutableCharBag result = new CharHashBag();
this.forEach(new CollectCharProcedure(charFunction, result));
return result;
}
public MutableDoubleBag collectDouble(DoubleFunction super V> doubleFunction)
{
MutableDoubleBag result = new DoubleHashBag();
this.forEach(new CollectDoubleProcedure(doubleFunction, result));
return result;
}
public MutableFloatBag collectFloat(FloatFunction super V> floatFunction)
{
MutableFloatBag result = new FloatHashBag();
this.forEach(new CollectFloatProcedure(floatFunction, result));
return result;
}
public MutableIntBag collectInt(IntFunction super V> intFunction)
{
MutableIntBag result = new IntHashBag();
this.forEach(new CollectIntProcedure(intFunction, result));
return result;
}
public MutableLongBag collectLong(LongFunction super V> longFunction)
{
MutableLongBag result = new LongHashBag();
this.forEach(new CollectLongProcedure(longFunction, result));
return result;
}
public MutableShortBag collectShort(ShortFunction super V> shortFunction)
{
MutableShortBag result = new ShortHashBag();
this.forEach(new CollectShortProcedure(shortFunction, result));
return result;
}
public MutableBag collectWith(Function2 super V, ? super P, ? extends VV> function, P parameter)
{
return this.collect(Functions.bind(function, parameter));
}
public MutableBag collectIf(Predicate super V> predicate, Function super V, ? extends R> function)
{
return this.collectIf(predicate, function, new HashBag());
}
public MutableBag flatCollect(Function super V, ? extends Iterable> function)
{
return this.flatCollect(function, new HashBag());
}
public MutableBag select(Predicate super V> predicate)
{
return this.select(predicate, new HashBag());
}
public MutableMap tap(Procedure super V> procedure)
{
this.forEach(procedure);
return this;
}
public MutableBag selectWith(Predicate2 super V, ? super P> predicate, P parameter)
{
return this.select(Predicates.bind(predicate, parameter));
}
public MutableBag reject(Predicate super V> predicate)
{
return this.reject(predicate, new HashBag());
}
public MutableBag rejectWith(Predicate2 super V, ? super P> predicate, P parameter)
{
return this.reject(Predicates.bind(predicate, parameter));
}
public PartitionMutableBag partition(Predicate super V> predicate)
{
PartitionMutableBag partitionMutableBag = new PartitionHashBag();
this.forEach(new PartitionProcedure(predicate, partitionMutableBag));
return partitionMutableBag;
}
public PartitionMutableBag partitionWith(Predicate2 super V, ? super P> predicate, P parameter)
{
PartitionMutableBag partitionMutableBag = new PartitionHashBag();
this.forEach(new PartitionPredicate2Procedure(predicate, parameter, partitionMutableBag));
return partitionMutableBag;
}
public MutableBag selectInstancesOf(Class clazz)
{
MutableBag result = HashBag.newBag();
this.forEach(new SelectInstancesOfProcedure(clazz, result));
return result;
}
/**
* @deprecated in 6.0. Use {@link OrderedIterable#zip(Iterable)} instead.
*/
@Deprecated
public MutableBag> zip(Iterable that)
{
return this.zip(that, new HashBag>(this.size()));
}
/**
* @deprecated in 6.0. Use {@link OrderedIterable#zipWithIndex()} instead.
*/
@Deprecated
public MutableSet> zipWithIndex()
{
return this.zipWithIndex(new UnifiedSet>(this.size()));
}
public MutableMap withKeyValue(K key, V value)
{
this.put(key, value);
return this;
}
public MutableMap withAllKeyValues(Iterable extends Pair extends K, ? extends V>> keyValues)
{
for (Pair extends K, ? extends V> keyVal : keyValues)
{
this.put(keyVal.getOne(), keyVal.getTwo());
}
return this;
}
public MutableMap withAllKeyValueArguments(Pair extends K, ? extends V>... keyValues)
{
return this.withAllKeyValues(ArrayAdapter.adapt(keyValues));
}
public MutableMap withoutKey(K key)
{
this.removeKey(key);
return this;
}
public MutableMap withoutAllKeys(Iterable extends K> keys)
{
for (K key : keys)
{
this.removeKey(key);
}
return this;
}
/**
* Trait-style class that is used to capture commonalities between ValuesCollection class implementations in order to
* avoid code duplication.
*/
protected abstract static class ValuesCollectionCommon implements Collection
{
public boolean add(V v)
{
throw new UnsupportedOperationException("Cannot call add() on " + this.getClass().getSimpleName());
}
public boolean addAll(Collection extends V> collection)
{
throw new UnsupportedOperationException("Cannot call addAll() on " + this.getClass().getSimpleName());
}
}
public MutableBagMultimap groupBy(Function super V, ? extends VV> function)
{
return this.groupBy(function, HashBagMultimap.newMultimap());
}
public MutableBagMultimap groupByEach(Function super V, ? extends Iterable> function)
{
return this.groupByEach(function, HashBagMultimap.newMultimap());
}
}